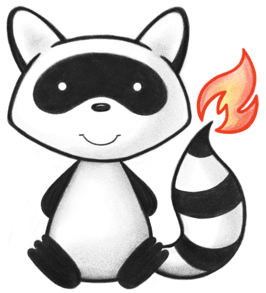
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ExDiagnosistype { 037 038 /** 039 * The diagnosis given as the reason why the patient was admitted to the 040 * hospital. 041 */ 042 ADMITTING, 043 /** 044 * A diagnosis made on the basis of medical signs and patient-reported symptoms, 045 * rather than diagnostic tests. 046 */ 047 CLINICAL, 048 /** 049 * One of a set of the possible diagnoses that could be connected to the signs, 050 * symptoms, and lab findings. 051 */ 052 DIFFERENTIAL, 053 /** 054 * The diagnosis given when the patient is discharged from the hospital. 055 */ 056 DISCHARGE, 057 /** 058 * A diagnosis based significantly on laboratory reports or test results, rather 059 * than the physical examination of the patient. 060 */ 061 LABORATORY, 062 /** 063 * A diagnosis which identifies people's responses to situations in their lives, 064 * such as a readiness to change or a willingness to accept assistance. 065 */ 066 NURSING, 067 /** 068 * A diagnosis determined prior to birth. 069 */ 070 PRENATAL, 071 /** 072 * The single medical diagnosis that is most relevant to the patient's chief 073 * complaint or need for treatment. 074 */ 075 PRINCIPAL, 076 /** 077 * A diagnosis based primarily on the results from medical imaging studies. 078 */ 079 RADIOLOGY, 080 /** 081 * A diagnosis determined using telemedicine techniques. 082 */ 083 REMOTE, 084 /** 085 * The labeling of an illness in a specific historical event using modern 086 * knowledge, methods and disease classifications. 087 */ 088 RETROSPECTIVE, 089 /** 090 * A diagnosis determined by the patient. 091 */ 092 SELF, 093 /** 094 * added to help the parsers 095 */ 096 NULL; 097 098 public static ExDiagnosistype fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("admitting".equals(codeString)) 102 return ADMITTING; 103 if ("clinical".equals(codeString)) 104 return CLINICAL; 105 if ("differential".equals(codeString)) 106 return DIFFERENTIAL; 107 if ("discharge".equals(codeString)) 108 return DISCHARGE; 109 if ("laboratory".equals(codeString)) 110 return LABORATORY; 111 if ("nursing".equals(codeString)) 112 return NURSING; 113 if ("prenatal".equals(codeString)) 114 return PRENATAL; 115 if ("principal".equals(codeString)) 116 return PRINCIPAL; 117 if ("radiology".equals(codeString)) 118 return RADIOLOGY; 119 if ("remote".equals(codeString)) 120 return REMOTE; 121 if ("retrospective".equals(codeString)) 122 return RETROSPECTIVE; 123 if ("self".equals(codeString)) 124 return SELF; 125 throw new FHIRException("Unknown ExDiagnosistype code '" + codeString + "'"); 126 } 127 128 public String toCode() { 129 switch (this) { 130 case ADMITTING: 131 return "admitting"; 132 case CLINICAL: 133 return "clinical"; 134 case DIFFERENTIAL: 135 return "differential"; 136 case DISCHARGE: 137 return "discharge"; 138 case LABORATORY: 139 return "laboratory"; 140 case NURSING: 141 return "nursing"; 142 case PRENATAL: 143 return "prenatal"; 144 case PRINCIPAL: 145 return "principal"; 146 case RADIOLOGY: 147 return "radiology"; 148 case REMOTE: 149 return "remote"; 150 case RETROSPECTIVE: 151 return "retrospective"; 152 case SELF: 153 return "self"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getSystem() { 162 return "http://terminology.hl7.org/CodeSystem/ex-diagnosistype"; 163 } 164 165 public String getDefinition() { 166 switch (this) { 167 case ADMITTING: 168 return "The diagnosis given as the reason why the patient was admitted to the hospital."; 169 case CLINICAL: 170 return "A diagnosis made on the basis of medical signs and patient-reported symptoms, rather than diagnostic tests."; 171 case DIFFERENTIAL: 172 return "One of a set of the possible diagnoses that could be connected to the signs, symptoms, and lab findings."; 173 case DISCHARGE: 174 return "The diagnosis given when the patient is discharged from the hospital."; 175 case LABORATORY: 176 return "A diagnosis based significantly on laboratory reports or test results, rather than the physical examination of the patient."; 177 case NURSING: 178 return "A diagnosis which identifies people's responses to situations in their lives, such as a readiness to change or a willingness to accept assistance."; 179 case PRENATAL: 180 return "A diagnosis determined prior to birth."; 181 case PRINCIPAL: 182 return "The single medical diagnosis that is most relevant to the patient's chief complaint or need for treatment."; 183 case RADIOLOGY: 184 return "A diagnosis based primarily on the results from medical imaging studies."; 185 case REMOTE: 186 return "A diagnosis determined using telemedicine techniques."; 187 case RETROSPECTIVE: 188 return "The labeling of an illness in a specific historical event using modern knowledge, methods and disease classifications."; 189 case SELF: 190 return "A diagnosis determined by the patient."; 191 case NULL: 192 return null; 193 default: 194 return "?"; 195 } 196 } 197 198 public String getDisplay() { 199 switch (this) { 200 case ADMITTING: 201 return "Admitting Diagnosis"; 202 case CLINICAL: 203 return "Clinical Diagnosis"; 204 case DIFFERENTIAL: 205 return "Differential Diagnosis"; 206 case DISCHARGE: 207 return "Discharge Diagnosis"; 208 case LABORATORY: 209 return "Laboratory Diagnosis"; 210 case NURSING: 211 return "Nursing Diagnosis"; 212 case PRENATAL: 213 return "Prenatal Diagnosis"; 214 case PRINCIPAL: 215 return "Principal Diagnosis"; 216 case RADIOLOGY: 217 return "Radiology Diagnosis"; 218 case REMOTE: 219 return "Remote Diagnosis"; 220 case RETROSPECTIVE: 221 return "Retrospective Diagnosis"; 222 case SELF: 223 return "Self Diagnosis"; 224 case NULL: 225 return null; 226 default: 227 return "?"; 228 } 229 } 230 231}