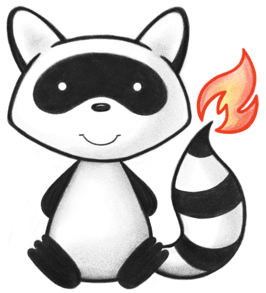
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Iso21089Lifecycle { 037 038 /** 039 * Occurs when an agent causes the system to obtain and open a record entry for 040 * inspection or review. 041 */ 042 ACCESS, 043 /** 044 * Occurs when an agent causes the system to tag or otherwise indicate special 045 * access management and suspension of record entry deletion/destruction, if 046 * deemed relevant to a lawsuit or which are reasonably anticipated to be 047 * relevant or to fulfill organizational policy under the legal doctrine of 048 * ?duty to preserve?. 049 */ 050 HOLD, 051 /** 052 * Occurs when an agent makes any change to record entry content currently 053 * residing in storage considered permanent (persistent). 054 */ 055 AMEND, 056 /** 057 * Occurs when an agent causes the system to create and move archive artifacts 058 * containing record entry content, typically to long-term offline storage. 059 */ 060 ARCHIVE, 061 /** 062 * Occurs when an agent causes the system to capture the agent?s digital 063 * signature (or equivalent indication) during formal validation of record entry 064 * content. 065 */ 066 ATTEST, 067 /** 068 * Occurs when an agent causes the system to decode record entry content from a 069 * cipher. 070 */ 071 DECRYPT, 072 /** 073 * Occurs when an agent causes the system to scrub record entry content to 074 * reduce the association between a set of identifying data and the data subject 075 * in a way that might or might not be reversible. 076 */ 077 DEIDENTIFY, 078 /** 079 * Occurs when an agent causes the system to tag record entry(ies) as obsolete, 080 * erroneous or untrustworthy, to warn against its future use. 081 */ 082 DEPRECATE, 083 /** 084 * Occurs when an agent causes the system to permanently erase record entry 085 * content from the system. 086 */ 087 DESTROY, 088 /** 089 * Occurs when an agent causes the system to release, transfer, provision access 090 * to, or otherwise divulge record entry content. 091 */ 092 DISCLOSE, 093 /** 094 * Occurs when an agent causes the system to encode record entry content in a 095 * cipher. 096 */ 097 ENCRYPT, 098 /** 099 * Occurs when an agent causes the system to selectively pull out a subset of 100 * record entry content, based on explicit criteria. 101 */ 102 EXTRACT, 103 /** 104 * Occurs when an agent causes the system to connect related record entries. 105 */ 106 LINK, 107 /** 108 * Occurs when an agent causes the system to combine or join content from two or 109 * more record entries, resulting in a single logical record entry. 110 */ 111 MERGE, 112 /** 113 * Occurs when an agent causes the system to: a) initiate capture of potential 114 * record content, and b) incorporate that content into the storage considered a 115 * permanent part of the health record. 116 */ 117 ORIGINATE, 118 /** 119 * Occurs when an agent causes the system to remove record entry content to 120 * reduce the association between a set of identifying data and the data subject 121 * in a way that may be reversible. 122 */ 123 PSEUDONYMIZE, 124 /** 125 * Occurs when an agent causes the system to recreate or restore full status to 126 * record entries previously deleted or deprecated. 127 */ 128 REACTIVATE, 129 /** 130 * Occurs when an agent causes the system to a) initiate capture of data content 131 * from elsewhere, and b) incorporate that content into the storage considered a 132 * permanent part of the health record. 133 */ 134 RECEIVE, 135 /** 136 * Occurs when an agent causes the system to restore information to data that 137 * allows identification of information source and/or information subject. 138 */ 139 REIDENTIFY, 140 /** 141 * Occurs when an agent causes the system to remove a tag or other cues for 142 * special access management had required to fulfill organizational policy under 143 * the legal doctrine of ?duty to preserve?. 144 */ 145 UNHOLD, 146 /** 147 * Occurs when an agent causes the system to produce and deliver record entry 148 * content in a particular form and manner. 149 */ 150 REPORT, 151 /** 152 * Occurs when an agent causes the system to recreate record entries and their 153 * content from a previous created archive artefact. 154 */ 155 RESTORE, 156 /** 157 * Occurs when an agent causes the system to change the form, language or code 158 * system used to represent record entry content. 159 */ 160 TRANSFORM, 161 /** 162 * Occurs when an agent causes the system to send record entry content from one 163 * (EHR/PHR/other) system to another. 164 */ 165 TRANSMIT, 166 /** 167 * Occurs when an agent causes the system to disconnect two or more record 168 * entries previously connected, rendering them separate (disconnected) again. 169 */ 170 UNLINK, 171 /** 172 * Occurs when an agent causes the system to reverse a previous record entry 173 * merge operation, rendering them separate again. 174 */ 175 UNMERGE, 176 /** 177 * Occurs when an agent causes the system to confirm compliance of data or data 178 * objects with regulations, requirements, specifications, or other imposed 179 * conditions based on organizational policy. 180 */ 181 VERIFY, 182 /** 183 * added to help the parsers 184 */ 185 NULL; 186 187 public static Iso21089Lifecycle fromCode(String codeString) throws FHIRException { 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("access".equals(codeString)) 191 return ACCESS; 192 if ("hold".equals(codeString)) 193 return HOLD; 194 if ("amend".equals(codeString)) 195 return AMEND; 196 if ("archive".equals(codeString)) 197 return ARCHIVE; 198 if ("attest".equals(codeString)) 199 return ATTEST; 200 if ("decrypt".equals(codeString)) 201 return DECRYPT; 202 if ("deidentify".equals(codeString)) 203 return DEIDENTIFY; 204 if ("deprecate".equals(codeString)) 205 return DEPRECATE; 206 if ("destroy".equals(codeString)) 207 return DESTROY; 208 if ("disclose".equals(codeString)) 209 return DISCLOSE; 210 if ("encrypt".equals(codeString)) 211 return ENCRYPT; 212 if ("extract".equals(codeString)) 213 return EXTRACT; 214 if ("link".equals(codeString)) 215 return LINK; 216 if ("merge".equals(codeString)) 217 return MERGE; 218 if ("originate".equals(codeString)) 219 return ORIGINATE; 220 if ("pseudonymize".equals(codeString)) 221 return PSEUDONYMIZE; 222 if ("reactivate".equals(codeString)) 223 return REACTIVATE; 224 if ("receive".equals(codeString)) 225 return RECEIVE; 226 if ("reidentify".equals(codeString)) 227 return REIDENTIFY; 228 if ("unhold".equals(codeString)) 229 return UNHOLD; 230 if ("report".equals(codeString)) 231 return REPORT; 232 if ("restore".equals(codeString)) 233 return RESTORE; 234 if ("transform".equals(codeString)) 235 return TRANSFORM; 236 if ("transmit".equals(codeString)) 237 return TRANSMIT; 238 if ("unlink".equals(codeString)) 239 return UNLINK; 240 if ("unmerge".equals(codeString)) 241 return UNMERGE; 242 if ("verify".equals(codeString)) 243 return VERIFY; 244 throw new FHIRException("Unknown Iso21089Lifecycle code '" + codeString + "'"); 245 } 246 247 public String toCode() { 248 switch (this) { 249 case ACCESS: 250 return "access"; 251 case HOLD: 252 return "hold"; 253 case AMEND: 254 return "amend"; 255 case ARCHIVE: 256 return "archive"; 257 case ATTEST: 258 return "attest"; 259 case DECRYPT: 260 return "decrypt"; 261 case DEIDENTIFY: 262 return "deidentify"; 263 case DEPRECATE: 264 return "deprecate"; 265 case DESTROY: 266 return "destroy"; 267 case DISCLOSE: 268 return "disclose"; 269 case ENCRYPT: 270 return "encrypt"; 271 case EXTRACT: 272 return "extract"; 273 case LINK: 274 return "link"; 275 case MERGE: 276 return "merge"; 277 case ORIGINATE: 278 return "originate"; 279 case PSEUDONYMIZE: 280 return "pseudonymize"; 281 case REACTIVATE: 282 return "reactivate"; 283 case RECEIVE: 284 return "receive"; 285 case REIDENTIFY: 286 return "reidentify"; 287 case UNHOLD: 288 return "unhold"; 289 case REPORT: 290 return "report"; 291 case RESTORE: 292 return "restore"; 293 case TRANSFORM: 294 return "transform"; 295 case TRANSMIT: 296 return "transmit"; 297 case UNLINK: 298 return "unlink"; 299 case UNMERGE: 300 return "unmerge"; 301 case VERIFY: 302 return "verify"; 303 case NULL: 304 return null; 305 default: 306 return "?"; 307 } 308 } 309 310 public String getSystem() { 311 return "http://terminology.hl7.org/CodeSystem/iso-21089-lifecycle"; 312 } 313 314 public String getDefinition() { 315 switch (this) { 316 case ACCESS: 317 return "Occurs when an agent causes the system to obtain and open a record entry for inspection or review."; 318 case HOLD: 319 return "Occurs when an agent causes the system to tag or otherwise indicate special access management and suspension of record entry deletion/destruction, if deemed relevant to a lawsuit or which are reasonably anticipated to be relevant or to fulfill organizational policy under the legal doctrine of ?duty to preserve?."; 320 case AMEND: 321 return "Occurs when an agent makes any change to record entry content currently residing in storage considered permanent (persistent)."; 322 case ARCHIVE: 323 return "Occurs when an agent causes the system to create and move archive artifacts containing record entry content, typically to long-term offline storage."; 324 case ATTEST: 325 return "Occurs when an agent causes the system to capture the agent?s digital signature (or equivalent indication) during formal validation of record entry content."; 326 case DECRYPT: 327 return "Occurs when an agent causes the system to decode record entry content from a cipher."; 328 case DEIDENTIFY: 329 return "Occurs when an agent causes the system to scrub record entry content to reduce the association between a set of identifying data and the data subject in a way that might or might not be reversible."; 330 case DEPRECATE: 331 return "Occurs when an agent causes the system to tag record entry(ies) as obsolete, erroneous or untrustworthy, to warn against its future use."; 332 case DESTROY: 333 return "Occurs when an agent causes the system to permanently erase record entry content from the system."; 334 case DISCLOSE: 335 return "Occurs when an agent causes the system to release, transfer, provision access to, or otherwise divulge record entry content."; 336 case ENCRYPT: 337 return "Occurs when an agent causes the system to encode record entry content in a cipher."; 338 case EXTRACT: 339 return "Occurs when an agent causes the system to selectively pull out a subset of record entry content, based on explicit criteria."; 340 case LINK: 341 return "Occurs when an agent causes the system to connect related record entries."; 342 case MERGE: 343 return "Occurs when an agent causes the system to combine or join content from two or more record entries, resulting in a single logical record entry."; 344 case ORIGINATE: 345 return "Occurs when an agent causes the system to: a) initiate capture of potential record content, and b) incorporate that content into the storage considered a permanent part of the health record."; 346 case PSEUDONYMIZE: 347 return "Occurs when an agent causes the system to remove record entry content to reduce the association between a set of identifying data and the data subject in a way that may be reversible."; 348 case REACTIVATE: 349 return "Occurs when an agent causes the system to recreate or restore full status to record entries previously deleted or deprecated."; 350 case RECEIVE: 351 return "Occurs when an agent causes the system to a) initiate capture of data content from elsewhere, and b) incorporate that content into the storage considered a permanent part of the health record."; 352 case REIDENTIFY: 353 return "Occurs when an agent causes the system to restore information to data that allows identification of information source and/or information subject."; 354 case UNHOLD: 355 return "Occurs when an agent causes the system to remove a tag or other cues for special access management had required to fulfill organizational policy under the legal doctrine of ?duty to preserve?."; 356 case REPORT: 357 return "Occurs when an agent causes the system to produce and deliver record entry content in a particular form and manner."; 358 case RESTORE: 359 return "Occurs when an agent causes the system to recreate record entries and their content from a previous created archive artefact."; 360 case TRANSFORM: 361 return "Occurs when an agent causes the system to change the form, language or code system used to represent record entry content."; 362 case TRANSMIT: 363 return "Occurs when an agent causes the system to send record entry content from one (EHR/PHR/other) system to another."; 364 case UNLINK: 365 return "Occurs when an agent causes the system to disconnect two or more record entries previously connected, rendering them separate (disconnected) again."; 366 case UNMERGE: 367 return "Occurs when an agent causes the system to reverse a previous record entry merge operation, rendering them separate again."; 368 case VERIFY: 369 return "Occurs when an agent causes the system to confirm compliance of data or data objects with regulations, requirements, specifications, or other imposed conditions based on organizational policy."; 370 case NULL: 371 return null; 372 default: 373 return "?"; 374 } 375 } 376 377 public String getDisplay() { 378 switch (this) { 379 case ACCESS: 380 return "Access/View Record Lifecycle Event"; 381 case HOLD: 382 return "Add Legal Hold Record Lifecycle Event"; 383 case AMEND: 384 return "Amend (Update) Record Lifecycle Event"; 385 case ARCHIVE: 386 return "Archive Record Lifecycle Event"; 387 case ATTEST: 388 return "Attest Record Lifecycle Event"; 389 case DECRYPT: 390 return "Decrypt Record Lifecycle Event"; 391 case DEIDENTIFY: 392 return "De-Identify (Anononymize) Record Lifecycle Event"; 393 case DEPRECATE: 394 return "Deprecate Record Lifecycle Event"; 395 case DESTROY: 396 return "Destroy/Delete Record Lifecycle Event"; 397 case DISCLOSE: 398 return "Disclose Record Lifecycle Event"; 399 case ENCRYPT: 400 return "Encrypt Record Lifecycle Event"; 401 case EXTRACT: 402 return "Extract Record Lifecycle Event"; 403 case LINK: 404 return "Link Record Lifecycle Event"; 405 case MERGE: 406 return "Merge Record Lifecycle Event"; 407 case ORIGINATE: 408 return "Originate/Retain Record Lifecycle Event"; 409 case PSEUDONYMIZE: 410 return "Pseudonymize Record Lifecycle Event"; 411 case REACTIVATE: 412 return "Re-activate Record Lifecycle Event"; 413 case RECEIVE: 414 return "Receive/Retain Record Lifecycle Event"; 415 case REIDENTIFY: 416 return "Re-identify Record Lifecycle Event"; 417 case UNHOLD: 418 return "Remove Legal Hold Record Lifecycle Event"; 419 case REPORT: 420 return "Report (Output) Record Lifecycle Event"; 421 case RESTORE: 422 return "Restore Record Lifecycle Event"; 423 case TRANSFORM: 424 return "Transform/Translate Record Lifecycle Event"; 425 case TRANSMIT: 426 return "Transmit Record Lifecycle Event"; 427 case UNLINK: 428 return "Unlink Record Lifecycle Event"; 429 case UNMERGE: 430 return "Unmerge Record Lifecycle Event"; 431 case VERIFY: 432 return "Verify Record Lifecycle Event"; 433 case NULL: 434 return null; 435 default: 436 return "?"; 437 } 438 } 439 440}