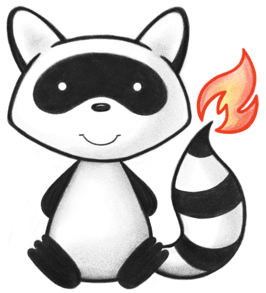
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MediaModality { 037 038 /** 039 * A diagram. Often used in diagnostic reports 040 */ 041 DIAGRAM, 042 /** 043 * A digital record of a fax document 044 */ 045 FAX, 046 /** 047 * A digital scan of a document. This is reserved for when there is not enough 048 * metadata to create a document reference 049 */ 050 SCAN, 051 /** 052 * A retinal image used for identification purposes 053 */ 054 RETINA, 055 /** 056 * A finger print scan used for identification purposes 057 */ 058 FINGERPRINT, 059 /** 060 * An iris scan used for identification purposes 061 */ 062 IRIS, 063 /** 064 * A palm scan used for identification purposes 065 */ 066 PALM, 067 /** 068 * A face scan used for identification purposes 069 */ 070 FACE, 071 /** 072 * added to help the parsers 073 */ 074 NULL; 075 076 public static MediaModality fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("diagram".equals(codeString)) 080 return DIAGRAM; 081 if ("fax".equals(codeString)) 082 return FAX; 083 if ("scan".equals(codeString)) 084 return SCAN; 085 if ("retina".equals(codeString)) 086 return RETINA; 087 if ("fingerprint".equals(codeString)) 088 return FINGERPRINT; 089 if ("iris".equals(codeString)) 090 return IRIS; 091 if ("palm".equals(codeString)) 092 return PALM; 093 if ("face".equals(codeString)) 094 return FACE; 095 throw new FHIRException("Unknown MediaModality code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case DIAGRAM: 101 return "diagram"; 102 case FAX: 103 return "fax"; 104 case SCAN: 105 return "scan"; 106 case RETINA: 107 return "retina"; 108 case FINGERPRINT: 109 return "fingerprint"; 110 case IRIS: 111 return "iris"; 112 case PALM: 113 return "palm"; 114 case FACE: 115 return "face"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 return "http://terminology.hl7.org/CodeSystem/media-modality"; 125 } 126 127 public String getDefinition() { 128 switch (this) { 129 case DIAGRAM: 130 return "A diagram. Often used in diagnostic reports"; 131 case FAX: 132 return "A digital record of a fax document"; 133 case SCAN: 134 return "A digital scan of a document. This is reserved for when there is not enough metadata to create a document reference"; 135 case RETINA: 136 return "A retinal image used for identification purposes"; 137 case FINGERPRINT: 138 return "A finger print scan used for identification purposes"; 139 case IRIS: 140 return "An iris scan used for identification purposes"; 141 case PALM: 142 return "A palm scan used for identification purposes"; 143 case FACE: 144 return "A face scan used for identification purposes"; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 152 public String getDisplay() { 153 switch (this) { 154 case DIAGRAM: 155 return "Diagram"; 156 case FAX: 157 return "Fax"; 158 case SCAN: 159 return "Scanned Document"; 160 case RETINA: 161 return "Retina Scan"; 162 case FINGERPRINT: 163 return "Fingerprint"; 164 case IRIS: 165 return "Iris Scan"; 166 case PALM: 167 return "Palm Scan"; 168 case FACE: 169 return "Face Scan"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 177}