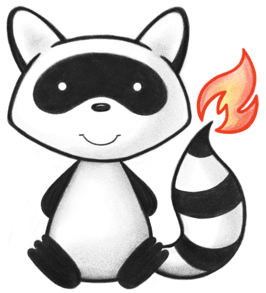
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ContactPointSystem { 037 038 /** 039 * The value is a telephone number used for voice calls. Use of full 040 * international numbers starting with + is recommended to enable automatic 041 * dialing support but not required. 042 */ 043 PHONE, 044 /** 045 * The value is a fax machine. Use of full international numbers starting with + 046 * is recommended to enable automatic dialing support but not required. 047 */ 048 FAX, 049 /** 050 * The value is an email address. 051 */ 052 EMAIL, 053 /** 054 * The value is a pager number. These may be local pager numbers that are only 055 * usable on a particular pager system. 056 */ 057 PAGER, 058 /** 059 * A contact that is not a phone, fax, pager or email address and is expressed 060 * as a URL. This is intended for various institutional or personal contacts 061 * including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for 062 * email addresses. 063 */ 064 URL, 065 /** 066 * A contact that can be used for sending an sms message (e.g. mobile phones, 067 * some landlines). 068 */ 069 SMS, 070 /** 071 * A contact that is not a phone, fax, page or email address and is not 072 * expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for 073 * contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) 074 * Extensions may be used to distinguish "other" contact types. 075 */ 076 OTHER, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static ContactPointSystem fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("phone".equals(codeString)) 086 return PHONE; 087 if ("fax".equals(codeString)) 088 return FAX; 089 if ("email".equals(codeString)) 090 return EMAIL; 091 if ("pager".equals(codeString)) 092 return PAGER; 093 if ("url".equals(codeString)) 094 return URL; 095 if ("sms".equals(codeString)) 096 return SMS; 097 if ("other".equals(codeString)) 098 return OTHER; 099 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PHONE: 105 return "phone"; 106 case FAX: 107 return "fax"; 108 case EMAIL: 109 return "email"; 110 case PAGER: 111 return "pager"; 112 case URL: 113 return "url"; 114 case SMS: 115 return "sms"; 116 case OTHER: 117 return "other"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getSystem() { 126 return "http://hl7.org/fhir/contact-point-system"; 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case PHONE: 132 return "The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 133 case FAX: 134 return "The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 135 case EMAIL: 136 return "The value is an email address."; 137 case PAGER: 138 return "The value is a pager number. These may be local pager numbers that are only usable on a particular pager system."; 139 case URL: 140 return "A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various institutional or personal contacts including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses."; 141 case SMS: 142 return "A contact that can be used for sending an sms message (e.g. mobile phones, some landlines)."; 143 case OTHER: 144 return "A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish \"other\" contact types."; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 152 public String getDisplay() { 153 switch (this) { 154 case PHONE: 155 return "Phone"; 156 case FAX: 157 return "Fax"; 158 case EMAIL: 159 return "Email"; 160 case PAGER: 161 return "Pager"; 162 case URL: 163 return "URL"; 164 case SMS: 165 return "SMS"; 166 case OTHER: 167 return "Other"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 175}