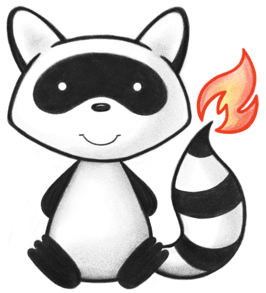
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CoverageClass { 037 038 /** 039 * An employee group 040 */ 041 GROUP, 042 /** 043 * A sub-group of an employee group 044 */ 045 SUBGROUP, 046 /** 047 * A specific suite of benefits. 048 */ 049 PLAN, 050 /** 051 * A subset of a specific suite of benefits. 052 */ 053 SUBPLAN, 054 /** 055 * A class of benefits. 056 */ 057 CLASS, 058 /** 059 * A subset of a class of benefits. 060 */ 061 SUBCLASS, 062 /** 063 * A sequence number associated with a short-term continuance of the coverage. 064 */ 065 SEQUENCE, 066 /** 067 * Pharmacy benefit manager's Business Identification Number. 068 */ 069 RXBIN, 070 /** 071 * A Pharmacy Benefit Manager specified Processor Control Number. 072 */ 073 RXPCN, 074 /** 075 * A Pharmacy Benefit Manager specified Member ID. 076 */ 077 RXID, 078 /** 079 * A Pharmacy Benefit Manager specified Group number. 080 */ 081 RXGROUP, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static CoverageClass fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("group".equals(codeString)) 091 return GROUP; 092 if ("subgroup".equals(codeString)) 093 return SUBGROUP; 094 if ("plan".equals(codeString)) 095 return PLAN; 096 if ("subplan".equals(codeString)) 097 return SUBPLAN; 098 if ("class".equals(codeString)) 099 return CLASS; 100 if ("subclass".equals(codeString)) 101 return SUBCLASS; 102 if ("sequence".equals(codeString)) 103 return SEQUENCE; 104 if ("rxbin".equals(codeString)) 105 return RXBIN; 106 if ("rxpcn".equals(codeString)) 107 return RXPCN; 108 if ("rxid".equals(codeString)) 109 return RXID; 110 if ("rxgroup".equals(codeString)) 111 return RXGROUP; 112 throw new FHIRException("Unknown CoverageClass code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case GROUP: 118 return "group"; 119 case SUBGROUP: 120 return "subgroup"; 121 case PLAN: 122 return "plan"; 123 case SUBPLAN: 124 return "subplan"; 125 case CLASS: 126 return "class"; 127 case SUBCLASS: 128 return "subclass"; 129 case SEQUENCE: 130 return "sequence"; 131 case RXBIN: 132 return "rxbin"; 133 case RXPCN: 134 return "rxpcn"; 135 case RXID: 136 return "rxid"; 137 case RXGROUP: 138 return "rxgroup"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getSystem() { 147 return "http://terminology.hl7.org/CodeSystem/coverage-class"; 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case GROUP: 153 return "An employee group"; 154 case SUBGROUP: 155 return "A sub-group of an employee group"; 156 case PLAN: 157 return "A specific suite of benefits."; 158 case SUBPLAN: 159 return "A subset of a specific suite of benefits."; 160 case CLASS: 161 return "A class of benefits."; 162 case SUBCLASS: 163 return "A subset of a class of benefits."; 164 case SEQUENCE: 165 return "A sequence number associated with a short-term continuance of the coverage."; 166 case RXBIN: 167 return "Pharmacy benefit manager's Business Identification Number."; 168 case RXPCN: 169 return "A Pharmacy Benefit Manager specified Processor Control Number."; 170 case RXID: 171 return "A Pharmacy Benefit Manager specified Member ID."; 172 case RXGROUP: 173 return "A Pharmacy Benefit Manager specified Group number."; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDisplay() { 182 switch (this) { 183 case GROUP: 184 return "Group"; 185 case SUBGROUP: 186 return "SubGroup"; 187 case PLAN: 188 return "Plan"; 189 case SUBPLAN: 190 return "SubPlan"; 191 case CLASS: 192 return "Class"; 193 case SUBCLASS: 194 return "SubClass"; 195 case SEQUENCE: 196 return "Sequence"; 197 case RXBIN: 198 return "RX BIN"; 199 case RXPCN: 200 return "RX PCN"; 201 case RXID: 202 return "RX Id"; 203 case RXGROUP: 204 return "RX Group"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212}