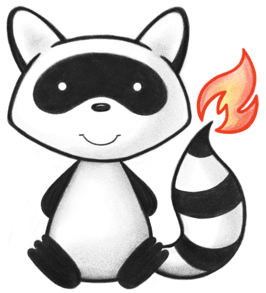
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MedicationStatementStatus { 037 038 /** 039 * The medication is still being taken. 040 */ 041 ACTIVE, 042 /** 043 * The medication is no longer being taken. 044 */ 045 COMPLETED, 046 /** 047 * Some of the actions that are implied by the medication statement may have 048 * occurred. For example, the patient may have taken some of the medication. 049 * Clinical decision support systems should take this status into account. 050 */ 051 ENTEREDINERROR, 052 /** 053 * The medication may be taken at some time in the future. 054 */ 055 INTENDED, 056 /** 057 * Actions implied by the statement have been permanently halted, before all of 058 * them occurred. This should not be used if the statement was entered in error. 059 */ 060 STOPPED, 061 /** 062 * Actions implied by the statement have been temporarily halted, but are 063 * expected to continue later. May also be called 'suspended'. 064 */ 065 ONHOLD, 066 /** 067 * The state of the medication use is not currently known. 068 */ 069 UNKNOWN, 070 /** 071 * The medication was not consumed by the patient 072 */ 073 NOTTAKEN, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 079 public static MedicationStatementStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("active".equals(codeString)) 083 return ACTIVE; 084 if ("completed".equals(codeString)) 085 return COMPLETED; 086 if ("entered-in-error".equals(codeString)) 087 return ENTEREDINERROR; 088 if ("intended".equals(codeString)) 089 return INTENDED; 090 if ("stopped".equals(codeString)) 091 return STOPPED; 092 if ("on-hold".equals(codeString)) 093 return ONHOLD; 094 if ("unknown".equals(codeString)) 095 return UNKNOWN; 096 if ("not-taken".equals(codeString)) 097 return NOTTAKEN; 098 throw new FHIRException("Unknown MedicationStatementStatus code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case ACTIVE: 104 return "active"; 105 case COMPLETED: 106 return "completed"; 107 case ENTEREDINERROR: 108 return "entered-in-error"; 109 case INTENDED: 110 return "intended"; 111 case STOPPED: 112 return "stopped"; 113 case ONHOLD: 114 return "on-hold"; 115 case UNKNOWN: 116 return "unknown"; 117 case NOTTAKEN: 118 return "not-taken"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getSystem() { 127 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 128 } 129 130 public String getDefinition() { 131 switch (this) { 132 case ACTIVE: 133 return "The medication is still being taken."; 134 case COMPLETED: 135 return "The medication is no longer being taken."; 136 case ENTEREDINERROR: 137 return "Some of the actions that are implied by the medication statement may have occurred. For example, the patient may have taken some of the medication. Clinical decision support systems should take this status into account."; 138 case INTENDED: 139 return "The medication may be taken at some time in the future."; 140 case STOPPED: 141 return "Actions implied by the statement have been permanently halted, before all of them occurred. This should not be used if the statement was entered in error."; 142 case ONHOLD: 143 return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called 'suspended'."; 144 case UNKNOWN: 145 return "The state of the medication use is not currently known."; 146 case NOTTAKEN: 147 return "The medication was not consumed by the patient"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDisplay() { 156 switch (this) { 157 case ACTIVE: 158 return "Active"; 159 case COMPLETED: 160 return "Completed"; 161 case ENTEREDINERROR: 162 return "Entered in Error"; 163 case INTENDED: 164 return "Intended"; 165 case STOPPED: 166 return "Stopped"; 167 case ONHOLD: 168 return "On Hold"; 169 case UNKNOWN: 170 return "Unknown"; 171 case NOTTAKEN: 172 return "Not Taken"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 180}