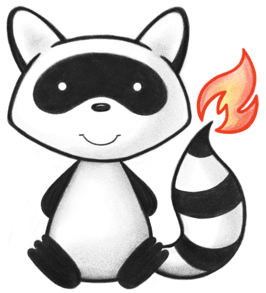
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ObservationStatistics { 037 038 /** 039 * The [mean](https://en.wikipedia.org/wiki/Arithmetic_mean) of N measurements 040 * over the stated period. 041 */ 042 AVERAGE, 043 /** 044 * The [maximum](https://en.wikipedia.org/wiki/Maximal_element) value of N 045 * measurements over the stated period. 046 */ 047 MAXIMUM, 048 /** 049 * The [minimum](https://en.wikipedia.org/wiki/Minimal_element) value of N 050 * measurements over the stated period. 051 */ 052 MINIMUM, 053 /** 054 * The [number] of valid measurements over the stated period that contributed to 055 * the other statistical outputs. 056 */ 057 COUNT, 058 /** 059 * The total [number] of valid measurements over the stated period, including 060 * observations that were ignored because they did not contain valid result 061 * values. 062 */ 063 TOTALCOUNT, 064 /** 065 * The [median](https://en.wikipedia.org/wiki/Median) of N measurements over the 066 * stated period. 067 */ 068 MEDIAN, 069 /** 070 * The [standard deviation](https://en.wikipedia.org/wiki/Standard_deviation) of 071 * N measurements over the stated period. 072 */ 073 STDDEV, 074 /** 075 * The [sum](https://en.wikipedia.org/wiki/Summation) of N measurements over the 076 * stated period. 077 */ 078 SUM, 079 /** 080 * The [variance](https://en.wikipedia.org/wiki/Variance) of N measurements over 081 * the stated period. 082 */ 083 VARIANCE, 084 /** 085 * The 20th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N 086 * measurements over the stated period. 087 */ 088 _20PERCENT, 089 /** 090 * The 80th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N 091 * measurements over the stated period. 092 */ 093 _80PERCENT, 094 /** 095 * The lower [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N 096 * measurements over the stated period. 097 */ 098 _4LOWER, 099 /** 100 * The upper [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N 101 * measurements over the stated period. 102 */ 103 _4UPPER, 104 /** 105 * The difference between the upper and lower 106 * [Quartiles](https://en.wikipedia.org/wiki/Quartile) is called the 107 * Interquartile range. (IQR = Q3-Q1) Quartile deviation or Semi-interquartile 108 * range is one-half the difference between the first and the third quartiles. 109 */ 110 _4DEV, 111 /** 112 * The lowest of four values that divide the N measurements into a frequency 113 * distribution of five classes with each containing one fifth of the total 114 * population. 115 */ 116 _51, 117 /** 118 * The second of four values that divide the N measurements into a frequency 119 * distribution of five classes with each containing one fifth of the total 120 * population. 121 */ 122 _52, 123 /** 124 * The third of four values that divide the N measurements into a frequency 125 * distribution of five classes with each containing one fifth of the total 126 * population. 127 */ 128 _53, 129 /** 130 * The fourth of four values that divide the N measurements into a frequency 131 * distribution of five classes with each containing one fifth of the total 132 * population. 133 */ 134 _54, 135 /** 136 * Skewness is a measure of the asymmetry of the probability distribution of a 137 * real-valued random variable about its mean. The skewness value can be 138 * positive or negative, or even undefined. Source: 139 * [Wikipedia](https://en.wikipedia.org/wiki/Skewness). 140 */ 141 SKEW, 142 /** 143 * Kurtosis is a measure of the "tailedness" of the probability distribution of 144 * a real-valued random variable. Source: 145 * [Wikipedia](https://en.wikipedia.org/wiki/Kurtosis). 146 */ 147 KURTOSIS, 148 /** 149 * Linear regression is an approach for modeling two-dimensional sample points 150 * with one independent variable and one dependent variable (conventionally, the 151 * x and y coordinates in a Cartesian coordinate system) and finds a linear 152 * function (a non-vertical straight line) that, as accurately as possible, 153 * predicts the dependent variable values as a function of the independent 154 * variables. Source: 155 * [Wikipedia](https://en.wikipedia.org/wiki/Simple_linear_regression) This 156 * Statistic code will return both a gradient and an intercept value. 157 */ 158 REGRESSION, 159 /** 160 * added to help the parsers 161 */ 162 NULL; 163 164 public static ObservationStatistics fromCode(String codeString) throws FHIRException { 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("average".equals(codeString)) 168 return AVERAGE; 169 if ("maximum".equals(codeString)) 170 return MAXIMUM; 171 if ("minimum".equals(codeString)) 172 return MINIMUM; 173 if ("count".equals(codeString)) 174 return COUNT; 175 if ("total-count".equals(codeString)) 176 return TOTALCOUNT; 177 if ("median".equals(codeString)) 178 return MEDIAN; 179 if ("std-dev".equals(codeString)) 180 return STDDEV; 181 if ("sum".equals(codeString)) 182 return SUM; 183 if ("variance".equals(codeString)) 184 return VARIANCE; 185 if ("20-percent".equals(codeString)) 186 return _20PERCENT; 187 if ("80-percent".equals(codeString)) 188 return _80PERCENT; 189 if ("4-lower".equals(codeString)) 190 return _4LOWER; 191 if ("4-upper".equals(codeString)) 192 return _4UPPER; 193 if ("4-dev".equals(codeString)) 194 return _4DEV; 195 if ("5-1".equals(codeString)) 196 return _51; 197 if ("5-2".equals(codeString)) 198 return _52; 199 if ("5-3".equals(codeString)) 200 return _53; 201 if ("5-4".equals(codeString)) 202 return _54; 203 if ("skew".equals(codeString)) 204 return SKEW; 205 if ("kurtosis".equals(codeString)) 206 return KURTOSIS; 207 if ("regression".equals(codeString)) 208 return REGRESSION; 209 throw new FHIRException("Unknown ObservationStatistics code '" + codeString + "'"); 210 } 211 212 public String toCode() { 213 switch (this) { 214 case AVERAGE: 215 return "average"; 216 case MAXIMUM: 217 return "maximum"; 218 case MINIMUM: 219 return "minimum"; 220 case COUNT: 221 return "count"; 222 case TOTALCOUNT: 223 return "total-count"; 224 case MEDIAN: 225 return "median"; 226 case STDDEV: 227 return "std-dev"; 228 case SUM: 229 return "sum"; 230 case VARIANCE: 231 return "variance"; 232 case _20PERCENT: 233 return "20-percent"; 234 case _80PERCENT: 235 return "80-percent"; 236 case _4LOWER: 237 return "4-lower"; 238 case _4UPPER: 239 return "4-upper"; 240 case _4DEV: 241 return "4-dev"; 242 case _51: 243 return "5-1"; 244 case _52: 245 return "5-2"; 246 case _53: 247 return "5-3"; 248 case _54: 249 return "5-4"; 250 case SKEW: 251 return "skew"; 252 case KURTOSIS: 253 return "kurtosis"; 254 case REGRESSION: 255 return "regression"; 256 case NULL: 257 return null; 258 default: 259 return "?"; 260 } 261 } 262 263 public String getSystem() { 264 return "http://terminology.hl7.org/CodeSystem/observation-statistics"; 265 } 266 267 public String getDefinition() { 268 switch (this) { 269 case AVERAGE: 270 return "The [mean](https://en.wikipedia.org/wiki/Arithmetic_mean) of N measurements over the stated period."; 271 case MAXIMUM: 272 return "The [maximum](https://en.wikipedia.org/wiki/Maximal_element) value of N measurements over the stated period."; 273 case MINIMUM: 274 return "The [minimum](https://en.wikipedia.org/wiki/Minimal_element) value of N measurements over the stated period."; 275 case COUNT: 276 return "The [number] of valid measurements over the stated period that contributed to the other statistical outputs."; 277 case TOTALCOUNT: 278 return "The total [number] of valid measurements over the stated period, including observations that were ignored because they did not contain valid result values."; 279 case MEDIAN: 280 return "The [median](https://en.wikipedia.org/wiki/Median) of N measurements over the stated period."; 281 case STDDEV: 282 return "The [standard deviation](https://en.wikipedia.org/wiki/Standard_deviation) of N measurements over the stated period."; 283 case SUM: 284 return "The [sum](https://en.wikipedia.org/wiki/Summation) of N measurements over the stated period."; 285 case VARIANCE: 286 return "The [variance](https://en.wikipedia.org/wiki/Variance) of N measurements over the stated period."; 287 case _20PERCENT: 288 return "The 20th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N measurements over the stated period."; 289 case _80PERCENT: 290 return "The 80th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N measurements over the stated period."; 291 case _4LOWER: 292 return "The lower [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N measurements over the stated period."; 293 case _4UPPER: 294 return "The upper [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N measurements over the stated period."; 295 case _4DEV: 296 return "The difference between the upper and lower [Quartiles](https://en.wikipedia.org/wiki/Quartile) is called the Interquartile range. (IQR = Q3-Q1) Quartile deviation or Semi-interquartile range is one-half the difference between the first and the third quartiles."; 297 case _51: 298 return "The lowest of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population."; 299 case _52: 300 return "The second of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population."; 301 case _53: 302 return "The third of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population."; 303 case _54: 304 return "The fourth of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population."; 305 case SKEW: 306 return "Skewness is a measure of the asymmetry of the probability distribution of a real-valued random variable about its mean. The skewness value can be positive or negative, or even undefined. Source: [Wikipedia](https://en.wikipedia.org/wiki/Skewness)."; 307 case KURTOSIS: 308 return "Kurtosis is a measure of the \"tailedness\" of the probability distribution of a real-valued random variable. Source: [Wikipedia](https://en.wikipedia.org/wiki/Kurtosis)."; 309 case REGRESSION: 310 return "Linear regression is an approach for modeling two-dimensional sample points with one independent variable and one dependent variable (conventionally, the x and y coordinates in a Cartesian coordinate system) and finds a linear function (a non-vertical straight line) that, as accurately as possible, predicts the dependent variable values as a function of the independent variables. Source: [Wikipedia](https://en.wikipedia.org/wiki/Simple_linear_regression) This Statistic code will return both a gradient and an intercept value."; 311 case NULL: 312 return null; 313 default: 314 return "?"; 315 } 316 } 317 318 public String getDisplay() { 319 switch (this) { 320 case AVERAGE: 321 return "Average"; 322 case MAXIMUM: 323 return "Maximum"; 324 case MINIMUM: 325 return "Minimum"; 326 case COUNT: 327 return "Count"; 328 case TOTALCOUNT: 329 return "Total Count"; 330 case MEDIAN: 331 return "Median"; 332 case STDDEV: 333 return "Standard Deviation"; 334 case SUM: 335 return "Sum"; 336 case VARIANCE: 337 return "Variance"; 338 case _20PERCENT: 339 return "20th Percentile"; 340 case _80PERCENT: 341 return "80th Percentile"; 342 case _4LOWER: 343 return "Lower Quartile"; 344 case _4UPPER: 345 return "Upper Quartile"; 346 case _4DEV: 347 return "Quartile Deviation"; 348 case _51: 349 return "1st Quintile"; 350 case _52: 351 return "2nd Quintile"; 352 case _53: 353 return "3rd Quintile"; 354 case _54: 355 return "4th Quintile"; 356 case SKEW: 357 return "Skew"; 358 case KURTOSIS: 359 return "Kurtosis"; 360 case REGRESSION: 361 return "Regression"; 362 case NULL: 363 return null; 364 default: 365 return "?"; 366 } 367 } 368 369}