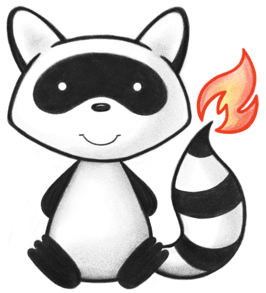
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum EncounterDischargeDisposition { 037 038 /** 039 * The patient was dicharged and has indicated that they are going to return 040 * home afterwards. 041 */ 042 HOME, 043 /** 044 * The patient was discharged and has indicated that they are going to return 045 * home afterwards, but not the patient's home - e.g. a family member's home. 046 */ 047 ALTHOME, 048 /** 049 * The patient was transferred to another healthcare facility. 050 */ 051 OTHERHCF, 052 /** 053 * The patient has been discharged into palliative care. 054 */ 055 HOSP, 056 /** 057 * The patient has been discharged into long-term care where is likely to be 058 * monitored through an ongoing episode-of-care. 059 */ 060 LONG, 061 /** 062 * The patient self discharged against medical advice. 063 */ 064 AADVICE, 065 /** 066 * The patient has deceased during this encounter. 067 */ 068 EXP, 069 /** 070 * The patient has been transferred to a psychiatric facility. 071 */ 072 PSY, 073 /** 074 * The patient was discharged and is to receive post acute care rehabilitation 075 * services. 076 */ 077 REHAB, 078 /** 079 * The patient has been discharged to a skilled nursing facility for the patient 080 * to receive additional care. 081 */ 082 SNF, 083 /** 084 * The discharge disposition has not otherwise defined. 085 */ 086 OTH, 087 /** 088 * added to help the parsers 089 */ 090 NULL; 091 092 public static EncounterDischargeDisposition fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("home".equals(codeString)) 096 return HOME; 097 if ("alt-home".equals(codeString)) 098 return ALTHOME; 099 if ("other-hcf".equals(codeString)) 100 return OTHERHCF; 101 if ("hosp".equals(codeString)) 102 return HOSP; 103 if ("long".equals(codeString)) 104 return LONG; 105 if ("aadvice".equals(codeString)) 106 return AADVICE; 107 if ("exp".equals(codeString)) 108 return EXP; 109 if ("psy".equals(codeString)) 110 return PSY; 111 if ("rehab".equals(codeString)) 112 return REHAB; 113 if ("snf".equals(codeString)) 114 return SNF; 115 if ("oth".equals(codeString)) 116 return OTH; 117 throw new FHIRException("Unknown EncounterDischargeDisposition code '" + codeString + "'"); 118 } 119 120 public String toCode() { 121 switch (this) { 122 case HOME: 123 return "home"; 124 case ALTHOME: 125 return "alt-home"; 126 case OTHERHCF: 127 return "other-hcf"; 128 case HOSP: 129 return "hosp"; 130 case LONG: 131 return "long"; 132 case AADVICE: 133 return "aadvice"; 134 case EXP: 135 return "exp"; 136 case PSY: 137 return "psy"; 138 case REHAB: 139 return "rehab"; 140 case SNF: 141 return "snf"; 142 case OTH: 143 return "oth"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getSystem() { 152 return "http://terminology.hl7.org/CodeSystem/discharge-disposition"; 153 } 154 155 public String getDefinition() { 156 switch (this) { 157 case HOME: 158 return "The patient was dicharged and has indicated that they are going to return home afterwards."; 159 case ALTHOME: 160 return "The patient was discharged and has indicated that they are going to return home afterwards, but not the patient's home - e.g. a family member's home."; 161 case OTHERHCF: 162 return "The patient was transferred to another healthcare facility."; 163 case HOSP: 164 return "The patient has been discharged into palliative care."; 165 case LONG: 166 return "The patient has been discharged into long-term care where is likely to be monitored through an ongoing episode-of-care."; 167 case AADVICE: 168 return "The patient self discharged against medical advice."; 169 case EXP: 170 return "The patient has deceased during this encounter."; 171 case PSY: 172 return "The patient has been transferred to a psychiatric facility."; 173 case REHAB: 174 return "The patient was discharged and is to receive post acute care rehabilitation services."; 175 case SNF: 176 return "The patient has been discharged to a skilled nursing facility for the patient to receive additional care."; 177 case OTH: 178 return "The discharge disposition has not otherwise defined."; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 186 public String getDisplay() { 187 switch (this) { 188 case HOME: 189 return "Home"; 190 case ALTHOME: 191 return "Alternative home"; 192 case OTHERHCF: 193 return "Other healthcare facility"; 194 case HOSP: 195 return "Hospice"; 196 case LONG: 197 return "Long-term care"; 198 case AADVICE: 199 return "Left against advice"; 200 case EXP: 201 return "Expired"; 202 case PSY: 203 return "Psychiatric hospital"; 204 case REHAB: 205 return "Rehabilitation"; 206 case SNF: 207 return "Skilled nursing facility"; 208 case OTH: 209 return "Other"; 210 case NULL: 211 return null; 212 default: 213 return "?"; 214 } 215 } 216 217}