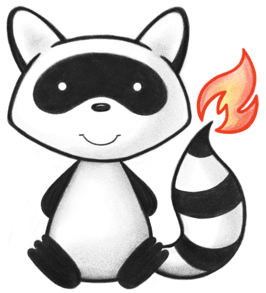
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ListEmptyReason { 037 038 /** 039 * Clinical judgment that there are no known items for this list after 040 * reasonable investigation. Note that this a positive statement by a clinical 041 * user, and not a default position asserted by a computer system in the lack of 042 * other information. Example uses: * For allergies: the patient or patient's 043 * agent/guardian has asserted that he/she is not aware of any allergies (NKA - 044 * nil known allergies) * For medications: the patient or patient's 045 * agent/guardian has asserted that the patient is known to be taking no 046 * medications * For diagnoses, problems and procedures: the patient or 047 * patient's agent/guardian has asserted that there is no known event to record. 048 */ 049 NILKNOWN, 050 /** 051 * The investigation to find out whether there are items for this list has not 052 * occurred. 053 */ 054 NOTASKED, 055 /** 056 * The content of the list was not provided due to privacy or confidentiality 057 * concerns. Note that it should not be assumed that this means that the 058 * particular information in question was withheld due to its contents - it can 059 * also be a policy decision. 060 */ 061 WITHHELD, 062 /** 063 * Information to populate this list cannot be obtained; e.g. unconscious 064 * patient. 065 */ 066 UNAVAILABLE, 067 /** 068 * The work to populate this list has not yet begun. 069 */ 070 NOTSTARTED, 071 /** 072 * This list has now closed or has ceased to be relevant or useful. 073 */ 074 CLOSED, 075 /** 076 * added to help the parsers 077 */ 078 NULL; 079 080 public static ListEmptyReason fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("nilknown".equals(codeString)) 084 return NILKNOWN; 085 if ("notasked".equals(codeString)) 086 return NOTASKED; 087 if ("withheld".equals(codeString)) 088 return WITHHELD; 089 if ("unavailable".equals(codeString)) 090 return UNAVAILABLE; 091 if ("notstarted".equals(codeString)) 092 return NOTSTARTED; 093 if ("closed".equals(codeString)) 094 return CLOSED; 095 throw new FHIRException("Unknown ListEmptyReason code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case NILKNOWN: 101 return "nilknown"; 102 case NOTASKED: 103 return "notasked"; 104 case WITHHELD: 105 return "withheld"; 106 case UNAVAILABLE: 107 return "unavailable"; 108 case NOTSTARTED: 109 return "notstarted"; 110 case CLOSED: 111 return "closed"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getSystem() { 120 return "http://terminology.hl7.org/CodeSystem/list-empty-reason"; 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case NILKNOWN: 126 return "Clinical judgment that there are no known items for this list after reasonable investigation. Note that this a positive statement by a clinical user, and not a default position asserted by a computer system in the lack of other information. Example uses: * For allergies: the patient or patient's agent/guardian has asserted that he/she is not aware of any allergies (NKA - nil known allergies) * For medications: the patient or patient's agent/guardian has asserted that the patient is known to be taking no medications * For diagnoses, problems and procedures: the patient or patient's agent/guardian has asserted that there is no known event to record."; 127 case NOTASKED: 128 return "The investigation to find out whether there are items for this list has not occurred."; 129 case WITHHELD: 130 return "The content of the list was not provided due to privacy or confidentiality concerns. Note that it should not be assumed that this means that the particular information in question was withheld due to its contents - it can also be a policy decision."; 131 case UNAVAILABLE: 132 return "Information to populate this list cannot be obtained; e.g. unconscious patient."; 133 case NOTSTARTED: 134 return "The work to populate this list has not yet begun."; 135 case CLOSED: 136 return "This list has now closed or has ceased to be relevant or useful."; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getDisplay() { 145 switch (this) { 146 case NILKNOWN: 147 return "Nil Known"; 148 case NOTASKED: 149 return "Not Asked"; 150 case WITHHELD: 151 return "Information Withheld"; 152 case UNAVAILABLE: 153 return "Unavailable"; 154 case NOTSTARTED: 155 return "Not Started"; 156 case CLOSED: 157 return "Closed"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165}