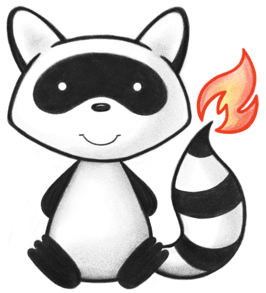
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum DicomAuditLifecycle { 037 038 /** 039 * null 040 */ 041 _1, 042 /** 043 * null 044 */ 045 _2, 046 /** 047 * null 048 */ 049 _3, 050 /** 051 * null 052 */ 053 _4, 054 /** 055 * null 056 */ 057 _5, 058 /** 059 * null 060 */ 061 _6, 062 /** 063 * null 064 */ 065 _7, 066 /** 067 * null 068 */ 069 _8, 070 /** 071 * null 072 */ 073 _9, 074 /** 075 * null 076 */ 077 _10, 078 /** 079 * null 080 */ 081 _11, 082 /** 083 * null 084 */ 085 _12, 086 /** 087 * null 088 */ 089 _13, 090 /** 091 * null 092 */ 093 _14, 094 /** 095 * null 096 */ 097 _15, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 103 public static DicomAuditLifecycle fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("1".equals(codeString)) 107 return _1; 108 if ("2".equals(codeString)) 109 return _2; 110 if ("3".equals(codeString)) 111 return _3; 112 if ("4".equals(codeString)) 113 return _4; 114 if ("5".equals(codeString)) 115 return _5; 116 if ("6".equals(codeString)) 117 return _6; 118 if ("7".equals(codeString)) 119 return _7; 120 if ("8".equals(codeString)) 121 return _8; 122 if ("9".equals(codeString)) 123 return _9; 124 if ("10".equals(codeString)) 125 return _10; 126 if ("11".equals(codeString)) 127 return _11; 128 if ("12".equals(codeString)) 129 return _12; 130 if ("13".equals(codeString)) 131 return _13; 132 if ("14".equals(codeString)) 133 return _14; 134 if ("15".equals(codeString)) 135 return _15; 136 throw new FHIRException("Unknown DicomAuditLifecycle code '" + codeString + "'"); 137 } 138 139 public String toCode() { 140 switch (this) { 141 case _1: 142 return "1"; 143 case _2: 144 return "2"; 145 case _3: 146 return "3"; 147 case _4: 148 return "4"; 149 case _5: 150 return "5"; 151 case _6: 152 return "6"; 153 case _7: 154 return "7"; 155 case _8: 156 return "8"; 157 case _9: 158 return "9"; 159 case _10: 160 return "10"; 161 case _11: 162 return "11"; 163 case _12: 164 return "12"; 165 case _13: 166 return "13"; 167 case _14: 168 return "14"; 169 case _15: 170 return "15"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getSystem() { 179 return "http://terminology.hl7.org/CodeSystem/dicom-audit-lifecycle"; 180 } 181 182 public String getDefinition() { 183 switch (this) { 184 case _1: 185 return ""; 186 case _2: 187 return ""; 188 case _3: 189 return ""; 190 case _4: 191 return ""; 192 case _5: 193 return ""; 194 case _6: 195 return ""; 196 case _7: 197 return ""; 198 case _8: 199 return ""; 200 case _9: 201 return ""; 202 case _10: 203 return ""; 204 case _11: 205 return ""; 206 case _12: 207 return ""; 208 case _13: 209 return ""; 210 case _14: 211 return ""; 212 case _15: 213 return ""; 214 case NULL: 215 return null; 216 default: 217 return "?"; 218 } 219 } 220 221 public String getDisplay() { 222 switch (this) { 223 case _1: 224 return "Origination / Creation"; 225 case _2: 226 return "Import / Copy"; 227 case _3: 228 return "Amendment"; 229 case _4: 230 return "Verification"; 231 case _5: 232 return "Translation"; 233 case _6: 234 return "Access / Use"; 235 case _7: 236 return "De-identification"; 237 case _8: 238 return "Aggregation / summarization / derivation"; 239 case _9: 240 return "Report"; 241 case _10: 242 return "Export"; 243 case _11: 244 return "Disclosure"; 245 case _12: 246 return "Receipt of disclosure"; 247 case _13: 248 return "Archiving"; 249 case _14: 250 return "Logical deletion"; 251 case _15: 252 return "Permanent erasure / Physical destruction"; 253 case NULL: 254 return null; 255 default: 256 return "?"; 257 } 258 } 259 260}