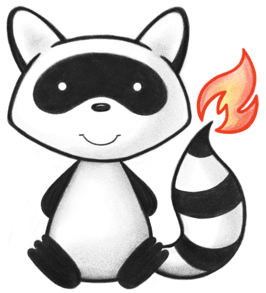
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ImagingstudyStatus { 037 038 /** 039 * The existence of the imaging study is registered, but there is nothing yet 040 * available. 041 */ 042 REGISTERED, 043 /** 044 * At least one instance has been associated with this imaging study. 045 */ 046 AVAILABLE, 047 /** 048 * The imaging study is unavailable because the imaging study was not started or 049 * not completed (also sometimes called "aborted"). 050 */ 051 CANCELLED, 052 /** 053 * The imaging study has been withdrawn following a previous final release. This 054 * electronic record should never have existed, though it is possible that 055 * real-world decisions were based on it. (If real-world activity has occurred, 056 * the status should be "cancelled" rather than "entered-in-error".). 057 */ 058 ENTEREDINERROR, 059 /** 060 * The system does not know which of the status values currently applies for 061 * this request. Note: This concept is not to be used for "other" - one of the 062 * listed statuses is presumed to apply, it's just not known which one. 063 */ 064 UNKNOWN, 065 /** 066 * added to help the parsers 067 */ 068 NULL; 069 070 public static ImagingstudyStatus fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("registered".equals(codeString)) 074 return REGISTERED; 075 if ("available".equals(codeString)) 076 return AVAILABLE; 077 if ("cancelled".equals(codeString)) 078 return CANCELLED; 079 if ("entered-in-error".equals(codeString)) 080 return ENTEREDINERROR; 081 if ("unknown".equals(codeString)) 082 return UNKNOWN; 083 throw new FHIRException("Unknown ImagingstudyStatus code '" + codeString + "'"); 084 } 085 086 public String toCode() { 087 switch (this) { 088 case REGISTERED: 089 return "registered"; 090 case AVAILABLE: 091 return "available"; 092 case CANCELLED: 093 return "cancelled"; 094 case ENTEREDINERROR: 095 return "entered-in-error"; 096 case UNKNOWN: 097 return "unknown"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getSystem() { 106 return "http://hl7.org/fhir/imagingstudy-status"; 107 } 108 109 public String getDefinition() { 110 switch (this) { 111 case REGISTERED: 112 return "The existence of the imaging study is registered, but there is nothing yet available."; 113 case AVAILABLE: 114 return "At least one instance has been associated with this imaging study."; 115 case CANCELLED: 116 return "The imaging study is unavailable because the imaging study was not started or not completed (also sometimes called \"aborted\")."; 117 case ENTEREDINERROR: 118 return "The imaging study has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 119 case UNKNOWN: 120 return "The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDisplay() { 129 switch (this) { 130 case REGISTERED: 131 return "Registered"; 132 case AVAILABLE: 133 return "Available"; 134 case CANCELLED: 135 return "Cancelled"; 136 case ENTEREDINERROR: 137 return "Entered in Error"; 138 case UNKNOWN: 139 return "Unknown"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147}