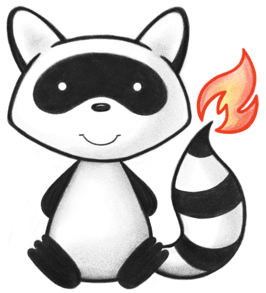
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum GuideParameterCode { 037 038 /** 039 * If the value of this string 0..* parameter is one of the metadata fields then 040 * all conformance resources will have any specified [Resource].[field] 041 * overwritten with the ImplementationGuide.[field], where field is one of: 042 * version, date, status, publisher, contact, copyright, experimental, 043 * jurisdiction, useContext. 044 */ 045 APPLY, 046 /** 047 * The value of this string 0..* parameter is a subfolder of the build context's 048 * location that is to be scanned to load resources. Scope is (if present) a 049 * particular resource type. 050 */ 051 PATHRESOURCE, 052 /** 053 * The value of this string 0..1 parameter is a subfolder of the build context's 054 * location that contains files that are part of the html content processed by 055 * the builder. 056 */ 057 PATHPAGES, 058 /** 059 * The value of this string 0..1 parameter is a subfolder of the build context's 060 * location that is used as the terminology cache. If this is not present, the 061 * terminology cache is on the local system, not under version control. 062 */ 063 PATHTXCACHE, 064 /** 065 * The value of this string 0..* parameter is a parameter (name=value) when 066 * expanding value sets for this implementation guide. This is particularly used 067 * to specify the versions of published terminologies such as SNOMED CT. 068 */ 069 EXPANSIONPARAMETER, 070 /** 071 * The value of this string 0..1 parameter is either "warning" or "error" 072 * (default = "error"). If the value is "warning" then IG build tools allow the 073 * IG to be considered successfully build even when there is no internal broken 074 * links. 075 */ 076 RULEBROKENLINKS, 077 /** 078 * The value of this boolean 0..1 parameter specifies whether the IG publisher 079 * creates examples in XML format. If not present, the Publication Tool decides 080 * whether to generate XML. 081 */ 082 GENERATEXML, 083 /** 084 * The value of this boolean 0..1 parameter specifies whether the IG publisher 085 * creates examples in JSON format. If not present, the Publication Tool decides 086 * whether to generate JSON. 087 */ 088 GENERATEJSON, 089 /** 090 * The value of this boolean 0..1 parameter specifies whether the IG publisher 091 * creates examples in Turtle format. If not present, the Publication Tool 092 * decides whether to generate Turtle. 093 */ 094 GENERATETURTLE, 095 /** 096 * The value of this string singleton parameter is the name of the file to use 097 * as the builder template for each generated page (see templating). 098 */ 099 HTMLTEMPLATE, 100 /** 101 * added to help the parsers 102 */ 103 NULL; 104 105 public static GuideParameterCode fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("apply".equals(codeString)) 109 return APPLY; 110 if ("path-resource".equals(codeString)) 111 return PATHRESOURCE; 112 if ("path-pages".equals(codeString)) 113 return PATHPAGES; 114 if ("path-tx-cache".equals(codeString)) 115 return PATHTXCACHE; 116 if ("expansion-parameter".equals(codeString)) 117 return EXPANSIONPARAMETER; 118 if ("rule-broken-links".equals(codeString)) 119 return RULEBROKENLINKS; 120 if ("generate-xml".equals(codeString)) 121 return GENERATEXML; 122 if ("generate-json".equals(codeString)) 123 return GENERATEJSON; 124 if ("generate-turtle".equals(codeString)) 125 return GENERATETURTLE; 126 if ("html-template".equals(codeString)) 127 return HTMLTEMPLATE; 128 throw new FHIRException("Unknown GuideParameterCode code '" + codeString + "'"); 129 } 130 131 public String toCode() { 132 switch (this) { 133 case APPLY: 134 return "apply"; 135 case PATHRESOURCE: 136 return "path-resource"; 137 case PATHPAGES: 138 return "path-pages"; 139 case PATHTXCACHE: 140 return "path-tx-cache"; 141 case EXPANSIONPARAMETER: 142 return "expansion-parameter"; 143 case RULEBROKENLINKS: 144 return "rule-broken-links"; 145 case GENERATEXML: 146 return "generate-xml"; 147 case GENERATEJSON: 148 return "generate-json"; 149 case GENERATETURTLE: 150 return "generate-turtle"; 151 case HTMLTEMPLATE: 152 return "html-template"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getSystem() { 161 return "http://hl7.org/fhir/guide-parameter-code"; 162 } 163 164 public String getDefinition() { 165 switch (this) { 166 case APPLY: 167 return "If the value of this string 0..* parameter is one of the metadata fields then all conformance resources will have any specified [Resource].[field] overwritten with the ImplementationGuide.[field], where field is one of: version, date, status, publisher, contact, copyright, experimental, jurisdiction, useContext."; 168 case PATHRESOURCE: 169 return "The value of this string 0..* parameter is a subfolder of the build context's location that is to be scanned to load resources. Scope is (if present) a particular resource type."; 170 case PATHPAGES: 171 return "The value of this string 0..1 parameter is a subfolder of the build context's location that contains files that are part of the html content processed by the builder."; 172 case PATHTXCACHE: 173 return "The value of this string 0..1 parameter is a subfolder of the build context's location that is used as the terminology cache. If this is not present, the terminology cache is on the local system, not under version control."; 174 case EXPANSIONPARAMETER: 175 return "The value of this string 0..* parameter is a parameter (name=value) when expanding value sets for this implementation guide. This is particularly used to specify the versions of published terminologies such as SNOMED CT."; 176 case RULEBROKENLINKS: 177 return "The value of this string 0..1 parameter is either \"warning\" or \"error\" (default = \"error\"). If the value is \"warning\" then IG build tools allow the IG to be considered successfully build even when there is no internal broken links."; 178 case GENERATEXML: 179 return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in XML format. If not present, the Publication Tool decides whether to generate XML."; 180 case GENERATEJSON: 181 return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in JSON format. If not present, the Publication Tool decides whether to generate JSON."; 182 case GENERATETURTLE: 183 return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in Turtle format. If not present, the Publication Tool decides whether to generate Turtle."; 184 case HTMLTEMPLATE: 185 return "The value of this string singleton parameter is the name of the file to use as the builder template for each generated page (see templating)."; 186 case NULL: 187 return null; 188 default: 189 return "?"; 190 } 191 } 192 193 public String getDisplay() { 194 switch (this) { 195 case APPLY: 196 return "Apply Metadata Value"; 197 case PATHRESOURCE: 198 return "Resource Path"; 199 case PATHPAGES: 200 return "Pages Path"; 201 case PATHTXCACHE: 202 return "Terminology Cache Path"; 203 case EXPANSIONPARAMETER: 204 return "Expansion Profile"; 205 case RULEBROKENLINKS: 206 return "Broken Links Rule"; 207 case GENERATEXML: 208 return "Generate XML"; 209 case GENERATEJSON: 210 return "Generate JSON"; 211 case GENERATETURTLE: 212 return "Generate Turtle"; 213 case HTMLTEMPLATE: 214 return "HTML Template"; 215 case NULL: 216 return null; 217 default: 218 return "?"; 219 } 220 } 221 222}