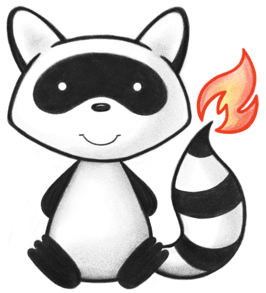
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ConceptMapEquivalence { 037 038 /** 039 * The concepts are related to each other, and have at least some overlap in 040 * meaning, but the exact relationship is not known. 041 */ 042 RELATEDTO, 043 /** 044 * The definitions of the concepts mean the same thing (including when 045 * structural implications of meaning are considered) (i.e. extensionally 046 * identical). 047 */ 048 EQUIVALENT, 049 /** 050 * The definitions of the concepts are exactly the same (i.e. only grammatical 051 * differences) and structural implications of meaning are identical or 052 * irrelevant (i.e. intentionally identical). 053 */ 054 EQUAL, 055 /** 056 * The target mapping is wider in meaning than the source concept. 057 */ 058 WIDER, 059 /** 060 * The target mapping subsumes the meaning of the source concept (e.g. the 061 * source is-a target). 062 */ 063 SUBSUMES, 064 /** 065 * The target mapping is narrower in meaning than the source concept. The sense 066 * in which the mapping is narrower SHALL be described in the comments in this 067 * case, and applications should be careful when attempting to use these 068 * mappings operationally. 069 */ 070 NARROWER, 071 /** 072 * The target mapping specializes the meaning of the source concept (e.g. the 073 * target is-a source). 074 */ 075 SPECIALIZES, 076 /** 077 * The target mapping overlaps with the source concept, but both source and 078 * target cover additional meaning, or the definitions are imprecise and it is 079 * uncertain whether they have the same boundaries to their meaning. The sense 080 * in which the mapping is inexact SHALL be described in the comments in this 081 * case, and applications should be careful when attempting to use these 082 * mappings operationally. 083 */ 084 INEXACT, 085 /** 086 * There is no match for this concept in the target code system. 087 */ 088 UNMATCHED, 089 /** 090 * This is an explicit assertion that there is no mapping between the source and 091 * target concept. 092 */ 093 DISJOINT, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static ConceptMapEquivalence fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("relatedto".equals(codeString)) 103 return RELATEDTO; 104 if ("equivalent".equals(codeString)) 105 return EQUIVALENT; 106 if ("equal".equals(codeString)) 107 return EQUAL; 108 if ("wider".equals(codeString)) 109 return WIDER; 110 if ("subsumes".equals(codeString)) 111 return SUBSUMES; 112 if ("narrower".equals(codeString)) 113 return NARROWER; 114 if ("specializes".equals(codeString)) 115 return SPECIALIZES; 116 if ("inexact".equals(codeString)) 117 return INEXACT; 118 if ("unmatched".equals(codeString)) 119 return UNMATCHED; 120 if ("disjoint".equals(codeString)) 121 return DISJOINT; 122 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 123 } 124 125 public String toCode() { 126 switch (this) { 127 case RELATEDTO: 128 return "relatedto"; 129 case EQUIVALENT: 130 return "equivalent"; 131 case EQUAL: 132 return "equal"; 133 case WIDER: 134 return "wider"; 135 case SUBSUMES: 136 return "subsumes"; 137 case NARROWER: 138 return "narrower"; 139 case SPECIALIZES: 140 return "specializes"; 141 case INEXACT: 142 return "inexact"; 143 case UNMATCHED: 144 return "unmatched"; 145 case DISJOINT: 146 return "disjoint"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 return "http://hl7.org/fhir/concept-map-equivalence"; 156 } 157 158 public String getDefinition() { 159 switch (this) { 160 case RELATEDTO: 161 return "The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known."; 162 case EQUIVALENT: 163 return "The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical)."; 164 case EQUAL: 165 return "The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical)."; 166 case WIDER: 167 return "The target mapping is wider in meaning than the source concept."; 168 case SUBSUMES: 169 return "The target mapping subsumes the meaning of the source concept (e.g. the source is-a target)."; 170 case NARROWER: 171 return "The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 172 case SPECIALIZES: 173 return "The target mapping specializes the meaning of the source concept (e.g. the target is-a source)."; 174 case INEXACT: 175 return "The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is inexact SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 176 case UNMATCHED: 177 return "There is no match for this concept in the target code system."; 178 case DISJOINT: 179 return "This is an explicit assertion that there is no mapping between the source and target concept."; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 187 public String getDisplay() { 188 switch (this) { 189 case RELATEDTO: 190 return "Related To"; 191 case EQUIVALENT: 192 return "Equivalent"; 193 case EQUAL: 194 return "Equal"; 195 case WIDER: 196 return "Wider"; 197 case SUBSUMES: 198 return "Subsumes"; 199 case NARROWER: 200 return "Narrower"; 201 case SPECIALIZES: 202 return "Specializes"; 203 case INEXACT: 204 return "Inexact"; 205 case UNMATCHED: 206 return "Unmatched"; 207 case DISJOINT: 208 return "Disjoint"; 209 case NULL: 210 return null; 211 default: 212 return "?"; 213 } 214 } 215 216}