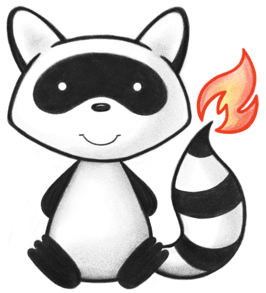
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum EventTiming { 037 038 /** 039 * Event occurs during the morning. The exact time is unspecified and 040 * established by institution convention or patient interpretation. 041 */ 042 MORN, 043 /** 044 * Event occurs during the early morning. The exact time is unspecified and 045 * established by institution convention or patient interpretation. 046 */ 047 MORN_EARLY, 048 /** 049 * Event occurs during the late morning. The exact time is unspecified and 050 * established by institution convention or patient interpretation. 051 */ 052 MORN_LATE, 053 /** 054 * Event occurs around 12:00pm. The exact time is unspecified and established by 055 * institution convention or patient interpretation. 056 */ 057 NOON, 058 /** 059 * Event occurs during the afternoon. The exact time is unspecified and 060 * established by institution convention or patient interpretation. 061 */ 062 AFT, 063 /** 064 * Event occurs during the early afternoon. The exact time is unspecified and 065 * established by institution convention or patient interpretation. 066 */ 067 AFT_EARLY, 068 /** 069 * Event occurs during the late afternoon. The exact time is unspecified and 070 * established by institution convention or patient interpretation. 071 */ 072 AFT_LATE, 073 /** 074 * Event occurs during the evening. The exact time is unspecified and 075 * established by institution convention or patient interpretation. 076 */ 077 EVE, 078 /** 079 * Event occurs during the early evening. The exact time is unspecified and 080 * established by institution convention or patient interpretation. 081 */ 082 EVE_EARLY, 083 /** 084 * Event occurs during the late evening. The exact time is unspecified and 085 * established by institution convention or patient interpretation. 086 */ 087 EVE_LATE, 088 /** 089 * Event occurs during the night. The exact time is unspecified and established 090 * by institution convention or patient interpretation. 091 */ 092 NIGHT, 093 /** 094 * Event occurs [offset] after subject goes to sleep. The exact time is 095 * unspecified and established by institution convention or patient 096 * interpretation. 097 */ 098 PHS, 099 /** 100 * added to help the parsers 101 */ 102 NULL; 103 104 public static EventTiming fromCode(String codeString) throws FHIRException { 105 if (codeString == null || "".equals(codeString)) 106 return null; 107 if ("MORN".equals(codeString)) 108 return MORN; 109 if ("MORN.early".equals(codeString)) 110 return MORN_EARLY; 111 if ("MORN.late".equals(codeString)) 112 return MORN_LATE; 113 if ("NOON".equals(codeString)) 114 return NOON; 115 if ("AFT".equals(codeString)) 116 return AFT; 117 if ("AFT.early".equals(codeString)) 118 return AFT_EARLY; 119 if ("AFT.late".equals(codeString)) 120 return AFT_LATE; 121 if ("EVE".equals(codeString)) 122 return EVE; 123 if ("EVE.early".equals(codeString)) 124 return EVE_EARLY; 125 if ("EVE.late".equals(codeString)) 126 return EVE_LATE; 127 if ("NIGHT".equals(codeString)) 128 return NIGHT; 129 if ("PHS".equals(codeString)) 130 return PHS; 131 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 132 } 133 134 public String toCode() { 135 switch (this) { 136 case MORN: 137 return "MORN"; 138 case MORN_EARLY: 139 return "MORN.early"; 140 case MORN_LATE: 141 return "MORN.late"; 142 case NOON: 143 return "NOON"; 144 case AFT: 145 return "AFT"; 146 case AFT_EARLY: 147 return "AFT.early"; 148 case AFT_LATE: 149 return "AFT.late"; 150 case EVE: 151 return "EVE"; 152 case EVE_EARLY: 153 return "EVE.early"; 154 case EVE_LATE: 155 return "EVE.late"; 156 case NIGHT: 157 return "NIGHT"; 158 case PHS: 159 return "PHS"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getSystem() { 168 return "http://hl7.org/fhir/event-timing"; 169 } 170 171 public String getDefinition() { 172 switch (this) { 173 case MORN: 174 return "Event occurs during the morning. The exact time is unspecified and established by institution convention or patient interpretation."; 175 case MORN_EARLY: 176 return "Event occurs during the early morning. The exact time is unspecified and established by institution convention or patient interpretation."; 177 case MORN_LATE: 178 return "Event occurs during the late morning. The exact time is unspecified and established by institution convention or patient interpretation."; 179 case NOON: 180 return "Event occurs around 12:00pm. The exact time is unspecified and established by institution convention or patient interpretation."; 181 case AFT: 182 return "Event occurs during the afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 183 case AFT_EARLY: 184 return "Event occurs during the early afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 185 case AFT_LATE: 186 return "Event occurs during the late afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 187 case EVE: 188 return "Event occurs during the evening. The exact time is unspecified and established by institution convention or patient interpretation."; 189 case EVE_EARLY: 190 return "Event occurs during the early evening. The exact time is unspecified and established by institution convention or patient interpretation."; 191 case EVE_LATE: 192 return "Event occurs during the late evening. The exact time is unspecified and established by institution convention or patient interpretation."; 193 case NIGHT: 194 return "Event occurs during the night. The exact time is unspecified and established by institution convention or patient interpretation."; 195 case PHS: 196 return "Event occurs [offset] after subject goes to sleep. The exact time is unspecified and established by institution convention or patient interpretation."; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204 public String getDisplay() { 205 switch (this) { 206 case MORN: 207 return "Morning"; 208 case MORN_EARLY: 209 return "Early Morning"; 210 case MORN_LATE: 211 return "Late Morning"; 212 case NOON: 213 return "Noon"; 214 case AFT: 215 return "Afternoon"; 216 case AFT_EARLY: 217 return "Early Afternoon"; 218 case AFT_LATE: 219 return "Late Afternoon"; 220 case EVE: 221 return "Evening"; 222 case EVE_EARLY: 223 return "Early Evening"; 224 case EVE_LATE: 225 return "Late Evening"; 226 case NIGHT: 227 return "Night"; 228 case PHS: 229 return "After Sleep"; 230 case NULL: 231 return null; 232 default: 233 return "?"; 234 } 235 } 236 237}