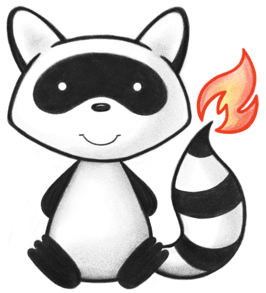
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum FlagCategory { 037 038 /** 039 * Flags related to the subject's dietary needs. 040 */ 041 DIET, 042 /** 043 * Flags related to the subject's medications. 044 */ 045 DRUG, 046 /** 047 * Flags related to performing laboratory tests and related processes (e.g. 048 * phlebotomy). 049 */ 050 LAB, 051 /** 052 * Flags related to administrative and financial processes. 053 */ 054 ADMIN, 055 /** 056 * Flags related to coming into contact with the patient. 057 */ 058 CONTACT, 059 /** 060 * Flags related to the subject's clinical data. 061 */ 062 CLINICAL, 063 /** 064 * Flags related to behavior. 065 */ 066 BEHAVIORAL, 067 /** 068 * Flags related to research. 069 */ 070 RESEARCH, 071 /** 072 * Flags related to subject's advance directives. 073 */ 074 ADVANCEDIRECTIVE, 075 /** 076 * Flags related to safety precautions. 077 */ 078 SAFETY, 079 /** 080 * added to help the parsers 081 */ 082 NULL; 083 084 public static FlagCategory fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("diet".equals(codeString)) 088 return DIET; 089 if ("drug".equals(codeString)) 090 return DRUG; 091 if ("lab".equals(codeString)) 092 return LAB; 093 if ("admin".equals(codeString)) 094 return ADMIN; 095 if ("contact".equals(codeString)) 096 return CONTACT; 097 if ("clinical".equals(codeString)) 098 return CLINICAL; 099 if ("behavioral".equals(codeString)) 100 return BEHAVIORAL; 101 if ("research".equals(codeString)) 102 return RESEARCH; 103 if ("advance-directive".equals(codeString)) 104 return ADVANCEDIRECTIVE; 105 if ("safety".equals(codeString)) 106 return SAFETY; 107 throw new FHIRException("Unknown FlagCategory code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case DIET: 113 return "diet"; 114 case DRUG: 115 return "drug"; 116 case LAB: 117 return "lab"; 118 case ADMIN: 119 return "admin"; 120 case CONTACT: 121 return "contact"; 122 case CLINICAL: 123 return "clinical"; 124 case BEHAVIORAL: 125 return "behavioral"; 126 case RESEARCH: 127 return "research"; 128 case ADVANCEDIRECTIVE: 129 return "advance-directive"; 130 case SAFETY: 131 return "safety"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getSystem() { 140 return "http://terminology.hl7.org/CodeSystem/flag-category"; 141 } 142 143 public String getDefinition() { 144 switch (this) { 145 case DIET: 146 return "Flags related to the subject's dietary needs."; 147 case DRUG: 148 return "Flags related to the subject's medications."; 149 case LAB: 150 return "Flags related to performing laboratory tests and related processes (e.g. phlebotomy)."; 151 case ADMIN: 152 return "Flags related to administrative and financial processes."; 153 case CONTACT: 154 return "Flags related to coming into contact with the patient."; 155 case CLINICAL: 156 return "Flags related to the subject's clinical data."; 157 case BEHAVIORAL: 158 return "Flags related to behavior."; 159 case RESEARCH: 160 return "Flags related to research."; 161 case ADVANCEDIRECTIVE: 162 return "Flags related to subject's advance directives."; 163 case SAFETY: 164 return "Flags related to safety precautions."; 165 case NULL: 166 return null; 167 default: 168 return "?"; 169 } 170 } 171 172 public String getDisplay() { 173 switch (this) { 174 case DIET: 175 return "Diet"; 176 case DRUG: 177 return "Drug"; 178 case LAB: 179 return "Lab"; 180 case ADMIN: 181 return "Administrative"; 182 case CONTACT: 183 return "Subject Contact"; 184 case CLINICAL: 185 return "Clinical"; 186 case BEHAVIORAL: 187 return "Behavioral"; 188 case RESEARCH: 189 return "Research"; 190 case ADVANCEDIRECTIVE: 191 return "Advance Directive"; 192 case SAFETY: 193 return "Safety"; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 201}