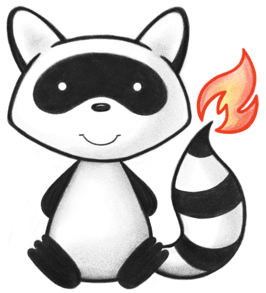
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ObservationCategory { 037 038 /** 039 * Social History Observations define the patient's occupational, personal 040 * (e.g., lifestyle), social, familial, and environmental history and health 041 * risk factors that may impact the patient's health. 042 */ 043 SOCIALHISTORY, 044 /** 045 * Clinical observations measure the body's basic functions such as blood 046 * pressure, heart rate, respiratory rate, height, weight, body mass index, head 047 * circumference, pulse oximetry, temperature, and body surface area. 048 */ 049 VITALSIGNS, 050 /** 051 * Observations generated by imaging. The scope includes observations regarding 052 * plain x-ray, ultrasound, CT, MRI, angiography, echocardiography, and nuclear 053 * medicine. 054 */ 055 IMAGING, 056 /** 057 * The results of observations generated by laboratories. Laboratory results are 058 * typically generated by laboratories providing analytic services in areas such 059 * as chemistry, hematology, serology, histology, cytology, anatomic pathology 060 * (including digital pathology), microbiology, and/or virology. These 061 * observations are based on analysis of specimens obtained from the patient and 062 * submitted to the laboratory. 063 */ 064 LABORATORY, 065 /** 066 * Observations generated by other procedures. This category includes 067 * observations resulting from interventional and non-interventional procedures 068 * excluding laboratory and imaging (e.g., cardiology catheterization, 069 * endoscopy, electrodiagnostics, etc.). Procedure results are typically 070 * generated by a clinician to provide more granular information about component 071 * observations made during a procedure. An example would be when a 072 * gastroenterologist reports the size of a polyp observed during a colonoscopy. 073 */ 074 PROCEDURE, 075 /** 076 * Assessment tool/survey instrument observations (e.g., Apgar Scores, Montreal 077 * Cognitive Assessment (MoCA)). 078 */ 079 SURVEY, 080 /** 081 * Observations generated by physical exam findings including direct 082 * observations made by a clinician and use of simple instruments and the result 083 * of simple maneuvers performed directly on the patient's body. 084 */ 085 EXAM, 086 /** 087 * Observations generated by non-interventional treatment protocols (e.g. 088 * occupational, physical, radiation, nutritional and medication therapy) 089 */ 090 THERAPY, 091 /** 092 * Observations that measure or record any bodily activity that enhances or 093 * maintains physical fitness and overall health and wellness. Not under direct 094 * supervision of practitioner such as a physical therapist. (e.g., laps swum, 095 * steps, sleep data) 096 */ 097 ACTIVITY, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 103 public static ObservationCategory fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("social-history".equals(codeString)) 107 return SOCIALHISTORY; 108 if ("vital-signs".equals(codeString)) 109 return VITALSIGNS; 110 if ("imaging".equals(codeString)) 111 return IMAGING; 112 if ("laboratory".equals(codeString)) 113 return LABORATORY; 114 if ("procedure".equals(codeString)) 115 return PROCEDURE; 116 if ("survey".equals(codeString)) 117 return SURVEY; 118 if ("exam".equals(codeString)) 119 return EXAM; 120 if ("therapy".equals(codeString)) 121 return THERAPY; 122 if ("activity".equals(codeString)) 123 return ACTIVITY; 124 throw new FHIRException("Unknown ObservationCategory code '" + codeString + "'"); 125 } 126 127 public String toCode() { 128 switch (this) { 129 case SOCIALHISTORY: 130 return "social-history"; 131 case VITALSIGNS: 132 return "vital-signs"; 133 case IMAGING: 134 return "imaging"; 135 case LABORATORY: 136 return "laboratory"; 137 case PROCEDURE: 138 return "procedure"; 139 case SURVEY: 140 return "survey"; 141 case EXAM: 142 return "exam"; 143 case THERAPY: 144 return "therapy"; 145 case ACTIVITY: 146 return "activity"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 return "http://terminology.hl7.org/CodeSystem/observation-category"; 156 } 157 158 public String getDefinition() { 159 switch (this) { 160 case SOCIALHISTORY: 161 return "Social History Observations define the patient's occupational, personal (e.g., lifestyle), social, familial, and environmental history and health risk factors that may impact the patient's health."; 162 case VITALSIGNS: 163 return " Clinical observations measure the body's basic functions such as blood pressure, heart rate, respiratory rate, height, weight, body mass index, head circumference, pulse oximetry, temperature, and body surface area."; 164 case IMAGING: 165 return "Observations generated by imaging. The scope includes observations regarding plain x-ray, ultrasound, CT, MRI, angiography, echocardiography, and nuclear medicine."; 166 case LABORATORY: 167 return "The results of observations generated by laboratories. Laboratory results are typically generated by laboratories providing analytic services in areas such as chemistry, hematology, serology, histology, cytology, anatomic pathology (including digital pathology), microbiology, and/or virology. These observations are based on analysis of specimens obtained from the patient and submitted to the laboratory."; 168 case PROCEDURE: 169 return "Observations generated by other procedures. This category includes observations resulting from interventional and non-interventional procedures excluding laboratory and imaging (e.g., cardiology catheterization, endoscopy, electrodiagnostics, etc.). Procedure results are typically generated by a clinician to provide more granular information about component observations made during a procedure. An example would be when a gastroenterologist reports the size of a polyp observed during a colonoscopy."; 170 case SURVEY: 171 return "Assessment tool/survey instrument observations (e.g., Apgar Scores, Montreal Cognitive Assessment (MoCA))."; 172 case EXAM: 173 return "Observations generated by physical exam findings including direct observations made by a clinician and use of simple instruments and the result of simple maneuvers performed directly on the patient's body."; 174 case THERAPY: 175 return "Observations generated by non-interventional treatment protocols (e.g. occupational, physical, radiation, nutritional and medication therapy)"; 176 case ACTIVITY: 177 return "Observations that measure or record any bodily activity that enhances or maintains physical fitness and overall health and wellness. Not under direct supervision of practitioner such as a physical therapist. (e.g., laps swum, steps, sleep data)"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 185 public String getDisplay() { 186 switch (this) { 187 case SOCIALHISTORY: 188 return "Social History"; 189 case VITALSIGNS: 190 return "Vital Signs"; 191 case IMAGING: 192 return "Imaging"; 193 case LABORATORY: 194 return "Laboratory"; 195 case PROCEDURE: 196 return "Procedure"; 197 case SURVEY: 198 return "Survey"; 199 case EXAM: 200 return "Exam"; 201 case THERAPY: 202 return "Therapy"; 203 case ACTIVITY: 204 return "Activity"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212}