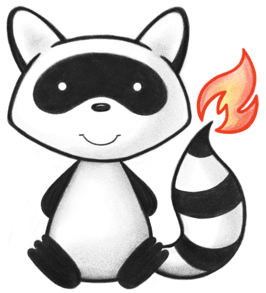
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum DefinitionResourceTypes { 037 038 /** 039 * This resource allows for the definition of some activity to be performed, 040 * independent of a particular patient, practitioner, or other performance 041 * context. 042 */ 043 ACTIVITYDEFINITION, 044 /** 045 * The EventDefinition resource provides a reusable description of when a 046 * particular event can occur. 047 */ 048 EVENTDEFINITION, 049 /** 050 * The Measure resource provides the definition of a quality measure. 051 */ 052 MEASURE, 053 /** 054 * A formal computable definition of an operation (on the RESTful interface) or 055 * a named query (using the search interaction). 056 */ 057 OPERATIONDEFINITION, 058 /** 059 * This resource allows for the definition of various types of plans as a 060 * sharable, consumable, and executable artifact. The resource is general enough 061 * to support the description of a broad range of clinical artifacts such as 062 * clinical decision support rules, order sets and protocols. 063 */ 064 PLANDEFINITION, 065 /** 066 * A structured set of questions intended to guide the collection of answers 067 * from end-users. Questionnaires provide detailed control over order, 068 * presentation, phraseology and grouping to allow coherent, consistent data 069 * collection. 070 */ 071 QUESTIONNAIRE, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static DefinitionResourceTypes fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("ActivityDefinition".equals(codeString)) 081 return ACTIVITYDEFINITION; 082 if ("EventDefinition".equals(codeString)) 083 return EVENTDEFINITION; 084 if ("Measure".equals(codeString)) 085 return MEASURE; 086 if ("OperationDefinition".equals(codeString)) 087 return OPERATIONDEFINITION; 088 if ("PlanDefinition".equals(codeString)) 089 return PLANDEFINITION; 090 if ("Questionnaire".equals(codeString)) 091 return QUESTIONNAIRE; 092 throw new FHIRException("Unknown DefinitionResourceTypes code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case ACTIVITYDEFINITION: 098 return "ActivityDefinition"; 099 case EVENTDEFINITION: 100 return "EventDefinition"; 101 case MEASURE: 102 return "Measure"; 103 case OPERATIONDEFINITION: 104 return "OperationDefinition"; 105 case PLANDEFINITION: 106 return "PlanDefinition"; 107 case QUESTIONNAIRE: 108 return "Questionnaire"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getSystem() { 117 return "http://hl7.org/fhir/definition-resource-types"; 118 } 119 120 public String getDefinition() { 121 switch (this) { 122 case ACTIVITYDEFINITION: 123 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 124 case EVENTDEFINITION: 125 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 126 case MEASURE: 127 return "The Measure resource provides the definition of a quality measure."; 128 case OPERATIONDEFINITION: 129 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 130 case PLANDEFINITION: 131 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 132 case QUESTIONNAIRE: 133 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case ACTIVITYDEFINITION: 144 return "ActivityDefinition"; 145 case EVENTDEFINITION: 146 return "EventDefinition"; 147 case MEASURE: 148 return "Measure"; 149 case OPERATIONDEFINITION: 150 return "OperationDefinition"; 151 case PLANDEFINITION: 152 return "PlanDefinition"; 153 case QUESTIONNAIRE: 154 return "Questionnaire"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162}