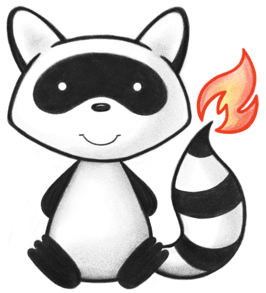
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum IssueType { 037 038 /** 039 * Content invalid against the specification or a profile. 040 */ 041 INVALID, 042 /** 043 * A structural issue in the content such as wrong namespace, unable to parse 044 * the content completely, invalid syntax, etc. 045 */ 046 STRUCTURE, 047 /** 048 * A required element is missing. 049 */ 050 REQUIRED, 051 /** 052 * An element or header value is invalid. 053 */ 054 VALUE, 055 /** 056 * A content validation rule failed - e.g. a schematron rule. 057 */ 058 INVARIANT, 059 /** 060 * An authentication/authorization/permissions issue of some kind. 061 */ 062 SECURITY, 063 /** 064 * The client needs to initiate an authentication process. 065 */ 066 LOGIN, 067 /** 068 * The user or system was not able to be authenticated (either there is no 069 * process, or the proferred token is unacceptable). 070 */ 071 UNKNOWN, 072 /** 073 * User session expired; a login may be required. 074 */ 075 EXPIRED, 076 /** 077 * The user does not have the rights to perform this action. 078 */ 079 FORBIDDEN, 080 /** 081 * Some information was not or might not have been returned due to business 082 * rules, consent or privacy rules, or access permission constraints. This 083 * information may be accessible through alternate processes. 084 */ 085 SUPPRESSED, 086 /** 087 * Processing issues. These are expected to be final e.g. there is no point 088 * resubmitting the same content unchanged. 089 */ 090 PROCESSING, 091 /** 092 * The interaction, operation, resource or profile is not supported. 093 */ 094 NOTSUPPORTED, 095 /** 096 * An attempt was made to create a duplicate record. 097 */ 098 DUPLICATE, 099 /** 100 * Multiple matching records were found when the operation required only one 101 * match. 102 */ 103 MULTIPLEMATCHES, 104 /** 105 * The reference provided was not found. In a pure RESTful environment, this 106 * would be an HTTP 404 error, but this code may be used where the content is 107 * not found further into the application architecture. 108 */ 109 NOTFOUND, 110 /** 111 * The reference pointed to content (usually a resource) that has been deleted. 112 */ 113 DELETED, 114 /** 115 * Provided content is too long (typically, this is a denial of service 116 * protection type of error). 117 */ 118 TOOLONG, 119 /** 120 * The code or system could not be understood, or it was not valid in the 121 * context of a particular ValueSet.code. 122 */ 123 CODEINVALID, 124 /** 125 * An extension was found that was not acceptable, could not be resolved, or a 126 * modifierExtension was not recognized. 127 */ 128 EXTENSION, 129 /** 130 * The operation was stopped to protect server resources; e.g. a request for a 131 * value set expansion on all of SNOMED CT. 132 */ 133 TOOCOSTLY, 134 /** 135 * The content/operation failed to pass some business rule and so could not 136 * proceed. 137 */ 138 BUSINESSRULE, 139 /** 140 * Content could not be accepted because of an edit conflict (i.e. version aware 141 * updates). (In a pure RESTful environment, this would be an HTTP 409 error, 142 * but this code may be used where the conflict is discovered further into the 143 * application architecture.). 144 */ 145 CONFLICT, 146 /** 147 * Transient processing issues. The system receiving the message may be able to 148 * resubmit the same content once an underlying issue is resolved. 149 */ 150 TRANSIENT, 151 /** 152 * A resource/record locking failure (usually in an underlying database). 153 */ 154 LOCKERROR, 155 /** 156 * The persistent store is unavailable; e.g. the database is down for 157 * maintenance or similar action, and the interaction or operation cannot be 158 * processed. 159 */ 160 NOSTORE, 161 /** 162 * y. 163 */ 164 EXCEPTION, 165 /** 166 * An internal timeout has occurred. 167 */ 168 TIMEOUT, 169 /** 170 * Not all data sources typically accessed could be reached or responded in 171 * time, so the returned information might not be complete (applies to search 172 * interactions and some operations). 173 */ 174 INCOMPLETE, 175 /** 176 * The system is not prepared to handle this request due to load management. 177 */ 178 THROTTLED, 179 /** 180 * A message unrelated to the processing success of the completed operation 181 * (examples of the latter include things like reminders of password expiry, 182 * system maintenance times, etc.). 183 */ 184 INFORMATIONAL, 185 /** 186 * added to help the parsers 187 */ 188 NULL; 189 190 public static IssueType fromCode(String codeString) throws FHIRException { 191 if (codeString == null || "".equals(codeString)) 192 return null; 193 if ("invalid".equals(codeString)) 194 return INVALID; 195 if ("structure".equals(codeString)) 196 return STRUCTURE; 197 if ("required".equals(codeString)) 198 return REQUIRED; 199 if ("value".equals(codeString)) 200 return VALUE; 201 if ("invariant".equals(codeString)) 202 return INVARIANT; 203 if ("security".equals(codeString)) 204 return SECURITY; 205 if ("login".equals(codeString)) 206 return LOGIN; 207 if ("unknown".equals(codeString)) 208 return UNKNOWN; 209 if ("expired".equals(codeString)) 210 return EXPIRED; 211 if ("forbidden".equals(codeString)) 212 return FORBIDDEN; 213 if ("suppressed".equals(codeString)) 214 return SUPPRESSED; 215 if ("processing".equals(codeString)) 216 return PROCESSING; 217 if ("not-supported".equals(codeString)) 218 return NOTSUPPORTED; 219 if ("duplicate".equals(codeString)) 220 return DUPLICATE; 221 if ("multiple-matches".equals(codeString)) 222 return MULTIPLEMATCHES; 223 if ("not-found".equals(codeString)) 224 return NOTFOUND; 225 if ("deleted".equals(codeString)) 226 return DELETED; 227 if ("too-long".equals(codeString)) 228 return TOOLONG; 229 if ("code-invalid".equals(codeString)) 230 return CODEINVALID; 231 if ("extension".equals(codeString)) 232 return EXTENSION; 233 if ("too-costly".equals(codeString)) 234 return TOOCOSTLY; 235 if ("business-rule".equals(codeString)) 236 return BUSINESSRULE; 237 if ("conflict".equals(codeString)) 238 return CONFLICT; 239 if ("transient".equals(codeString)) 240 return TRANSIENT; 241 if ("lock-error".equals(codeString)) 242 return LOCKERROR; 243 if ("no-store".equals(codeString)) 244 return NOSTORE; 245 if ("exception".equals(codeString)) 246 return EXCEPTION; 247 if ("timeout".equals(codeString)) 248 return TIMEOUT; 249 if ("incomplete".equals(codeString)) 250 return INCOMPLETE; 251 if ("throttled".equals(codeString)) 252 return THROTTLED; 253 if ("informational".equals(codeString)) 254 return INFORMATIONAL; 255 throw new FHIRException("Unknown IssueType code '" + codeString + "'"); 256 } 257 258 public String toCode() { 259 switch (this) { 260 case INVALID: 261 return "invalid"; 262 case STRUCTURE: 263 return "structure"; 264 case REQUIRED: 265 return "required"; 266 case VALUE: 267 return "value"; 268 case INVARIANT: 269 return "invariant"; 270 case SECURITY: 271 return "security"; 272 case LOGIN: 273 return "login"; 274 case UNKNOWN: 275 return "unknown"; 276 case EXPIRED: 277 return "expired"; 278 case FORBIDDEN: 279 return "forbidden"; 280 case SUPPRESSED: 281 return "suppressed"; 282 case PROCESSING: 283 return "processing"; 284 case NOTSUPPORTED: 285 return "not-supported"; 286 case DUPLICATE: 287 return "duplicate"; 288 case MULTIPLEMATCHES: 289 return "multiple-matches"; 290 case NOTFOUND: 291 return "not-found"; 292 case DELETED: 293 return "deleted"; 294 case TOOLONG: 295 return "too-long"; 296 case CODEINVALID: 297 return "code-invalid"; 298 case EXTENSION: 299 return "extension"; 300 case TOOCOSTLY: 301 return "too-costly"; 302 case BUSINESSRULE: 303 return "business-rule"; 304 case CONFLICT: 305 return "conflict"; 306 case TRANSIENT: 307 return "transient"; 308 case LOCKERROR: 309 return "lock-error"; 310 case NOSTORE: 311 return "no-store"; 312 case EXCEPTION: 313 return "exception"; 314 case TIMEOUT: 315 return "timeout"; 316 case INCOMPLETE: 317 return "incomplete"; 318 case THROTTLED: 319 return "throttled"; 320 case INFORMATIONAL: 321 return "informational"; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 329 public String getSystem() { 330 return "http://hl7.org/fhir/issue-type"; 331 } 332 333 public String getDefinition() { 334 switch (this) { 335 case INVALID: 336 return "Content invalid against the specification or a profile."; 337 case STRUCTURE: 338 return "A structural issue in the content such as wrong namespace, unable to parse the content completely, invalid syntax, etc."; 339 case REQUIRED: 340 return "A required element is missing."; 341 case VALUE: 342 return "An element or header value is invalid."; 343 case INVARIANT: 344 return "A content validation rule failed - e.g. a schematron rule."; 345 case SECURITY: 346 return "An authentication/authorization/permissions issue of some kind."; 347 case LOGIN: 348 return "The client needs to initiate an authentication process."; 349 case UNKNOWN: 350 return "The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable)."; 351 case EXPIRED: 352 return "User session expired; a login may be required."; 353 case FORBIDDEN: 354 return "The user does not have the rights to perform this action."; 355 case SUPPRESSED: 356 return "Some information was not or might not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes."; 357 case PROCESSING: 358 return "Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged."; 359 case NOTSUPPORTED: 360 return "The interaction, operation, resource or profile is not supported."; 361 case DUPLICATE: 362 return "An attempt was made to create a duplicate record."; 363 case MULTIPLEMATCHES: 364 return "Multiple matching records were found when the operation required only one match."; 365 case NOTFOUND: 366 return "The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture."; 367 case DELETED: 368 return "The reference pointed to content (usually a resource) that has been deleted."; 369 case TOOLONG: 370 return "Provided content is too long (typically, this is a denial of service protection type of error)."; 371 case CODEINVALID: 372 return "The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code."; 373 case EXTENSION: 374 return "An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized."; 375 case TOOCOSTLY: 376 return "The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT."; 377 case BUSINESSRULE: 378 return "The content/operation failed to pass some business rule and so could not proceed."; 379 case CONFLICT: 380 return "Content could not be accepted because of an edit conflict (i.e. version aware updates). (In a pure RESTful environment, this would be an HTTP 409 error, but this code may be used where the conflict is discovered further into the application architecture.)."; 381 case TRANSIENT: 382 return "Transient processing issues. The system receiving the message may be able to resubmit the same content once an underlying issue is resolved."; 383 case LOCKERROR: 384 return "A resource/record locking failure (usually in an underlying database)."; 385 case NOSTORE: 386 return "The persistent store is unavailable; e.g. the database is down for maintenance or similar action, and the interaction or operation cannot be processed."; 387 case EXCEPTION: 388 return "y."; 389 case TIMEOUT: 390 return "An internal timeout has occurred."; 391 case INCOMPLETE: 392 return "Not all data sources typically accessed could be reached or responded in time, so the returned information might not be complete (applies to search interactions and some operations)."; 393 case THROTTLED: 394 return "The system is not prepared to handle this request due to load management."; 395 case INFORMATIONAL: 396 return "A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.)."; 397 case NULL: 398 return null; 399 default: 400 return "?"; 401 } 402 } 403 404 public String getDisplay() { 405 switch (this) { 406 case INVALID: 407 return "Invalid Content"; 408 case STRUCTURE: 409 return "Structural Issue"; 410 case REQUIRED: 411 return "Required element missing"; 412 case VALUE: 413 return "Element value invalid"; 414 case INVARIANT: 415 return "Validation rule failed"; 416 case SECURITY: 417 return "Security Problem"; 418 case LOGIN: 419 return "Login Required"; 420 case UNKNOWN: 421 return "Unknown User"; 422 case EXPIRED: 423 return "Session Expired"; 424 case FORBIDDEN: 425 return "Forbidden"; 426 case SUPPRESSED: 427 return "Information Suppressed"; 428 case PROCESSING: 429 return "Processing Failure"; 430 case NOTSUPPORTED: 431 return "Content not supported"; 432 case DUPLICATE: 433 return "Duplicate"; 434 case MULTIPLEMATCHES: 435 return "Multiple Matches"; 436 case NOTFOUND: 437 return "Not Found"; 438 case DELETED: 439 return "Deleted"; 440 case TOOLONG: 441 return "Content Too Long"; 442 case CODEINVALID: 443 return "Invalid Code"; 444 case EXTENSION: 445 return "Unacceptable Extension"; 446 case TOOCOSTLY: 447 return "Operation Too Costly"; 448 case BUSINESSRULE: 449 return "Business Rule Violation"; 450 case CONFLICT: 451 return "Edit Version Conflict"; 452 case TRANSIENT: 453 return "Transient Issue"; 454 case LOCKERROR: 455 return "Lock Error"; 456 case NOSTORE: 457 return "No Store Available"; 458 case EXCEPTION: 459 return "Exception"; 460 case TIMEOUT: 461 return "Timeout"; 462 case INCOMPLETE: 463 return "Incomplete Results"; 464 case THROTTLED: 465 return "Throttled"; 466 case INFORMATIONAL: 467 return "Informational Note"; 468 case NULL: 469 return null; 470 default: 471 return "?"; 472 } 473 } 474 475}