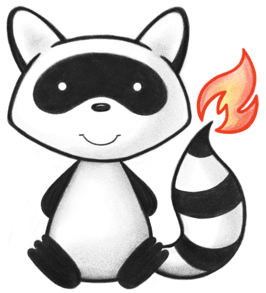
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ConceptPropertyType { 037 038 /** 039 * The property value is a code that identifies a concept defined in the code 040 * system. 041 */ 042 CODE, 043 /** 044 * The property value is a code defined in an external code system. This may be 045 * used for translations, but is not the intent. 046 */ 047 CODING, 048 /** 049 * The property value is a string. 050 */ 051 STRING, 052 /** 053 * The property value is a string (often used to assign ranking values to 054 * concepts for supporting score assessments). 055 */ 056 INTEGER, 057 /** 058 * The property value is a boolean true | false. 059 */ 060 BOOLEAN, 061 /** 062 * The property is a date or a date + time. 063 */ 064 DATETIME, 065 /** 066 * The property value is a decimal number. 067 */ 068 DECIMAL, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static ConceptPropertyType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("code".equals(codeString)) 078 return CODE; 079 if ("Coding".equals(codeString)) 080 return CODING; 081 if ("string".equals(codeString)) 082 return STRING; 083 if ("integer".equals(codeString)) 084 return INTEGER; 085 if ("boolean".equals(codeString)) 086 return BOOLEAN; 087 if ("dateTime".equals(codeString)) 088 return DATETIME; 089 if ("decimal".equals(codeString)) 090 return DECIMAL; 091 throw new FHIRException("Unknown ConceptPropertyType code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case CODE: 097 return "code"; 098 case CODING: 099 return "Coding"; 100 case STRING: 101 return "string"; 102 case INTEGER: 103 return "integer"; 104 case BOOLEAN: 105 return "boolean"; 106 case DATETIME: 107 return "dateTime"; 108 case DECIMAL: 109 return "decimal"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getSystem() { 118 return "http://hl7.org/fhir/concept-property-type"; 119 } 120 121 public String getDefinition() { 122 switch (this) { 123 case CODE: 124 return "The property value is a code that identifies a concept defined in the code system."; 125 case CODING: 126 return "The property value is a code defined in an external code system. This may be used for translations, but is not the intent."; 127 case STRING: 128 return "The property value is a string."; 129 case INTEGER: 130 return "The property value is a string (often used to assign ranking values to concepts for supporting score assessments)."; 131 case BOOLEAN: 132 return "The property value is a boolean true | false."; 133 case DATETIME: 134 return "The property is a date or a date + time."; 135 case DECIMAL: 136 return "The property value is a decimal number."; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getDisplay() { 145 switch (this) { 146 case CODE: 147 return "code (internal reference)"; 148 case CODING: 149 return "Coding (external reference)"; 150 case STRING: 151 return "string"; 152 case INTEGER: 153 return "integer"; 154 case BOOLEAN: 155 return "boolean"; 156 case DATETIME: 157 return "dateTime"; 158 case DECIMAL: 159 return "decimal"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167}