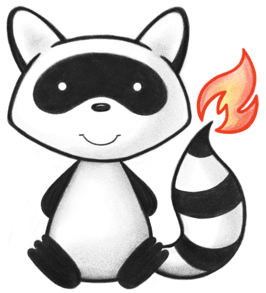
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum EpisodeOfCareStatus { 037 038 /** 039 * This episode of care is planned to start at the date specified in the 040 * period.start. During this status, an organization may perform assessments to 041 * determine if the patient is eligible to receive services, or be organizing to 042 * make resources available to provide care services. 043 */ 044 PLANNED, 045 /** 046 * This episode has been placed on a waitlist, pending the episode being made 047 * active (or cancelled). 048 */ 049 WAITLIST, 050 /** 051 * This episode of care is current. 052 */ 053 ACTIVE, 054 /** 055 * This episode of care is on hold; the organization has limited responsibility 056 * for the patient (such as while on respite). 057 */ 058 ONHOLD, 059 /** 060 * This episode of care is finished and the organization is not expecting to be 061 * providing further care to the patient. Can also be known as "closed", 062 * "completed" or other similar terms. 063 */ 064 FINISHED, 065 /** 066 * The episode of care was cancelled, or withdrawn from service, often selected 067 * during the planned stage as the patient may have gone elsewhere, or the 068 * circumstances have changed and the organization is unable to provide the 069 * care. It indicates that services terminated outside the planned/expected 070 * workflow. 071 */ 072 CANCELLED, 073 /** 074 * This instance should not have been part of this patient's medical record. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("planned".equals(codeString)) 086 return PLANNED; 087 if ("waitlist".equals(codeString)) 088 return WAITLIST; 089 if ("active".equals(codeString)) 090 return ACTIVE; 091 if ("onhold".equals(codeString)) 092 return ONHOLD; 093 if ("finished".equals(codeString)) 094 return FINISHED; 095 if ("cancelled".equals(codeString)) 096 return CANCELLED; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PLANNED: 105 return "planned"; 106 case WAITLIST: 107 return "waitlist"; 108 case ACTIVE: 109 return "active"; 110 case ONHOLD: 111 return "onhold"; 112 case FINISHED: 113 return "finished"; 114 case CANCELLED: 115 return "cancelled"; 116 case ENTEREDINERROR: 117 return "entered-in-error"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getSystem() { 126 return "http://hl7.org/fhir/episode-of-care-status"; 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case PLANNED: 132 return "This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services."; 133 case WAITLIST: 134 return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 135 case ACTIVE: 136 return "This episode of care is current."; 137 case ONHOLD: 138 return "This episode of care is on hold; the organization has limited responsibility for the patient (such as while on respite)."; 139 case FINISHED: 140 return "This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 141 case CANCELLED: 142 return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 143 case ENTEREDINERROR: 144 return "This instance should not have been part of this patient's medical record."; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 152 public String getDisplay() { 153 switch (this) { 154 case PLANNED: 155 return "Planned"; 156 case WAITLIST: 157 return "Waitlist"; 158 case ACTIVE: 159 return "Active"; 160 case ONHOLD: 161 return "On Hold"; 162 case FINISHED: 163 return "Finished"; 164 case CANCELLED: 165 return "Cancelled"; 166 case ENTEREDINERROR: 167 return "Entered in Error"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 175}