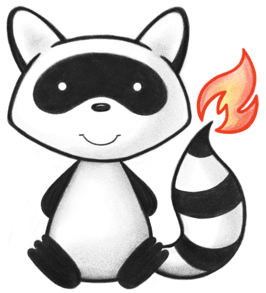
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MedicationrequestStatus { 037 038 /** 039 * The prescription is 'actionable', but not all actions that are implied by it 040 * have occurred yet. 041 */ 042 ACTIVE, 043 /** 044 * Actions implied by the prescription are to be temporarily halted, but are 045 * expected to continue later. May also be called 'suspended'. 046 */ 047 ONHOLD, 048 /** 049 * The prescription has been withdrawn before any administrations have occurred 050 */ 051 CANCELLED, 052 /** 053 * All actions that are implied by the prescription have occurred. 054 */ 055 COMPLETED, 056 /** 057 * Some of the actions that are implied by the medication request may have 058 * occurred. For example, the medication may have been dispensed and the patient 059 * may have taken some of the medication. Clinical decision support systems 060 * should take this status into account 061 */ 062 ENTEREDINERROR, 063 /** 064 * Actions implied by the prescription are to be permanently halted, before all 065 * of the administrations occurred. This should not be used if the original 066 * order was entered in error 067 */ 068 STOPPED, 069 /** 070 * The prescription is not yet 'actionable', e.g. it is a work in progress, 071 * requires sign-off, verification or needs to be run through decision support 072 * process. 073 */ 074 DRAFT, 075 /** 076 * The authoring/source system does not know which of the status values 077 * currently applies for this observation. Note: This concept is not to be used 078 * for 'other' - one of the listed statuses is presumed to apply, but the 079 * authoring/source system does not know which. 080 */ 081 UNKNOWN, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static MedicationrequestStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("active".equals(codeString)) 091 return ACTIVE; 092 if ("on-hold".equals(codeString)) 093 return ONHOLD; 094 if ("cancelled".equals(codeString)) 095 return CANCELLED; 096 if ("completed".equals(codeString)) 097 return COMPLETED; 098 if ("entered-in-error".equals(codeString)) 099 return ENTEREDINERROR; 100 if ("stopped".equals(codeString)) 101 return STOPPED; 102 if ("draft".equals(codeString)) 103 return DRAFT; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 throw new FHIRException("Unknown MedicationrequestStatus code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case ACTIVE: 112 return "active"; 113 case ONHOLD: 114 return "on-hold"; 115 case CANCELLED: 116 return "cancelled"; 117 case COMPLETED: 118 return "completed"; 119 case ENTEREDINERROR: 120 return "entered-in-error"; 121 case STOPPED: 122 return "stopped"; 123 case DRAFT: 124 return "draft"; 125 case UNKNOWN: 126 return "unknown"; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getSystem() { 135 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case ACTIVE: 141 return "The prescription is 'actionable', but not all actions that are implied by it have occurred yet."; 142 case ONHOLD: 143 return "Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called 'suspended'."; 144 case CANCELLED: 145 return "The prescription has been withdrawn before any administrations have occurred"; 146 case COMPLETED: 147 return "All actions that are implied by the prescription have occurred."; 148 case ENTEREDINERROR: 149 return "Some of the actions that are implied by the medication request may have occurred. For example, the medication may have been dispensed and the patient may have taken some of the medication. Clinical decision support systems should take this status into account"; 150 case STOPPED: 151 return "Actions implied by the prescription are to be permanently halted, before all of the administrations occurred. This should not be used if the original order was entered in error"; 152 case DRAFT: 153 return "The prescription is not yet 'actionable', e.g. it is a work in progress, requires sign-off, verification or needs to be run through decision support process."; 154 case UNKNOWN: 155 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 163 public String getDisplay() { 164 switch (this) { 165 case ACTIVE: 166 return "Active"; 167 case ONHOLD: 168 return "On Hold"; 169 case CANCELLED: 170 return "Cancelled"; 171 case COMPLETED: 172 return "Completed"; 173 case ENTEREDINERROR: 174 return "Entered in Error"; 175 case STOPPED: 176 return "Stopped"; 177 case DRAFT: 178 return "Draft"; 179 case UNKNOWN: 180 return "Unknown"; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188}