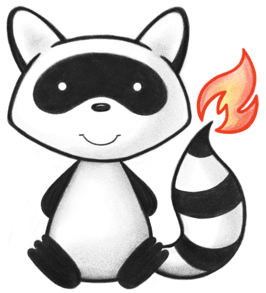
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MedicationrequestStatusReason { 037 038 /** 039 * This therapy has been ordered as a backup to a preferred therapy. This order 040 * will be released when and if the preferred therapy is unsuccessful. 041 */ 042 ALTCHOICE, 043 /** 044 * Clarification is required before the order can be acted upon. 045 */ 046 CLARIF, 047 /** 048 * The current level of the medication in the patient's system is too high. The 049 * medication is suspended to allow the level to subside to a safer level. 050 */ 051 DRUGHIGH, 052 /** 053 * The patient has been admitted to a care facility and their community 054 * medications are suspended until hospital discharge. 055 */ 056 HOSPADM, 057 /** 058 * The therapy would interfere with a planned lab test and the therapy is being 059 * withdrawn until the test is completed. 060 */ 061 LABINT, 062 /** 063 * Patient not available for a period of time due to a scheduled therapy, leave 064 * of absence or other reason. 065 */ 066 NONAVAIL, 067 /** 068 * The patient is pregnant or breast feeding. The therapy will be resumed when 069 * the pregnancy is complete and the patient is no longer breastfeeding. 070 */ 071 PREG, 072 /** 073 * The patient is believed to be allergic to a substance that is part of the 074 * therapy and the therapy is being temporarily withdrawn to confirm. 075 */ 076 SALG, 077 /** 078 * The drug interacts with a short-term treatment that is more urgently 079 * required. This order will be resumed when the short-term treatment is 080 * complete. 081 */ 082 SDDI, 083 /** 084 * The drug interacts with a short-term treatment that is more urgently 085 * required. This order will be resumed when the short-term treatment is 086 * complete. 087 */ 088 SDUPTHER, 089 /** 090 * The drug interacts with a short-term treatment that is more urgently 091 * required. This order will be resumed when the short-term treatment is 092 * complete. 093 */ 094 SINTOL, 095 /** 096 * The drug is contraindicated for patients receiving surgery and the patient is 097 * scheduled to be admitted for surgery in the near future. The drug will be 098 * resumed when the patient has sufficiently recovered from the surgery. 099 */ 100 SURG, 101 /** 102 * The patient was previously receiving a medication contraindicated with the 103 * current medication. The current medication will remain on hold until the 104 * prior medication has been cleansed from their system. 105 */ 106 WASHOUT, 107 /** 108 * added to help the parsers 109 */ 110 NULL; 111 112 public static MedicationrequestStatusReason fromCode(String codeString) throws FHIRException { 113 if (codeString == null || "".equals(codeString)) 114 return null; 115 if ("altchoice".equals(codeString)) 116 return ALTCHOICE; 117 if ("clarif".equals(codeString)) 118 return CLARIF; 119 if ("drughigh".equals(codeString)) 120 return DRUGHIGH; 121 if ("hospadm".equals(codeString)) 122 return HOSPADM; 123 if ("labint".equals(codeString)) 124 return LABINT; 125 if ("non-avail".equals(codeString)) 126 return NONAVAIL; 127 if ("preg".equals(codeString)) 128 return PREG; 129 if ("salg".equals(codeString)) 130 return SALG; 131 if ("sddi".equals(codeString)) 132 return SDDI; 133 if ("sdupther".equals(codeString)) 134 return SDUPTHER; 135 if ("sintol".equals(codeString)) 136 return SINTOL; 137 if ("surg".equals(codeString)) 138 return SURG; 139 if ("washout".equals(codeString)) 140 return WASHOUT; 141 throw new FHIRException("Unknown MedicationrequestStatusReason code '" + codeString + "'"); 142 } 143 144 public String toCode() { 145 switch (this) { 146 case ALTCHOICE: 147 return "altchoice"; 148 case CLARIF: 149 return "clarif"; 150 case DRUGHIGH: 151 return "drughigh"; 152 case HOSPADM: 153 return "hospadm"; 154 case LABINT: 155 return "labint"; 156 case NONAVAIL: 157 return "non-avail"; 158 case PREG: 159 return "preg"; 160 case SALG: 161 return "salg"; 162 case SDDI: 163 return "sddi"; 164 case SDUPTHER: 165 return "sdupther"; 166 case SINTOL: 167 return "sintol"; 168 case SURG: 169 return "surg"; 170 case WASHOUT: 171 return "washout"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getSystem() { 180 return "http://terminology.hl7.org/CodeSystem/medicationrequest-status-reason"; 181 } 182 183 public String getDefinition() { 184 switch (this) { 185 case ALTCHOICE: 186 return "This therapy has been ordered as a backup to a preferred therapy. This order will be released when and if the preferred therapy is unsuccessful."; 187 case CLARIF: 188 return "Clarification is required before the order can be acted upon."; 189 case DRUGHIGH: 190 return "The current level of the medication in the patient's system is too high. The medication is suspended to allow the level to subside to a safer level."; 191 case HOSPADM: 192 return "The patient has been admitted to a care facility and their community medications are suspended until hospital discharge."; 193 case LABINT: 194 return "The therapy would interfere with a planned lab test and the therapy is being withdrawn until the test is completed."; 195 case NONAVAIL: 196 return "Patient not available for a period of time due to a scheduled therapy, leave of absence or other reason."; 197 case PREG: 198 return "The patient is pregnant or breast feeding. The therapy will be resumed when the pregnancy is complete and the patient is no longer breastfeeding."; 199 case SALG: 200 return "The patient is believed to be allergic to a substance that is part of the therapy and the therapy is being temporarily withdrawn to confirm."; 201 case SDDI: 202 return "The drug interacts with a short-term treatment that is more urgently required. This order will be resumed when the short-term treatment is complete."; 203 case SDUPTHER: 204 return "The drug interacts with a short-term treatment that is more urgently required. This order will be resumed when the short-term treatment is complete."; 205 case SINTOL: 206 return "The drug interacts with a short-term treatment that is more urgently required. This order will be resumed when the short-term treatment is complete."; 207 case SURG: 208 return "The drug is contraindicated for patients receiving surgery and the patient is scheduled to be admitted for surgery in the near future. The drug will be resumed when the patient has sufficiently recovered from the surgery."; 209 case WASHOUT: 210 return "The patient was previously receiving a medication contraindicated with the current medication. The current medication will remain on hold until the prior medication has been cleansed from their system."; 211 case NULL: 212 return null; 213 default: 214 return "?"; 215 } 216 } 217 218 public String getDisplay() { 219 switch (this) { 220 case ALTCHOICE: 221 return "Try another treatment first"; 222 case CLARIF: 223 return "Prescription requires clarification"; 224 case DRUGHIGH: 225 return "Drug level too high"; 226 case HOSPADM: 227 return "Admission to hospital"; 228 case LABINT: 229 return "Lab interference issues"; 230 case NONAVAIL: 231 return "Patient not available"; 232 case PREG: 233 return "Parent is pregnant/breast feeding"; 234 case SALG: 235 return "Allergy"; 236 case SDDI: 237 return "Drug interacts with another drug"; 238 case SDUPTHER: 239 return "Duplicate therapy"; 240 case SINTOL: 241 return "Suspected intolerance"; 242 case SURG: 243 return "Patient scheduled for surgery."; 244 case WASHOUT: 245 return "Waiting for old drug to wash out"; 246 case NULL: 247 return null; 248 default: 249 return "?"; 250 } 251 } 252 253}