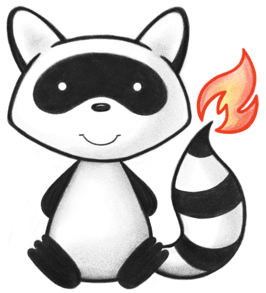
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MedicationrequestIntent { 037 038 /** 039 * The request is a suggestion made by someone/something that doesn't have an 040 * intention to ensure it occurs and without providing an authorization to act 041 */ 042 PROPOSAL, 043 /** 044 * The request represents an intention to ensure something occurs without 045 * providing an authorization for others to act. 046 */ 047 PLAN, 048 /** 049 * The request represents a request/demand and authorization for action 050 */ 051 ORDER, 052 /** 053 * The request represents the original authorization for the medication request. 054 */ 055 ORIGINALORDER, 056 /** 057 * The request represents an automatically generated supplemental authorization 058 * for action based on a parent authorization together with initial results of 059 * the action taken against that parent authorization.. 060 */ 061 REFLEXORDER, 062 /** 063 * The request represents the view of an authorization instantiated by a 064 * fulfilling system representing the details of the fulfiller's intention to 065 * act upon a submitted order. 066 */ 067 FILLERORDER, 068 /** 069 * The request represents an instance for the particular order, for example a 070 * medication administration record. 071 */ 072 INSTANCEORDER, 073 /** 074 * The request represents a component or option for a RequestGroup that 075 * establishes timing, conditionality and/or other constraints among a set of 076 * requests. 077 */ 078 OPTION, 079 /** 080 * added to help the parsers 081 */ 082 NULL; 083 084 public static MedicationrequestIntent fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("proposal".equals(codeString)) 088 return PROPOSAL; 089 if ("plan".equals(codeString)) 090 return PLAN; 091 if ("order".equals(codeString)) 092 return ORDER; 093 if ("original-order".equals(codeString)) 094 return ORIGINALORDER; 095 if ("reflex-order".equals(codeString)) 096 return REFLEXORDER; 097 if ("filler-order".equals(codeString)) 098 return FILLERORDER; 099 if ("instance-order".equals(codeString)) 100 return INSTANCEORDER; 101 if ("option".equals(codeString)) 102 return OPTION; 103 throw new FHIRException("Unknown MedicationrequestIntent code '" + codeString + "'"); 104 } 105 106 public String toCode() { 107 switch (this) { 108 case PROPOSAL: 109 return "proposal"; 110 case PLAN: 111 return "plan"; 112 case ORDER: 113 return "order"; 114 case ORIGINALORDER: 115 return "original-order"; 116 case REFLEXORDER: 117 return "reflex-order"; 118 case FILLERORDER: 119 return "filler-order"; 120 case INSTANCEORDER: 121 return "instance-order"; 122 case OPTION: 123 return "option"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getSystem() { 132 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case PROPOSAL: 138 return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 139 case PLAN: 140 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 141 case ORDER: 142 return "The request represents a request/demand and authorization for action"; 143 case ORIGINALORDER: 144 return "The request represents the original authorization for the medication request."; 145 case REFLEXORDER: 146 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization.."; 147 case FILLERORDER: 148 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 149 case INSTANCEORDER: 150 return "The request represents an instance for the particular order, for example a medication administration record."; 151 case OPTION: 152 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case PROPOSAL: 163 return "Proposal"; 164 case PLAN: 165 return "Plan"; 166 case ORDER: 167 return "Order"; 168 case ORIGINALORDER: 169 return "Original Order"; 170 case REFLEXORDER: 171 return "Reflex Order"; 172 case FILLERORDER: 173 return "Filler Order"; 174 case INSTANCEORDER: 175 return "Instance Order"; 176 case OPTION: 177 return "Option"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 185}