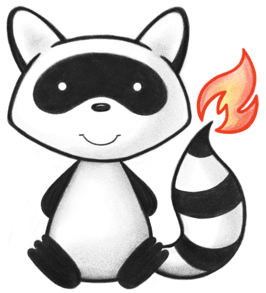
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Hl7WorkGroup { 037 038 /** 039 * Community Based Collaborative Care 040 * (http://www.hl7.org/Special/committees/cbcc/index.cfm). 041 */ 042 CBCC, 043 /** 044 * Clinical Decision Support 045 * (http://www.hl7.org/Special/committees/dss/index.cfm). 046 */ 047 CDS, 048 /** 049 * Clinical Quality Information 050 * (http://www.hl7.org/Special/committees/cqi/index.cfm). 051 */ 052 CQI, 053 /** 054 * Clinical Genomics 055 * (http://www.hl7.org/Special/committees/clingenomics/index.cfm). 056 */ 057 CG, 058 /** 059 * Health Care Devices 060 * (http://www.hl7.org/Special/committees/healthcaredevices/index.cfm). 061 */ 062 DEV, 063 /** 064 * Electronic Health Records 065 * (http://www.hl7.org/Special/committees/ehr/index.cfm). 066 */ 067 EHR, 068 /** 069 * FHIR Infrastructure (http://www.hl7.org/Special/committees/fiwg/index.cfm). 070 */ 071 FHIR, 072 /** 073 * Financial Management (http://www.hl7.org/Special/committees/fm/index.cfm). 074 */ 075 FM, 076 /** 077 * Health Standards Integration 078 * (http://www.hl7.org/Special/committees/hsi/index.cfm). 079 */ 080 HSI, 081 /** 082 * Imaging Integration 083 * (http://www.hl7.org/Special/committees/imagemgt/index.cfm). 084 */ 085 II, 086 /** 087 * Infrastructure And Messaging 088 * (http://www.hl7.org/Special/committees/inm/index.cfm). 089 */ 090 INM, 091 /** 092 * Implementable Technology Specifications 093 * (http://www.hl7.org/Special/committees/xml/index.cfm). 094 */ 095 ITS, 096 /** 097 * Modeling and Methodology 098 * (http://www.hl7.org/Special/committees/mnm/index.cfm). 099 */ 100 MNM, 101 /** 102 * Orders and Observations 103 * (http://www.hl7.org/Special/committees/orders/index.cfm). 104 */ 105 OO, 106 /** 107 * Patient Administration 108 * (http://www.hl7.org/Special/committees/pafm/index.cfm). 109 */ 110 PA, 111 /** 112 * Patient Care (http://www.hl7.org/Special/committees/patientcare/index.cfm). 113 */ 114 PC, 115 /** 116 * Public Health and Emergency Response 117 * (http://www.hl7.org/Special/committees/pher/index.cfm). 118 */ 119 PHER, 120 /** 121 * Pharmacy (http://www.hl7.org/Special/committees/medication/index.cfm). 122 */ 123 PHX, 124 /** 125 * Biomedical Research and Regulation 126 * (http://www.hl7.org/Special/committees/rcrim/index.cfm). 127 */ 128 BRR, 129 /** 130 * Structured Documents 131 * (http://www.hl7.org/Special/committees/structure/index.cfm). 132 */ 133 SD, 134 /** 135 * Security (http://www.hl7.org/Special/committees/secure/index.cfm). 136 */ 137 SEC, 138 /** 139 * US Realm Taskforce (http://www.hl7.org/Special/committees/usrealm/index.cfm). 140 */ 141 US, 142 /** 143 * Vocabulary (http://www.hl7.org/Special/committees/Vocab/index.cfm). 144 */ 145 VOCAB, 146 /** 147 * Application Implementation and Design 148 * (http://www.hl7.org/Special/committees/java/index.cfm). 149 */ 150 AID, 151 /** 152 * added to help the parsers 153 */ 154 NULL; 155 156 public static Hl7WorkGroup fromCode(String codeString) throws FHIRException { 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("cbcc".equals(codeString)) 160 return CBCC; 161 if ("cds".equals(codeString)) 162 return CDS; 163 if ("cqi".equals(codeString)) 164 return CQI; 165 if ("cg".equals(codeString)) 166 return CG; 167 if ("dev".equals(codeString)) 168 return DEV; 169 if ("ehr".equals(codeString)) 170 return EHR; 171 if ("fhir".equals(codeString)) 172 return FHIR; 173 if ("fm".equals(codeString)) 174 return FM; 175 if ("hsi".equals(codeString)) 176 return HSI; 177 if ("ii".equals(codeString)) 178 return II; 179 if ("inm".equals(codeString)) 180 return INM; 181 if ("its".equals(codeString)) 182 return ITS; 183 if ("mnm".equals(codeString)) 184 return MNM; 185 if ("oo".equals(codeString)) 186 return OO; 187 if ("pa".equals(codeString)) 188 return PA; 189 if ("pc".equals(codeString)) 190 return PC; 191 if ("pher".equals(codeString)) 192 return PHER; 193 if ("phx".equals(codeString)) 194 return PHX; 195 if ("brr".equals(codeString)) 196 return BRR; 197 if ("sd".equals(codeString)) 198 return SD; 199 if ("sec".equals(codeString)) 200 return SEC; 201 if ("us".equals(codeString)) 202 return US; 203 if ("vocab".equals(codeString)) 204 return VOCAB; 205 if ("aid".equals(codeString)) 206 return AID; 207 throw new FHIRException("Unknown Hl7WorkGroup code '" + codeString + "'"); 208 } 209 210 public String toCode() { 211 switch (this) { 212 case CBCC: 213 return "cbcc"; 214 case CDS: 215 return "cds"; 216 case CQI: 217 return "cqi"; 218 case CG: 219 return "cg"; 220 case DEV: 221 return "dev"; 222 case EHR: 223 return "ehr"; 224 case FHIR: 225 return "fhir"; 226 case FM: 227 return "fm"; 228 case HSI: 229 return "hsi"; 230 case II: 231 return "ii"; 232 case INM: 233 return "inm"; 234 case ITS: 235 return "its"; 236 case MNM: 237 return "mnm"; 238 case OO: 239 return "oo"; 240 case PA: 241 return "pa"; 242 case PC: 243 return "pc"; 244 case PHER: 245 return "pher"; 246 case PHX: 247 return "phx"; 248 case BRR: 249 return "brr"; 250 case SD: 251 return "sd"; 252 case SEC: 253 return "sec"; 254 case US: 255 return "us"; 256 case VOCAB: 257 return "vocab"; 258 case AID: 259 return "aid"; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getSystem() { 268 return "http://terminology.hl7.org/CodeSystem/hl7-work-group"; 269 } 270 271 public String getDefinition() { 272 switch (this) { 273 case CBCC: 274 return "Community Based Collaborative Care (http://www.hl7.org/Special/committees/cbcc/index.cfm)."; 275 case CDS: 276 return "Clinical Decision Support (http://www.hl7.org/Special/committees/dss/index.cfm)."; 277 case CQI: 278 return "Clinical Quality Information (http://www.hl7.org/Special/committees/cqi/index.cfm)."; 279 case CG: 280 return "Clinical Genomics (http://www.hl7.org/Special/committees/clingenomics/index.cfm)."; 281 case DEV: 282 return "Health Care Devices (http://www.hl7.org/Special/committees/healthcaredevices/index.cfm)."; 283 case EHR: 284 return "Electronic Health Records (http://www.hl7.org/Special/committees/ehr/index.cfm)."; 285 case FHIR: 286 return "FHIR Infrastructure (http://www.hl7.org/Special/committees/fiwg/index.cfm)."; 287 case FM: 288 return "Financial Management (http://www.hl7.org/Special/committees/fm/index.cfm)."; 289 case HSI: 290 return "Health Standards Integration (http://www.hl7.org/Special/committees/hsi/index.cfm)."; 291 case II: 292 return "Imaging Integration (http://www.hl7.org/Special/committees/imagemgt/index.cfm)."; 293 case INM: 294 return "Infrastructure And Messaging (http://www.hl7.org/Special/committees/inm/index.cfm)."; 295 case ITS: 296 return "Implementable Technology Specifications (http://www.hl7.org/Special/committees/xml/index.cfm)."; 297 case MNM: 298 return "Modeling and Methodology (http://www.hl7.org/Special/committees/mnm/index.cfm)."; 299 case OO: 300 return "Orders and Observations (http://www.hl7.org/Special/committees/orders/index.cfm)."; 301 case PA: 302 return "Patient Administration (http://www.hl7.org/Special/committees/pafm/index.cfm)."; 303 case PC: 304 return "Patient Care (http://www.hl7.org/Special/committees/patientcare/index.cfm)."; 305 case PHER: 306 return "Public Health and Emergency Response (http://www.hl7.org/Special/committees/pher/index.cfm)."; 307 case PHX: 308 return "Pharmacy (http://www.hl7.org/Special/committees/medication/index.cfm)."; 309 case BRR: 310 return "Biomedical Research and Regulation (http://www.hl7.org/Special/committees/rcrim/index.cfm)."; 311 case SD: 312 return "Structured Documents (http://www.hl7.org/Special/committees/structure/index.cfm)."; 313 case SEC: 314 return "Security (http://www.hl7.org/Special/committees/secure/index.cfm)."; 315 case US: 316 return "US Realm Taskforce (http://www.hl7.org/Special/committees/usrealm/index.cfm)."; 317 case VOCAB: 318 return "Vocabulary (http://www.hl7.org/Special/committees/Vocab/index.cfm)."; 319 case AID: 320 return "Application Implementation and Design (http://www.hl7.org/Special/committees/java/index.cfm)."; 321 case NULL: 322 return null; 323 default: 324 return "?"; 325 } 326 } 327 328 public String getDisplay() { 329 switch (this) { 330 case CBCC: 331 return "Community Based Collaborative Care"; 332 case CDS: 333 return "Clinical Decision Support"; 334 case CQI: 335 return "Clinical Quality Information"; 336 case CG: 337 return "Clinical Genomics"; 338 case DEV: 339 return "Health Care Devices"; 340 case EHR: 341 return "Electronic Health Records"; 342 case FHIR: 343 return "FHIR Infrastructure"; 344 case FM: 345 return "Financial Management"; 346 case HSI: 347 return "Health Standards Integration"; 348 case II: 349 return "Imaging Integration"; 350 case INM: 351 return "Infrastructure And Messaging"; 352 case ITS: 353 return "Implementable Technology Specifications"; 354 case MNM: 355 return "Modeling and Methodology"; 356 case OO: 357 return "Orders and Observations"; 358 case PA: 359 return "Patient Administration"; 360 case PC: 361 return "Patient Care"; 362 case PHER: 363 return "Public Health and Emergency Response"; 364 case PHX: 365 return "Pharmacy"; 366 case BRR: 367 return "Biomedical Research and Regulation"; 368 case SD: 369 return "Structured Documents"; 370 case SEC: 371 return "Security"; 372 case US: 373 return "US Realm Taskforce"; 374 case VOCAB: 375 return "Vocabulary"; 376 case AID: 377 return "Application Implementation and Design"; 378 case NULL: 379 return null; 380 default: 381 return "?"; 382 } 383 } 384 385}