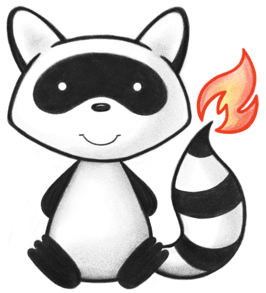
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum FeedingDevice { 037 038 /** 039 * Standard nipple definition: 040 */ 041 STANDARDNIPPLE, 042 /** 043 * Preemie nipple definition: 044 */ 045 PREEMIENIPPLE, 046 /** 047 * Orthodontic nipple definition: 048 */ 049 ORTHONIPPLE, 050 /** 051 * Slow flow nipple definition: 052 */ 053 SLOFLONIPPLE, 054 /** 055 * Middle flow nipple definition: 056 */ 057 MIDFLONIPPLE, 058 /** 059 * Enlarged, cross-cut nipple definition: 060 */ 061 BIGCUTNIPPLE, 062 /** 063 * Haberman bottle definition: 064 */ 065 HABERMANBOTTLE, 066 /** 067 * Sippy cup with valve definition: 068 */ 069 SIPPYVALVE, 070 /** 071 * Sippy cup without valve definition: 072 */ 073 SIPPYNOVALVE, 074 /** 075 * Provale Cup definition: 076 */ 077 PROVALECUP, 078 /** 079 * Glass with lid/sippy cup definition: 080 */ 081 GLASSLID, 082 /** 083 * Double handhold on glass/cup definition: 084 */ 085 HANDHOLDCUP, 086 /** 087 * Rubber matting under tray definition: 088 */ 089 RUBBERMAT, 090 /** 091 * Straw definition: 092 */ 093 STRAW, 094 /** 095 * Nose cup definition: 096 */ 097 NOSECUP, 098 /** 099 * Scoop plate definition: 100 */ 101 SCOOPPLATE, 102 /** 103 * Hand wrap utensil holder definition: 104 */ 105 UTENSILHOLDER, 106 /** 107 * Foam handle utensils definition: 108 */ 109 FOAMHANDLE, 110 /** 111 * Angled utensils definition: 112 */ 113 ANGLEDUTENSIL, 114 /** 115 * Spout cup definition: 116 */ 117 SPOUTCUP, 118 /** 119 * Automated feeding devices definition: 120 */ 121 AUTOFEEDINGDEVICE, 122 /** 123 * Rocker knife definition: 124 */ 125 ROCKERKNIFE, 126 /** 127 * added to help the parsers 128 */ 129 NULL; 130 131 public static FeedingDevice fromCode(String codeString) throws FHIRException { 132 if (codeString == null || "".equals(codeString)) 133 return null; 134 if ("standard-nipple".equals(codeString)) 135 return STANDARDNIPPLE; 136 if ("preemie-nipple".equals(codeString)) 137 return PREEMIENIPPLE; 138 if ("ortho-nipple".equals(codeString)) 139 return ORTHONIPPLE; 140 if ("sloflo-nipple".equals(codeString)) 141 return SLOFLONIPPLE; 142 if ("midflo-nipple".equals(codeString)) 143 return MIDFLONIPPLE; 144 if ("bigcut-nipple".equals(codeString)) 145 return BIGCUTNIPPLE; 146 if ("haberman-bottle".equals(codeString)) 147 return HABERMANBOTTLE; 148 if ("sippy-valve".equals(codeString)) 149 return SIPPYVALVE; 150 if ("sippy-no-valve".equals(codeString)) 151 return SIPPYNOVALVE; 152 if ("provale-cup".equals(codeString)) 153 return PROVALECUP; 154 if ("glass-lid".equals(codeString)) 155 return GLASSLID; 156 if ("handhold-cup".equals(codeString)) 157 return HANDHOLDCUP; 158 if ("rubber-mat".equals(codeString)) 159 return RUBBERMAT; 160 if ("straw".equals(codeString)) 161 return STRAW; 162 if ("nose-cup".equals(codeString)) 163 return NOSECUP; 164 if ("scoop-plate".equals(codeString)) 165 return SCOOPPLATE; 166 if ("utensil-holder".equals(codeString)) 167 return UTENSILHOLDER; 168 if ("foam-handle".equals(codeString)) 169 return FOAMHANDLE; 170 if ("angled-utensil".equals(codeString)) 171 return ANGLEDUTENSIL; 172 if ("spout-cup".equals(codeString)) 173 return SPOUTCUP; 174 if ("autofeeding-device".equals(codeString)) 175 return AUTOFEEDINGDEVICE; 176 if ("rocker-knife".equals(codeString)) 177 return ROCKERKNIFE; 178 throw new FHIRException("Unknown FeedingDevice code '" + codeString + "'"); 179 } 180 181 public String toCode() { 182 switch (this) { 183 case STANDARDNIPPLE: 184 return "standard-nipple"; 185 case PREEMIENIPPLE: 186 return "preemie-nipple"; 187 case ORTHONIPPLE: 188 return "ortho-nipple"; 189 case SLOFLONIPPLE: 190 return "sloflo-nipple"; 191 case MIDFLONIPPLE: 192 return "midflo-nipple"; 193 case BIGCUTNIPPLE: 194 return "bigcut-nipple"; 195 case HABERMANBOTTLE: 196 return "haberman-bottle"; 197 case SIPPYVALVE: 198 return "sippy-valve"; 199 case SIPPYNOVALVE: 200 return "sippy-no-valve"; 201 case PROVALECUP: 202 return "provale-cup"; 203 case GLASSLID: 204 return "glass-lid"; 205 case HANDHOLDCUP: 206 return "handhold-cup"; 207 case RUBBERMAT: 208 return "rubber-mat"; 209 case STRAW: 210 return "straw"; 211 case NOSECUP: 212 return "nose-cup"; 213 case SCOOPPLATE: 214 return "scoop-plate"; 215 case UTENSILHOLDER: 216 return "utensil-holder"; 217 case FOAMHANDLE: 218 return "foam-handle"; 219 case ANGLEDUTENSIL: 220 return "angled-utensil"; 221 case SPOUTCUP: 222 return "spout-cup"; 223 case AUTOFEEDINGDEVICE: 224 return "autofeeding-device"; 225 case ROCKERKNIFE: 226 return "rocker-knife"; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 234 public String getSystem() { 235 return "http://hl7.org/fhir/feeding-device"; 236 } 237 238 public String getDefinition() { 239 switch (this) { 240 case STANDARDNIPPLE: 241 return "Standard nipple definition:"; 242 case PREEMIENIPPLE: 243 return "Preemie nipple definition:"; 244 case ORTHONIPPLE: 245 return "Orthodontic nipple definition:"; 246 case SLOFLONIPPLE: 247 return "Slow flow nipple definition:"; 248 case MIDFLONIPPLE: 249 return "Middle flow nipple definition:"; 250 case BIGCUTNIPPLE: 251 return "Enlarged, cross-cut nipple definition:"; 252 case HABERMANBOTTLE: 253 return "Haberman bottle definition:"; 254 case SIPPYVALVE: 255 return "Sippy cup with valve definition:"; 256 case SIPPYNOVALVE: 257 return "Sippy cup without valve definition:"; 258 case PROVALECUP: 259 return "Provale Cup definition:"; 260 case GLASSLID: 261 return "Glass with lid/sippy cup definition:"; 262 case HANDHOLDCUP: 263 return "Double handhold on glass/cup definition:"; 264 case RUBBERMAT: 265 return "Rubber matting under tray definition:"; 266 case STRAW: 267 return "Straw definition:"; 268 case NOSECUP: 269 return "Nose cup definition:"; 270 case SCOOPPLATE: 271 return "Scoop plate definition:"; 272 case UTENSILHOLDER: 273 return "Hand wrap utensil holder definition:"; 274 case FOAMHANDLE: 275 return "Foam handle utensils definition:"; 276 case ANGLEDUTENSIL: 277 return "Angled utensils definition:"; 278 case SPOUTCUP: 279 return "Spout cup definition:"; 280 case AUTOFEEDINGDEVICE: 281 return "Automated feeding devices definition:"; 282 case ROCKERKNIFE: 283 return "Rocker knife definition:"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 291 public String getDisplay() { 292 switch (this) { 293 case STANDARDNIPPLE: 294 return "Standard nipple"; 295 case PREEMIENIPPLE: 296 return "Preemie nipple"; 297 case ORTHONIPPLE: 298 return "Orthodontic nipple"; 299 case SLOFLONIPPLE: 300 return "Slow flow nipple"; 301 case MIDFLONIPPLE: 302 return "Middle flow nipple"; 303 case BIGCUTNIPPLE: 304 return "Enlarged, cross-cut nipple"; 305 case HABERMANBOTTLE: 306 return "Haberman bottle"; 307 case SIPPYVALVE: 308 return "Sippy cup with valve"; 309 case SIPPYNOVALVE: 310 return "Sippy cup without valve"; 311 case PROVALECUP: 312 return "Provale Cup"; 313 case GLASSLID: 314 return "Glass with lid/sippy cup"; 315 case HANDHOLDCUP: 316 return "Double handhold on glass/cup"; 317 case RUBBERMAT: 318 return "Rubber matting under tray"; 319 case STRAW: 320 return "Straw"; 321 case NOSECUP: 322 return "Nose cup"; 323 case SCOOPPLATE: 324 return "Scoop plate"; 325 case UTENSILHOLDER: 326 return "Hand wrap utensil holder"; 327 case FOAMHANDLE: 328 return "Foam handle utensils"; 329 case ANGLEDUTENSIL: 330 return "Angled utensils"; 331 case SPOUTCUP: 332 return "Spout cup"; 333 case AUTOFEEDINGDEVICE: 334 return "Automated feeding devices"; 335 case ROCKERKNIFE: 336 return "Rocker knife"; 337 case NULL: 338 return null; 339 default: 340 return "?"; 341 } 342 } 343 344}