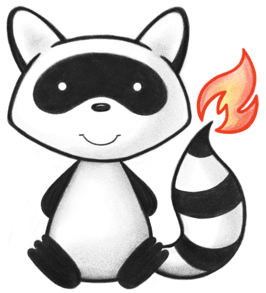
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ExtraSecurityRoleType { 037 038 /** 039 * An entity providing authorization services to enable the electronic sharing 040 * of health-related information based on resource owner's preapproved 041 * permissions. For example, an UMA Authorization Server[UMA] 042 */ 043 AUTHSERVER, 044 /** 045 * An entity that collects information over which the data subject may have 046 * certain rights under policy or law to control that information's management 047 * and distribution by data collectors, including the right to access, retrieve, 048 * distribute, or delete that information. 049 */ 050 DATACOLLECTOR, 051 /** 052 * An entity that processes collected information over which the data subject 053 * may have certain rights under policy or law to control that information's 054 * management and distribution by data processors, including the right to 055 * access, retrieve, distribute, or delete that information. 056 */ 057 DATAPROCESSOR, 058 /** 059 * A person whose personal information is collected or processed, and who may 060 * have certain rights under policy or law to control that information's 061 * management and distribution by data collectors or processors, including the 062 * right to access, retrieve, distribute, or delete that information. 063 */ 064 DATASUBJECT, 065 /** 066 * The human user that has participated. 067 */ 068 HUMANUSER, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static ExtraSecurityRoleType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("authserver".equals(codeString)) 078 return AUTHSERVER; 079 if ("datacollector".equals(codeString)) 080 return DATACOLLECTOR; 081 if ("dataprocessor".equals(codeString)) 082 return DATAPROCESSOR; 083 if ("datasubject".equals(codeString)) 084 return DATASUBJECT; 085 if ("humanuser".equals(codeString)) 086 return HUMANUSER; 087 throw new FHIRException("Unknown ExtraSecurityRoleType code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case AUTHSERVER: 093 return "authserver"; 094 case DATACOLLECTOR: 095 return "datacollector"; 096 case DATAPROCESSOR: 097 return "dataprocessor"; 098 case DATASUBJECT: 099 return "datasubject"; 100 case HUMANUSER: 101 return "humanuser"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 return "http://terminology.hl7.org/CodeSystem/extra-security-role-type"; 111 } 112 113 public String getDefinition() { 114 switch (this) { 115 case AUTHSERVER: 116 return "An entity providing authorization services to enable the electronic sharing of health-related information based on resource owner's preapproved permissions. For example, an UMA Authorization Server[UMA]"; 117 case DATACOLLECTOR: 118 return "An entity that collects information over which the data subject may have certain rights under policy or law to control that information's management and distribution by data collectors, including the right to access, retrieve, distribute, or delete that information. "; 119 case DATAPROCESSOR: 120 return "An entity that processes collected information over which the data subject may have certain rights under policy or law to control that information's management and distribution by data processors, including the right to access, retrieve, distribute, or delete that information."; 121 case DATASUBJECT: 122 return "A person whose personal information is collected or processed, and who may have certain rights under policy or law to control that information's management and distribution by data collectors or processors, including the right to access, retrieve, distribute, or delete that information."; 123 case HUMANUSER: 124 return "The human user that has participated."; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case AUTHSERVER: 135 return "authorization server"; 136 case DATACOLLECTOR: 137 return "data collector"; 138 case DATAPROCESSOR: 139 return "data processor"; 140 case DATASUBJECT: 141 return "data subject"; 142 case HUMANUSER: 143 return "human user"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151}