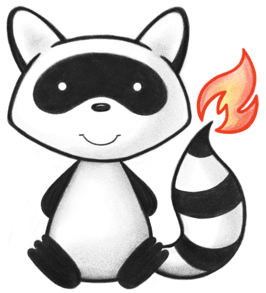
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum LocationPhysicalType { 037 038 /** 039 * A collection of buildings or other locations such as a site or a campus. 040 */ 041 SI, 042 /** 043 * Any Building or structure. This may contain rooms, corridors, wings, etc. It 044 * might not have walls, or a roof, but is considered a defined/allocated space. 045 */ 046 BU, 047 /** 048 * A Wing within a Building, this often contains levels, rooms and corridors. 049 */ 050 WI, 051 /** 052 * A Ward is a section of a medical facility that may contain rooms and other 053 * types of location. 054 */ 055 WA, 056 /** 057 * A Level in a multi-level Building/Structure. 058 */ 059 LVL, 060 /** 061 * Any corridor within a Building, that may connect rooms. 062 */ 063 CO, 064 /** 065 * A space that is allocated as a room, it may have walls/roof etc., but does 066 * not require these. 067 */ 068 RO, 069 /** 070 * A space that is allocated for sleeping/laying on. This is not the physical 071 * bed/trolley that may be moved about, but the space it may occupy. 072 */ 073 BD, 074 /** 075 * A means of transportation. 076 */ 077 VE, 078 /** 079 * A residential dwelling. Usually used to reference a location that a 080 * person/patient may reside. 081 */ 082 HO, 083 /** 084 * A container that can store goods, equipment, medications or other items. 085 */ 086 CA, 087 /** 088 * A defined path to travel between 2 points that has a known name. 089 */ 090 RD, 091 /** 092 * A defined physical boundary of something, such as a flood risk zone, region, 093 * postcode 094 */ 095 AREA, 096 /** 097 * A wide scope that covers a conceptual domain, such as a Nation (Country wide 098 * community or Federal Government - e.g. Ministry of Health), Province or State 099 * (community or Government), Business (throughout the enterprise), Nation with 100 * a business scope of an agency (e.g. CDC, FDA etc.) or a Business segment (UK 101 * Pharmacy), not just an physical boundary 102 */ 103 JDN, 104 /** 105 * added to help the parsers 106 */ 107 NULL; 108 109 public static LocationPhysicalType fromCode(String codeString) throws FHIRException { 110 if (codeString == null || "".equals(codeString)) 111 return null; 112 if ("si".equals(codeString)) 113 return SI; 114 if ("bu".equals(codeString)) 115 return BU; 116 if ("wi".equals(codeString)) 117 return WI; 118 if ("wa".equals(codeString)) 119 return WA; 120 if ("lvl".equals(codeString)) 121 return LVL; 122 if ("co".equals(codeString)) 123 return CO; 124 if ("ro".equals(codeString)) 125 return RO; 126 if ("bd".equals(codeString)) 127 return BD; 128 if ("ve".equals(codeString)) 129 return VE; 130 if ("ho".equals(codeString)) 131 return HO; 132 if ("ca".equals(codeString)) 133 return CA; 134 if ("rd".equals(codeString)) 135 return RD; 136 if ("area".equals(codeString)) 137 return AREA; 138 if ("jdn".equals(codeString)) 139 return JDN; 140 throw new FHIRException("Unknown LocationPhysicalType code '" + codeString + "'"); 141 } 142 143 public String toCode() { 144 switch (this) { 145 case SI: 146 return "si"; 147 case BU: 148 return "bu"; 149 case WI: 150 return "wi"; 151 case WA: 152 return "wa"; 153 case LVL: 154 return "lvl"; 155 case CO: 156 return "co"; 157 case RO: 158 return "ro"; 159 case BD: 160 return "bd"; 161 case VE: 162 return "ve"; 163 case HO: 164 return "ho"; 165 case CA: 166 return "ca"; 167 case RD: 168 return "rd"; 169 case AREA: 170 return "area"; 171 case JDN: 172 return "jdn"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 180 public String getSystem() { 181 return "http://terminology.hl7.org/CodeSystem/location-physical-type"; 182 } 183 184 public String getDefinition() { 185 switch (this) { 186 case SI: 187 return "A collection of buildings or other locations such as a site or a campus."; 188 case BU: 189 return "Any Building or structure. This may contain rooms, corridors, wings, etc. It might not have walls, or a roof, but is considered a defined/allocated space."; 190 case WI: 191 return "A Wing within a Building, this often contains levels, rooms and corridors."; 192 case WA: 193 return "A Ward is a section of a medical facility that may contain rooms and other types of location."; 194 case LVL: 195 return "A Level in a multi-level Building/Structure."; 196 case CO: 197 return "Any corridor within a Building, that may connect rooms."; 198 case RO: 199 return "A space that is allocated as a room, it may have walls/roof etc., but does not require these."; 200 case BD: 201 return "A space that is allocated for sleeping/laying on. This is not the physical bed/trolley that may be moved about, but the space it may occupy."; 202 case VE: 203 return "A means of transportation."; 204 case HO: 205 return "A residential dwelling. Usually used to reference a location that a person/patient may reside."; 206 case CA: 207 return "A container that can store goods, equipment, medications or other items."; 208 case RD: 209 return "A defined path to travel between 2 points that has a known name."; 210 case AREA: 211 return "A defined physical boundary of something, such as a flood risk zone, region, postcode"; 212 case JDN: 213 return "A wide scope that covers a conceptual domain, such as a Nation (Country wide community or Federal Government - e.g. Ministry of Health), Province or State (community or Government), Business (throughout the enterprise), Nation with a business scope of an agency (e.g. CDC, FDA etc.) or a Business segment (UK Pharmacy), not just an physical boundary"; 214 case NULL: 215 return null; 216 default: 217 return "?"; 218 } 219 } 220 221 public String getDisplay() { 222 switch (this) { 223 case SI: 224 return "Site"; 225 case BU: 226 return "Building"; 227 case WI: 228 return "Wing"; 229 case WA: 230 return "Ward"; 231 case LVL: 232 return "Level"; 233 case CO: 234 return "Corridor"; 235 case RO: 236 return "Room"; 237 case BD: 238 return "Bed"; 239 case VE: 240 return "Vehicle"; 241 case HO: 242 return "House"; 243 case CA: 244 return "Cabinet"; 245 case RD: 246 return "Road"; 247 case AREA: 248 return "Area"; 249 case JDN: 250 return "Jurisdiction"; 251 case NULL: 252 return null; 253 default: 254 return "?"; 255 } 256 } 257 258}