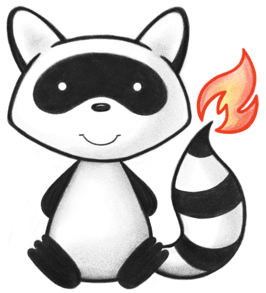
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ExBenefitcategory { 037 038 /** 039 * Medical Care. 040 */ 041 _1, 042 /** 043 * Surgical. 044 */ 045 _2, 046 /** 047 * Consultation. 048 */ 049 _3, 050 /** 051 * Diagnostic XRay. 052 */ 053 _4, 054 /** 055 * Diagnostic Lab. 056 */ 057 _5, 058 /** 059 * Renal Supplies excluding Dialysis. 060 */ 061 _14, 062 /** 063 * Diagnostic Dental. 064 */ 065 _23, 066 /** 067 * Periodontics. 068 */ 069 _24, 070 /** 071 * Restorative. 072 */ 073 _25, 074 /** 075 * Endodontics. 076 */ 077 _26, 078 /** 079 * Maxillofacial Prosthetics. 080 */ 081 _27, 082 /** 083 * Adjunctive Dental Services. 084 */ 085 _28, 086 /** 087 * Health Benefit Plan Coverage. 088 */ 089 _30, 090 /** 091 * Dental Care. 092 */ 093 _35, 094 /** 095 * Dental Crowns. 096 */ 097 _36, 098 /** 099 * Dental Accident. 100 */ 101 _37, 102 /** 103 * Hospital Room and Board. 104 */ 105 _49, 106 /** 107 * Major Medical. 108 */ 109 _55, 110 /** 111 * Medically Related Transportation. 112 */ 113 _56, 114 /** 115 * In-vitro Fertilization. 116 */ 117 _61, 118 /** 119 * MRI Scan. 120 */ 121 _62, 122 /** 123 * Donor Procedures such as organ harvest. 124 */ 125 _63, 126 /** 127 * Maternity. 128 */ 129 _69, 130 /** 131 * Renal dialysis. 132 */ 133 _76, 134 /** 135 * Medical Coverage. 136 */ 137 F1, 138 /** 139 * Dental Coverage. 140 */ 141 F3, 142 /** 143 * Hearing Coverage. 144 */ 145 F4, 146 /** 147 * Vision Coverage. 148 */ 149 F6, 150 /** 151 * added to help the parsers 152 */ 153 NULL; 154 155 public static ExBenefitcategory fromCode(String codeString) throws FHIRException { 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("1".equals(codeString)) 159 return _1; 160 if ("2".equals(codeString)) 161 return _2; 162 if ("3".equals(codeString)) 163 return _3; 164 if ("4".equals(codeString)) 165 return _4; 166 if ("5".equals(codeString)) 167 return _5; 168 if ("14".equals(codeString)) 169 return _14; 170 if ("23".equals(codeString)) 171 return _23; 172 if ("24".equals(codeString)) 173 return _24; 174 if ("25".equals(codeString)) 175 return _25; 176 if ("26".equals(codeString)) 177 return _26; 178 if ("27".equals(codeString)) 179 return _27; 180 if ("28".equals(codeString)) 181 return _28; 182 if ("30".equals(codeString)) 183 return _30; 184 if ("35".equals(codeString)) 185 return _35; 186 if ("36".equals(codeString)) 187 return _36; 188 if ("37".equals(codeString)) 189 return _37; 190 if ("49".equals(codeString)) 191 return _49; 192 if ("55".equals(codeString)) 193 return _55; 194 if ("56".equals(codeString)) 195 return _56; 196 if ("61".equals(codeString)) 197 return _61; 198 if ("62".equals(codeString)) 199 return _62; 200 if ("63".equals(codeString)) 201 return _63; 202 if ("69".equals(codeString)) 203 return _69; 204 if ("76".equals(codeString)) 205 return _76; 206 if ("F1".equals(codeString)) 207 return F1; 208 if ("F3".equals(codeString)) 209 return F3; 210 if ("F4".equals(codeString)) 211 return F4; 212 if ("F6".equals(codeString)) 213 return F6; 214 throw new FHIRException("Unknown ExBenefitcategory code '" + codeString + "'"); 215 } 216 217 public String toCode() { 218 switch (this) { 219 case _1: 220 return "1"; 221 case _2: 222 return "2"; 223 case _3: 224 return "3"; 225 case _4: 226 return "4"; 227 case _5: 228 return "5"; 229 case _14: 230 return "14"; 231 case _23: 232 return "23"; 233 case _24: 234 return "24"; 235 case _25: 236 return "25"; 237 case _26: 238 return "26"; 239 case _27: 240 return "27"; 241 case _28: 242 return "28"; 243 case _30: 244 return "30"; 245 case _35: 246 return "35"; 247 case _36: 248 return "36"; 249 case _37: 250 return "37"; 251 case _49: 252 return "49"; 253 case _55: 254 return "55"; 255 case _56: 256 return "56"; 257 case _61: 258 return "61"; 259 case _62: 260 return "62"; 261 case _63: 262 return "63"; 263 case _69: 264 return "69"; 265 case _76: 266 return "76"; 267 case F1: 268 return "F1"; 269 case F3: 270 return "F3"; 271 case F4: 272 return "F4"; 273 case F6: 274 return "F6"; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getSystem() { 283 return "http://terminology.hl7.org/CodeSystem/ex-benefitcategory"; 284 } 285 286 public String getDefinition() { 287 switch (this) { 288 case _1: 289 return "Medical Care."; 290 case _2: 291 return "Surgical."; 292 case _3: 293 return "Consultation."; 294 case _4: 295 return "Diagnostic XRay."; 296 case _5: 297 return "Diagnostic Lab."; 298 case _14: 299 return "Renal Supplies excluding Dialysis."; 300 case _23: 301 return "Diagnostic Dental."; 302 case _24: 303 return "Periodontics."; 304 case _25: 305 return "Restorative."; 306 case _26: 307 return "Endodontics."; 308 case _27: 309 return "Maxillofacial Prosthetics."; 310 case _28: 311 return "Adjunctive Dental Services."; 312 case _30: 313 return "Health Benefit Plan Coverage."; 314 case _35: 315 return "Dental Care."; 316 case _36: 317 return "Dental Crowns."; 318 case _37: 319 return "Dental Accident."; 320 case _49: 321 return "Hospital Room and Board."; 322 case _55: 323 return "Major Medical."; 324 case _56: 325 return "Medically Related Transportation."; 326 case _61: 327 return "In-vitro Fertilization."; 328 case _62: 329 return "MRI Scan."; 330 case _63: 331 return "Donor Procedures such as organ harvest."; 332 case _69: 333 return "Maternity."; 334 case _76: 335 return "Renal dialysis."; 336 case F1: 337 return "Medical Coverage."; 338 case F3: 339 return "Dental Coverage."; 340 case F4: 341 return "Hearing Coverage."; 342 case F6: 343 return "Vision Coverage."; 344 case NULL: 345 return null; 346 default: 347 return "?"; 348 } 349 } 350 351 public String getDisplay() { 352 switch (this) { 353 case _1: 354 return "Medical Care"; 355 case _2: 356 return "Surgical"; 357 case _3: 358 return "Consultation"; 359 case _4: 360 return "Diagnostic XRay"; 361 case _5: 362 return "Diagnostic Lab"; 363 case _14: 364 return "Renal Supplies"; 365 case _23: 366 return "Diagnostic Dental"; 367 case _24: 368 return "Periodontics"; 369 case _25: 370 return "Restorative"; 371 case _26: 372 return "Endodontics"; 373 case _27: 374 return "Maxillofacial Prosthetics"; 375 case _28: 376 return "Adjunctive Dental Services"; 377 case _30: 378 return "Health Benefit Plan Coverage"; 379 case _35: 380 return "Dental Care"; 381 case _36: 382 return "Dental Crowns"; 383 case _37: 384 return "Dental Accident"; 385 case _49: 386 return "Hospital Room and Board"; 387 case _55: 388 return "Major Medical"; 389 case _56: 390 return "Medically Related Transportation"; 391 case _61: 392 return "In-vitro Fertilization"; 393 case _62: 394 return "MRI Scan"; 395 case _63: 396 return "Donor Procedures"; 397 case _69: 398 return "Maternity"; 399 case _76: 400 return "Renal Dialysis"; 401 case F1: 402 return "Medical Coverage"; 403 case F3: 404 return "Dental Coverage"; 405 case F4: 406 return "Hearing Coverage"; 407 case F6: 408 return "Vision Coverage"; 409 case NULL: 410 return null; 411 default: 412 return "?"; 413 } 414 } 415 416}