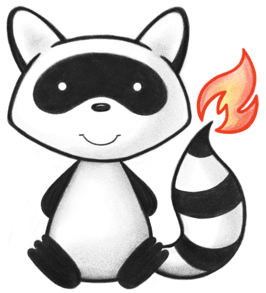
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CoverageCopayType { 037 038 /** 039 * An office visit for a general practitioner of a discipline. 040 */ 041 GPVISIT, 042 /** 043 * An office visit for a specialist practitioner of a discipline 044 */ 045 SPVISIT, 046 /** 047 * An episode in an emergency department. 048 */ 049 EMERGENCY, 050 /** 051 * An episode of an Inpatient hospital stay. 052 */ 053 INPTHOSP, 054 /** 055 * A visit held where the patient is remote relative to the practitioner, e.g. 056 * by phone, computer or video conference. 057 */ 058 TELEVISIT, 059 /** 060 * A visit to an urgent care facility - typically a community care clinic. 061 */ 062 URGENTCARE, 063 /** 064 * A standard percentage applied to all classes or service or product not 065 * otherwise specified. 066 */ 067 COPAYPCT, 068 /** 069 * A standard fixed currency amount applied to all classes or service or product 070 * not otherwise specified. 071 */ 072 COPAY, 073 /** 074 * The accumulated amount of patient payment before the coverage begins to pay 075 * for services. 076 */ 077 DEDUCTIBLE, 078 /** 079 * The maximum amout of payment for services which a patient, or family, is 080 * expected to incur - typically annually. 081 */ 082 MAXOUTOFPOCKET, 083 /** 084 * added to help the parsers 085 */ 086 NULL; 087 088 public static CoverageCopayType fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("gpvisit".equals(codeString)) 092 return GPVISIT; 093 if ("spvisit".equals(codeString)) 094 return SPVISIT; 095 if ("emergency".equals(codeString)) 096 return EMERGENCY; 097 if ("inpthosp".equals(codeString)) 098 return INPTHOSP; 099 if ("televisit".equals(codeString)) 100 return TELEVISIT; 101 if ("urgentcare".equals(codeString)) 102 return URGENTCARE; 103 if ("copaypct".equals(codeString)) 104 return COPAYPCT; 105 if ("copay".equals(codeString)) 106 return COPAY; 107 if ("deductible".equals(codeString)) 108 return DEDUCTIBLE; 109 if ("maxoutofpocket".equals(codeString)) 110 return MAXOUTOFPOCKET; 111 throw new FHIRException("Unknown CoverageCopayType code '" + codeString + "'"); 112 } 113 114 public String toCode() { 115 switch (this) { 116 case GPVISIT: 117 return "gpvisit"; 118 case SPVISIT: 119 return "spvisit"; 120 case EMERGENCY: 121 return "emergency"; 122 case INPTHOSP: 123 return "inpthosp"; 124 case TELEVISIT: 125 return "televisit"; 126 case URGENTCARE: 127 return "urgentcare"; 128 case COPAYPCT: 129 return "copaypct"; 130 case COPAY: 131 return "copay"; 132 case DEDUCTIBLE: 133 return "deductible"; 134 case MAXOUTOFPOCKET: 135 return "maxoutofpocket"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getSystem() { 144 return "http://terminology.hl7.org/CodeSystem/coverage-copay-type"; 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case GPVISIT: 150 return "An office visit for a general practitioner of a discipline."; 151 case SPVISIT: 152 return "An office visit for a specialist practitioner of a discipline"; 153 case EMERGENCY: 154 return "An episode in an emergency department."; 155 case INPTHOSP: 156 return "An episode of an Inpatient hospital stay."; 157 case TELEVISIT: 158 return "A visit held where the patient is remote relative to the practitioner, e.g. by phone, computer or video conference."; 159 case URGENTCARE: 160 return "A visit to an urgent care facility - typically a community care clinic."; 161 case COPAYPCT: 162 return "A standard percentage applied to all classes or service or product not otherwise specified."; 163 case COPAY: 164 return "A standard fixed currency amount applied to all classes or service or product not otherwise specified."; 165 case DEDUCTIBLE: 166 return "The accumulated amount of patient payment before the coverage begins to pay for services."; 167 case MAXOUTOFPOCKET: 168 return "The maximum amout of payment for services which a patient, or family, is expected to incur - typically annually."; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176 public String getDisplay() { 177 switch (this) { 178 case GPVISIT: 179 return "GP Office Visit"; 180 case SPVISIT: 181 return "Specialist Office Visit"; 182 case EMERGENCY: 183 return "Emergency"; 184 case INPTHOSP: 185 return "Inpatient Hospital"; 186 case TELEVISIT: 187 return "Tele-visit"; 188 case URGENTCARE: 189 return "Urgent Care"; 190 case COPAYPCT: 191 return "Copay Percentage"; 192 case COPAY: 193 return "Copay Amount"; 194 case DEDUCTIBLE: 195 return "Deductible"; 196 case MAXOUTOFPOCKET: 197 return "Maximum out of pocket"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 205}