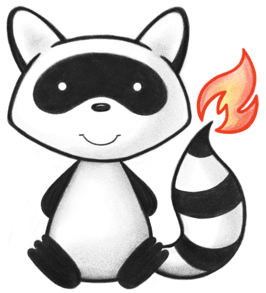
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum GoalStatus { 037 038 /** 039 * A goal is proposed for this patient. 040 */ 041 PROPOSED, 042 /** 043 * A goal is planned for this patient. 044 */ 045 PLANNED, 046 /** 047 * A proposed goal was accepted or acknowledged. 048 */ 049 ACCEPTED, 050 /** 051 * The goal is being sought actively. 052 */ 053 ACTIVE, 054 /** 055 * The goal remains a long term objective but is no longer being actively 056 * pursued for a temporary period of time. 057 */ 058 ONHOLD, 059 /** 060 * The goal is no longer being sought. 061 */ 062 COMPLETED, 063 /** 064 * The goal has been abandoned. 065 */ 066 CANCELLED, 067 /** 068 * The goal was entered in error and voided. 069 */ 070 ENTEREDINERROR, 071 /** 072 * A proposed goal was rejected. 073 */ 074 REJECTED, 075 /** 076 * added to help the parsers 077 */ 078 NULL; 079 080 public static GoalStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("proposed".equals(codeString)) 084 return PROPOSED; 085 if ("planned".equals(codeString)) 086 return PLANNED; 087 if ("accepted".equals(codeString)) 088 return ACCEPTED; 089 if ("active".equals(codeString)) 090 return ACTIVE; 091 if ("on-hold".equals(codeString)) 092 return ONHOLD; 093 if ("completed".equals(codeString)) 094 return COMPLETED; 095 if ("cancelled".equals(codeString)) 096 return CANCELLED; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if ("rejected".equals(codeString)) 100 return REJECTED; 101 throw new FHIRException("Unknown GoalStatus code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case PROPOSED: 107 return "proposed"; 108 case PLANNED: 109 return "planned"; 110 case ACCEPTED: 111 return "accepted"; 112 case ACTIVE: 113 return "active"; 114 case ONHOLD: 115 return "on-hold"; 116 case COMPLETED: 117 return "completed"; 118 case CANCELLED: 119 return "cancelled"; 120 case ENTEREDINERROR: 121 return "entered-in-error"; 122 case REJECTED: 123 return "rejected"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getSystem() { 132 return "http://hl7.org/fhir/goal-status"; 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case PROPOSED: 138 return "A goal is proposed for this patient."; 139 case PLANNED: 140 return "A goal is planned for this patient."; 141 case ACCEPTED: 142 return "A proposed goal was accepted or acknowledged."; 143 case ACTIVE: 144 return "The goal is being sought actively."; 145 case ONHOLD: 146 return "The goal remains a long term objective but is no longer being actively pursued for a temporary period of time."; 147 case COMPLETED: 148 return "The goal is no longer being sought."; 149 case CANCELLED: 150 return "The goal has been abandoned."; 151 case ENTEREDINERROR: 152 return "The goal was entered in error and voided."; 153 case REJECTED: 154 return "A proposed goal was rejected."; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162 public String getDisplay() { 163 switch (this) { 164 case PROPOSED: 165 return "Proposed"; 166 case PLANNED: 167 return "Planned"; 168 case ACCEPTED: 169 return "Accepted"; 170 case ACTIVE: 171 return "Active"; 172 case ONHOLD: 173 return "On Hold"; 174 case COMPLETED: 175 return "Completed"; 176 case CANCELLED: 177 return "Cancelled"; 178 case ENTEREDINERROR: 179 return "Entered in Error"; 180 case REJECTED: 181 return "Rejected"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 189}