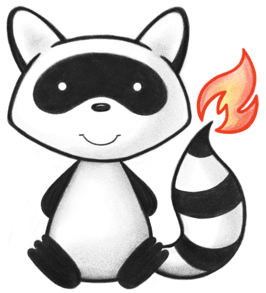
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MedicationknowledgePackageType { 037 038 /** 039 * null 040 */ 041 AMP, 042 /** 043 * null 044 */ 045 BAG, 046 /** 047 * null 048 */ 049 BLSTRPK, 050 /** 051 * null 052 */ 053 BOT, 054 /** 055 * null 056 */ 057 BOX, 058 /** 059 * null 060 */ 061 CAN, 062 /** 063 * null 064 */ 065 CART, 066 /** 067 * null 068 */ 069 DISK, 070 /** 071 * null 072 */ 073 DOSET, 074 /** 075 * null 076 */ 077 JAR, 078 /** 079 * null 080 */ 081 JUG, 082 /** 083 * null 084 */ 085 MINIM, 086 /** 087 * null 088 */ 089 NEBAMP, 090 /** 091 * null 092 */ 093 OVUL, 094 /** 095 * null 096 */ 097 PCH, 098 /** 099 * null 100 */ 101 PKT, 102 /** 103 * null 104 */ 105 SASH, 106 /** 107 * null 108 */ 109 STRIP, 110 /** 111 * null 112 */ 113 TIN, 114 /** 115 * null 116 */ 117 TUB, 118 /** 119 * null 120 */ 121 TUBE, 122 /** 123 * null 124 */ 125 VIAL, 126 /** 127 * added to help the parsers 128 */ 129 NULL; 130 131 public static MedicationknowledgePackageType fromCode(String codeString) throws FHIRException { 132 if (codeString == null || "".equals(codeString)) 133 return null; 134 if ("amp".equals(codeString)) 135 return AMP; 136 if ("bag".equals(codeString)) 137 return BAG; 138 if ("blstrpk".equals(codeString)) 139 return BLSTRPK; 140 if ("bot".equals(codeString)) 141 return BOT; 142 if ("box".equals(codeString)) 143 return BOX; 144 if ("can".equals(codeString)) 145 return CAN; 146 if ("cart".equals(codeString)) 147 return CART; 148 if ("disk".equals(codeString)) 149 return DISK; 150 if ("doset".equals(codeString)) 151 return DOSET; 152 if ("jar".equals(codeString)) 153 return JAR; 154 if ("jug".equals(codeString)) 155 return JUG; 156 if ("minim".equals(codeString)) 157 return MINIM; 158 if ("nebamp".equals(codeString)) 159 return NEBAMP; 160 if ("ovul".equals(codeString)) 161 return OVUL; 162 if ("pch".equals(codeString)) 163 return PCH; 164 if ("pkt".equals(codeString)) 165 return PKT; 166 if ("sash".equals(codeString)) 167 return SASH; 168 if ("strip".equals(codeString)) 169 return STRIP; 170 if ("tin".equals(codeString)) 171 return TIN; 172 if ("tub".equals(codeString)) 173 return TUB; 174 if ("tube".equals(codeString)) 175 return TUBE; 176 if ("vial".equals(codeString)) 177 return VIAL; 178 throw new FHIRException("Unknown MedicationknowledgePackageType code '" + codeString + "'"); 179 } 180 181 public String toCode() { 182 switch (this) { 183 case AMP: 184 return "amp"; 185 case BAG: 186 return "bag"; 187 case BLSTRPK: 188 return "blstrpk"; 189 case BOT: 190 return "bot"; 191 case BOX: 192 return "box"; 193 case CAN: 194 return "can"; 195 case CART: 196 return "cart"; 197 case DISK: 198 return "disk"; 199 case DOSET: 200 return "doset"; 201 case JAR: 202 return "jar"; 203 case JUG: 204 return "jug"; 205 case MINIM: 206 return "minim"; 207 case NEBAMP: 208 return "nebamp"; 209 case OVUL: 210 return "ovul"; 211 case PCH: 212 return "pch"; 213 case PKT: 214 return "pkt"; 215 case SASH: 216 return "sash"; 217 case STRIP: 218 return "strip"; 219 case TIN: 220 return "tin"; 221 case TUB: 222 return "tub"; 223 case TUBE: 224 return "tube"; 225 case VIAL: 226 return "vial"; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 234 public String getSystem() { 235 return "http://terminology.hl7.org/CodeSystem/medicationknowledge-package-type"; 236 } 237 238 public String getDefinition() { 239 switch (this) { 240 case AMP: 241 return ""; 242 case BAG: 243 return ""; 244 case BLSTRPK: 245 return ""; 246 case BOT: 247 return ""; 248 case BOX: 249 return ""; 250 case CAN: 251 return ""; 252 case CART: 253 return ""; 254 case DISK: 255 return ""; 256 case DOSET: 257 return ""; 258 case JAR: 259 return ""; 260 case JUG: 261 return ""; 262 case MINIM: 263 return ""; 264 case NEBAMP: 265 return ""; 266 case OVUL: 267 return ""; 268 case PCH: 269 return ""; 270 case PKT: 271 return ""; 272 case SASH: 273 return ""; 274 case STRIP: 275 return ""; 276 case TIN: 277 return ""; 278 case TUB: 279 return ""; 280 case TUBE: 281 return ""; 282 case VIAL: 283 return ""; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 291 public String getDisplay() { 292 switch (this) { 293 case AMP: 294 return "Ampule"; 295 case BAG: 296 return "Bag"; 297 case BLSTRPK: 298 return "Blister Pack"; 299 case BOT: 300 return "Bottle"; 301 case BOX: 302 return "Box"; 303 case CAN: 304 return "Can"; 305 case CART: 306 return "Cartridge"; 307 case DISK: 308 return "Disk"; 309 case DOSET: 310 return "Dosette"; 311 case JAR: 312 return "Jar"; 313 case JUG: 314 return "Jug"; 315 case MINIM: 316 return "Minim"; 317 case NEBAMP: 318 return "Nebule Amp"; 319 case OVUL: 320 return "Ovule"; 321 case PCH: 322 return "Pouch"; 323 case PKT: 324 return "Packet"; 325 case SASH: 326 return "Sashet"; 327 case STRIP: 328 return "Strip"; 329 case TIN: 330 return "Tin"; 331 case TUB: 332 return "Tub"; 333 case TUBE: 334 return "Tube"; 335 case VIAL: 336 return "Vial"; 337 case NULL: 338 return null; 339 default: 340 return "?"; 341 } 342 } 343 344}