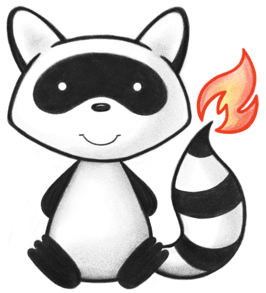
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum EncounterAdmitSource { 037 038 /** 039 * The Patient has been transferred from another hospital for this encounter. 040 */ 041 HOSPTRANS, 042 /** 043 * The patient has been transferred from the emergency department within the 044 * hospital. This is typically used in the transition to an inpatient encounter 045 */ 046 EMD, 047 /** 048 * The patient has been transferred from an outpatient department within the 049 * hospital. 050 */ 051 OUTP, 052 /** 053 * The patient is a newborn and the encounter will track the baby related 054 * activities (as opposed to the Mothers encounter - that may be associated 055 * using the newborn encounters partof property) 056 */ 057 BORN, 058 /** 059 * The patient has been admitted due to a referred from a General Practitioner. 060 */ 061 GP, 062 /** 063 * The patient has been admitted due to a referred from a Specialist (as opposed 064 * to a General Practitioner). 065 */ 066 MP, 067 /** 068 * The patient has been transferred from a nursing home. 069 */ 070 NURSING, 071 /** 072 * The patient has been transferred from a psychiatric facility. 073 */ 074 PSYCH, 075 /** 076 * The patient has been transferred from a rehabilitation facility or clinic. 077 */ 078 REHAB, 079 /** 080 * The patient has been admitted from a source otherwise not specified here. 081 */ 082 OTHER, 083 /** 084 * added to help the parsers 085 */ 086 NULL; 087 088 public static EncounterAdmitSource fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("hosp-trans".equals(codeString)) 092 return HOSPTRANS; 093 if ("emd".equals(codeString)) 094 return EMD; 095 if ("outp".equals(codeString)) 096 return OUTP; 097 if ("born".equals(codeString)) 098 return BORN; 099 if ("gp".equals(codeString)) 100 return GP; 101 if ("mp".equals(codeString)) 102 return MP; 103 if ("nursing".equals(codeString)) 104 return NURSING; 105 if ("psych".equals(codeString)) 106 return PSYCH; 107 if ("rehab".equals(codeString)) 108 return REHAB; 109 if ("other".equals(codeString)) 110 return OTHER; 111 throw new FHIRException("Unknown EncounterAdmitSource code '" + codeString + "'"); 112 } 113 114 public String toCode() { 115 switch (this) { 116 case HOSPTRANS: 117 return "hosp-trans"; 118 case EMD: 119 return "emd"; 120 case OUTP: 121 return "outp"; 122 case BORN: 123 return "born"; 124 case GP: 125 return "gp"; 126 case MP: 127 return "mp"; 128 case NURSING: 129 return "nursing"; 130 case PSYCH: 131 return "psych"; 132 case REHAB: 133 return "rehab"; 134 case OTHER: 135 return "other"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getSystem() { 144 return "http://terminology.hl7.org/CodeSystem/admit-source"; 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case HOSPTRANS: 150 return "The Patient has been transferred from another hospital for this encounter."; 151 case EMD: 152 return "The patient has been transferred from the emergency department within the hospital. This is typically used in the transition to an inpatient encounter"; 153 case OUTP: 154 return "The patient has been transferred from an outpatient department within the hospital."; 155 case BORN: 156 return "The patient is a newborn and the encounter will track the baby related activities (as opposed to the Mothers encounter - that may be associated using the newborn encounters partof property)"; 157 case GP: 158 return "The patient has been admitted due to a referred from a General Practitioner."; 159 case MP: 160 return "The patient has been admitted due to a referred from a Specialist (as opposed to a General Practitioner)."; 161 case NURSING: 162 return "The patient has been transferred from a nursing home."; 163 case PSYCH: 164 return "The patient has been transferred from a psychiatric facility."; 165 case REHAB: 166 return "The patient has been transferred from a rehabilitation facility or clinic."; 167 case OTHER: 168 return "The patient has been admitted from a source otherwise not specified here."; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176 public String getDisplay() { 177 switch (this) { 178 case HOSPTRANS: 179 return "Transferred from other hospital"; 180 case EMD: 181 return "From accident/emergency department"; 182 case OUTP: 183 return "From outpatient department"; 184 case BORN: 185 return "Born in hospital"; 186 case GP: 187 return "General Practitioner referral"; 188 case MP: 189 return "Medical Practitioner/physician referral"; 190 case NURSING: 191 return "From nursing home"; 192 case PSYCH: 193 return "From psychiatric hospital"; 194 case REHAB: 195 return "From rehabilitation facility"; 196 case OTHER: 197 return "Other"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 205}