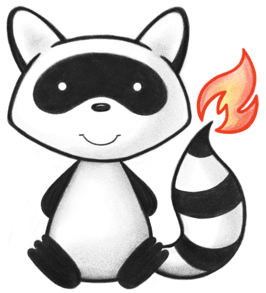
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum FilterOperator { 037 038 /** 039 * The specified property of the code equals the provided value. 040 */ 041 EQUAL, 042 /** 043 * Includes all concept ids that have a transitive is-a relationship with the 044 * concept Id provided as the value, including the provided concept itself 045 * (include descendant codes and self). 046 */ 047 ISA, 048 /** 049 * Includes all concept ids that have a transitive is-a relationship with the 050 * concept Id provided as the value, excluding the provided concept itself i.e. 051 * include descendant codes only). 052 */ 053 DESCENDENTOF, 054 /** 055 * The specified property of the code does not have an is-a relationship with 056 * the provided value. 057 */ 058 ISNOTA, 059 /** 060 * The specified property of the code matches the regex specified in the 061 * provided value. 062 */ 063 REGEX, 064 /** 065 * The specified property of the code is in the set of codes or concepts 066 * specified in the provided value (comma separated list). 067 */ 068 IN, 069 /** 070 * The specified property of the code is not in the set of codes or concepts 071 * specified in the provided value (comma separated list). 072 */ 073 NOTIN, 074 /** 075 * Includes all concept ids that have a transitive is-a relationship from the 076 * concept Id provided as the value, including the provided concept itself (i.e. 077 * include ancestor codes and self). 078 */ 079 GENERALIZES, 080 /** 081 * The specified property of the code has at least one value (if the specified 082 * value is true; if the specified value is false, then matches when the 083 * specified property of the code has no values). 084 */ 085 EXISTS, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 091 public static FilterOperator fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("=".equals(codeString)) 095 return EQUAL; 096 if ("is-a".equals(codeString)) 097 return ISA; 098 if ("descendent-of".equals(codeString)) 099 return DESCENDENTOF; 100 if ("is-not-a".equals(codeString)) 101 return ISNOTA; 102 if ("regex".equals(codeString)) 103 return REGEX; 104 if ("in".equals(codeString)) 105 return IN; 106 if ("not-in".equals(codeString)) 107 return NOTIN; 108 if ("generalizes".equals(codeString)) 109 return GENERALIZES; 110 if ("exists".equals(codeString)) 111 return EXISTS; 112 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case EQUAL: 118 return "="; 119 case ISA: 120 return "is-a"; 121 case DESCENDENTOF: 122 return "descendent-of"; 123 case ISNOTA: 124 return "is-not-a"; 125 case REGEX: 126 return "regex"; 127 case IN: 128 return "in"; 129 case NOTIN: 130 return "not-in"; 131 case GENERALIZES: 132 return "generalizes"; 133 case EXISTS: 134 return "exists"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getSystem() { 143 return "http://hl7.org/fhir/filter-operator"; 144 } 145 146 public String getDefinition() { 147 switch (this) { 148 case EQUAL: 149 return "The specified property of the code equals the provided value."; 150 case ISA: 151 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (include descendant codes and self)."; 152 case DESCENDENTOF: 153 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself i.e. include descendant codes only)."; 154 case ISNOTA: 155 return "The specified property of the code does not have an is-a relationship with the provided value."; 156 case REGEX: 157 return "The specified property of the code matches the regex specified in the provided value."; 158 case IN: 159 return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 160 case NOTIN: 161 return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 162 case GENERALIZES: 163 return "Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (i.e. include ancestor codes and self)."; 164 case EXISTS: 165 return "The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values)."; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 173 public String getDisplay() { 174 switch (this) { 175 case EQUAL: 176 return "Equals"; 177 case ISA: 178 return "Is A (by subsumption)"; 179 case DESCENDENTOF: 180 return "Descendent Of (by subsumption)"; 181 case ISNOTA: 182 return "Not (Is A) (by subsumption)"; 183 case REGEX: 184 return "Regular Expression"; 185 case IN: 186 return "In Set"; 187 case NOTIN: 188 return "Not in Set"; 189 case GENERALIZES: 190 return "Generalizes (by Subsumption)"; 191 case EXISTS: 192 return "Exists"; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 200}