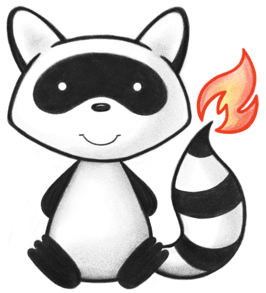
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CompositionStatus { 037 038 /** 039 * This is a preliminary composition or document (also known as initial or 040 * interim). The content may be incomplete or unverified. 041 */ 042 PRELIMINARY, 043 /** 044 * This version of the composition is complete and verified by an appropriate 045 * person and no further work is planned. Any subsequent updates would be on a 046 * new version of the composition. 047 */ 048 FINAL, 049 /** 050 * The composition content or the referenced resources have been modified 051 * (edited or added to) subsequent to being released as "final" and the 052 * composition is complete and verified by an authorized person. 053 */ 054 AMENDED, 055 /** 056 * The composition or document was originally created/issued in error, and this 057 * is an amendment that marks that the entire series should not be considered as 058 * valid. 059 */ 060 ENTEREDINERROR, 061 /** 062 * added to help the parsers 063 */ 064 NULL; 065 066 public static CompositionStatus fromCode(String codeString) throws FHIRException { 067 if (codeString == null || "".equals(codeString)) 068 return null; 069 if ("preliminary".equals(codeString)) 070 return PRELIMINARY; 071 if ("final".equals(codeString)) 072 return FINAL; 073 if ("amended".equals(codeString)) 074 return AMENDED; 075 if ("entered-in-error".equals(codeString)) 076 return ENTEREDINERROR; 077 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 078 } 079 080 public String toCode() { 081 switch (this) { 082 case PRELIMINARY: 083 return "preliminary"; 084 case FINAL: 085 return "final"; 086 case AMENDED: 087 return "amended"; 088 case ENTEREDINERROR: 089 return "entered-in-error"; 090 case NULL: 091 return null; 092 default: 093 return "?"; 094 } 095 } 096 097 public String getSystem() { 098 return "http://hl7.org/fhir/composition-status"; 099 } 100 101 public String getDefinition() { 102 switch (this) { 103 case PRELIMINARY: 104 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 105 case FINAL: 106 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 107 case AMENDED: 108 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 109 case ENTEREDINERROR: 110 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getDisplay() { 119 switch (this) { 120 case PRELIMINARY: 121 return "Preliminary"; 122 case FINAL: 123 return "Final"; 124 case AMENDED: 125 return "Amended"; 126 case ENTEREDINERROR: 127 return "Entered in Error"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135}