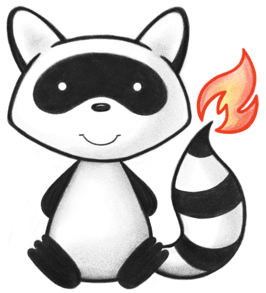
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ImmunizationRecommendationStatus { 037 038 /** 039 * The patient is due for their next vaccination. 040 */ 041 DUE, 042 /** 043 * The patient is considered overdue for their next vaccination. 044 */ 045 OVERDUE, 046 /** 047 * The patient is immune to the target disease and further immunization against 048 * the disease is not likely to provide benefit. 049 */ 050 IMMUNE, 051 /** 052 * The patient is contraindicated for futher doses. 053 */ 054 CONTRAINDICATED, 055 /** 056 * The patient is fully protected and no further doses are recommended. 057 */ 058 COMPLETE, 059 /** 060 * added to help the parsers 061 */ 062 NULL; 063 064 public static ImmunizationRecommendationStatus fromCode(String codeString) throws FHIRException { 065 if (codeString == null || "".equals(codeString)) 066 return null; 067 if ("due".equals(codeString)) 068 return DUE; 069 if ("overdue".equals(codeString)) 070 return OVERDUE; 071 if ("immune".equals(codeString)) 072 return IMMUNE; 073 if ("contraindicated".equals(codeString)) 074 return CONTRAINDICATED; 075 if ("complete".equals(codeString)) 076 return COMPLETE; 077 throw new FHIRException("Unknown ImmunizationRecommendationStatus code '" + codeString + "'"); 078 } 079 080 public String toCode() { 081 switch (this) { 082 case DUE: 083 return "due"; 084 case OVERDUE: 085 return "overdue"; 086 case IMMUNE: 087 return "immune"; 088 case CONTRAINDICATED: 089 return "contraindicated"; 090 case COMPLETE: 091 return "complete"; 092 case NULL: 093 return null; 094 default: 095 return "?"; 096 } 097 } 098 099 public String getSystem() { 100 return "http://terminology.hl7.org/CodeSystem/immunization-recommendation-status"; 101 } 102 103 public String getDefinition() { 104 switch (this) { 105 case DUE: 106 return "The patient is due for their next vaccination."; 107 case OVERDUE: 108 return "The patient is considered overdue for their next vaccination."; 109 case IMMUNE: 110 return "The patient is immune to the target disease and further immunization against the disease is not likely to provide benefit."; 111 case CONTRAINDICATED: 112 return "The patient is contraindicated for futher doses."; 113 case COMPLETE: 114 return "The patient is fully protected and no further doses are recommended."; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDisplay() { 123 switch (this) { 124 case DUE: 125 return "Due"; 126 case OVERDUE: 127 return "Overdue"; 128 case IMMUNE: 129 return "Immune"; 130 case CONTRAINDICATED: 131 return "Contraindicated"; 132 case COMPLETE: 133 return "Complete"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141}