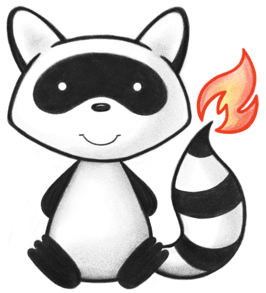
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ImmunizationOrigin { 037 038 /** 039 * The data for the immunization event originated with another provider. 040 */ 041 PROVIDER, 042 /** 043 * The data for the immunization event originated with a written record for the 044 * patient. 045 */ 046 RECORD, 047 /** 048 * The data for the immunization event originated from the recollection of the 049 * patient or parent/guardian of the patient. 050 */ 051 RECALL, 052 /** 053 * The data for the immunization event originated with a school record for the 054 * patient. 055 */ 056 SCHOOL, 057 /** 058 * The data for the immunization event originated with an immunization 059 * information system (IIS) or registry operating within the jurisdiction. 060 */ 061 JURISDICTION, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 067 public static ImmunizationOrigin fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("provider".equals(codeString)) 071 return PROVIDER; 072 if ("record".equals(codeString)) 073 return RECORD; 074 if ("recall".equals(codeString)) 075 return RECALL; 076 if ("school".equals(codeString)) 077 return SCHOOL; 078 if ("jurisdiction".equals(codeString)) 079 return JURISDICTION; 080 throw new FHIRException("Unknown ImmunizationOrigin code '" + codeString + "'"); 081 } 082 083 public String toCode() { 084 switch (this) { 085 case PROVIDER: 086 return "provider"; 087 case RECORD: 088 return "record"; 089 case RECALL: 090 return "recall"; 091 case SCHOOL: 092 return "school"; 093 case JURISDICTION: 094 return "jurisdiction"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 return "http://terminology.hl7.org/CodeSystem/immunization-origin"; 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case PROVIDER: 109 return "The data for the immunization event originated with another provider."; 110 case RECORD: 111 return "The data for the immunization event originated with a written record for the patient."; 112 case RECALL: 113 return "The data for the immunization event originated from the recollection of the patient or parent/guardian of the patient."; 114 case SCHOOL: 115 return "The data for the immunization event originated with a school record for the patient."; 116 case JURISDICTION: 117 return "The data for the immunization event originated with an immunization information system (IIS) or registry operating within the jurisdiction."; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getDisplay() { 126 switch (this) { 127 case PROVIDER: 128 return "Other Provider"; 129 case RECORD: 130 return "Written Record"; 131 case RECALL: 132 return "Parent/Guardian/Patient Recall"; 133 case SCHOOL: 134 return "School Record"; 135 case JURISDICTION: 136 return "Jurisdictional IIS"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144}