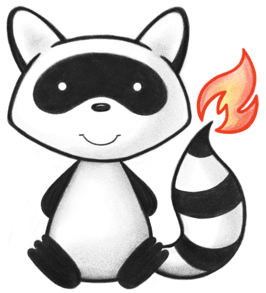
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum EventStatus { 037 038 /** 039 * The core event has not started yet, but some staging activities have begun 040 * (e.g. surgical suite preparation). Preparation stages may be tracked for 041 * billing purposes. 042 */ 043 PREPARATION, 044 /** 045 * The event is currently occurring. 046 */ 047 INPROGRESS, 048 /** 049 * The event was terminated prior to any activity beyond preparation. I.e. The 050 * 'main' activity has not yet begun. The boundary between preparatory and the 051 * 'main' activity is context-specific. 052 */ 053 NOTDONE, 054 /** 055 * The event has been temporarily stopped but is expected to resume in the 056 * future. 057 */ 058 ONHOLD, 059 /** 060 * The event was terminated prior to the full completion of the intended 061 * activity but after at least some of the 'main' activity (beyond preparation) 062 * has occurred. 063 */ 064 STOPPED, 065 /** 066 * The event has now concluded. 067 */ 068 COMPLETED, 069 /** 070 * This electronic record should never have existed, though it is possible that 071 * real-world decisions were based on it. (If real-world activity has occurred, 072 * the status should be "cancelled" rather than "entered-in-error".). 073 */ 074 ENTEREDINERROR, 075 /** 076 * The authoring/source system does not know which of the status values 077 * currently applies for this event. Note: This concept is not to be used for 078 * "other" - one of the listed statuses is presumed to apply, but the 079 * authoring/source system does not know which. 080 */ 081 UNKNOWN, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static EventStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("preparation".equals(codeString)) 091 return PREPARATION; 092 if ("in-progress".equals(codeString)) 093 return INPROGRESS; 094 if ("not-done".equals(codeString)) 095 return NOTDONE; 096 if ("on-hold".equals(codeString)) 097 return ONHOLD; 098 if ("stopped".equals(codeString)) 099 return STOPPED; 100 if ("completed".equals(codeString)) 101 return COMPLETED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 throw new FHIRException("Unknown EventStatus code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case PREPARATION: 112 return "preparation"; 113 case INPROGRESS: 114 return "in-progress"; 115 case NOTDONE: 116 return "not-done"; 117 case ONHOLD: 118 return "on-hold"; 119 case STOPPED: 120 return "stopped"; 121 case COMPLETED: 122 return "completed"; 123 case ENTEREDINERROR: 124 return "entered-in-error"; 125 case UNKNOWN: 126 return "unknown"; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getSystem() { 135 return "http://hl7.org/fhir/event-status"; 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case PREPARATION: 141 return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 142 case INPROGRESS: 143 return "The event is currently occurring."; 144 case NOTDONE: 145 return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 146 case ONHOLD: 147 return "The event has been temporarily stopped but is expected to resume in the future."; 148 case STOPPED: 149 return "The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred."; 150 case COMPLETED: 151 return "The event has now concluded."; 152 case ENTEREDINERROR: 153 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 154 case UNKNOWN: 155 return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 163 public String getDisplay() { 164 switch (this) { 165 case PREPARATION: 166 return "Preparation"; 167 case INPROGRESS: 168 return "In Progress"; 169 case NOTDONE: 170 return "Not Done"; 171 case ONHOLD: 172 return "On Hold"; 173 case STOPPED: 174 return "Stopped"; 175 case COMPLETED: 176 return "Completed"; 177 case ENTEREDINERROR: 178 return "Entered in Error"; 179 case UNKNOWN: 180 return "Unknown"; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188}