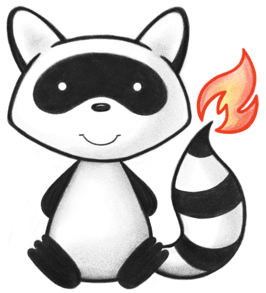
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ObservationStatus { 037 038 /** 039 * The existence of the observation is registered, but there is no result yet 040 * available. 041 */ 042 REGISTERED, 043 /** 044 * This is an initial or interim observation: data may be incomplete or 045 * unverified. 046 */ 047 PRELIMINARY, 048 /** 049 * The observation is complete and there are no further actions needed. 050 * Additional information such "released", "signed", etc would be represented 051 * using [Provenance](provenance.html) which provides not only the act but also 052 * the actors and dates and other related data. These act states would be 053 * associated with an observation status of `preliminary` until they are all 054 * completed and then a status of `final` would be applied. 055 */ 056 FINAL, 057 /** 058 * Subsequent to being Final, the observation has been modified subsequent. This 059 * includes updates/new information and corrections. 060 */ 061 AMENDED, 062 /** 063 * Subsequent to being Final, the observation has been modified to correct an 064 * error in the test result. 065 */ 066 CORRECTED, 067 /** 068 * The observation is unavailable because the measurement was not started or not 069 * completed (also sometimes called "aborted"). 070 */ 071 CANCELLED, 072 /** 073 * The observation has been withdrawn following previous final release. This 074 * electronic record should never have existed, though it is possible that 075 * real-world decisions were based on it. (If real-world activity has occurred, 076 * the status should be "cancelled" rather than "entered-in-error".). 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring/source system does not know which of the status values 081 * currently applies for this observation. Note: This concept is not to be used 082 * for "other" - one of the listed statuses is presumed to apply, but the 083 * authoring/source system does not know which. 084 */ 085 UNKNOWN, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 091 public static ObservationStatus fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("registered".equals(codeString)) 095 return REGISTERED; 096 if ("preliminary".equals(codeString)) 097 return PRELIMINARY; 098 if ("final".equals(codeString)) 099 return FINAL; 100 if ("amended".equals(codeString)) 101 return AMENDED; 102 if ("corrected".equals(codeString)) 103 return CORRECTED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("entered-in-error".equals(codeString)) 107 return ENTEREDINERROR; 108 if ("unknown".equals(codeString)) 109 return UNKNOWN; 110 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 111 } 112 113 public String toCode() { 114 switch (this) { 115 case REGISTERED: 116 return "registered"; 117 case PRELIMINARY: 118 return "preliminary"; 119 case FINAL: 120 return "final"; 121 case AMENDED: 122 return "amended"; 123 case CORRECTED: 124 return "corrected"; 125 case CANCELLED: 126 return "cancelled"; 127 case ENTEREDINERROR: 128 return "entered-in-error"; 129 case UNKNOWN: 130 return "unknown"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getSystem() { 139 return "http://hl7.org/fhir/observation-status"; 140 } 141 142 public String getDefinition() { 143 switch (this) { 144 case REGISTERED: 145 return "The existence of the observation is registered, but there is no result yet available."; 146 case PRELIMINARY: 147 return "This is an initial or interim observation: data may be incomplete or unverified."; 148 case FINAL: 149 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 150 case AMENDED: 151 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 152 case CORRECTED: 153 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 154 case CANCELLED: 155 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 156 case ENTEREDINERROR: 157 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 158 case UNKNOWN: 159 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDisplay() { 168 switch (this) { 169 case REGISTERED: 170 return "Registered"; 171 case PRELIMINARY: 172 return "Preliminary"; 173 case FINAL: 174 return "Final"; 175 case AMENDED: 176 return "Amended"; 177 case CORRECTED: 178 return "Corrected"; 179 case CANCELLED: 180 return "Cancelled"; 181 case ENTEREDINERROR: 182 return "Entered in Error"; 183 case UNKNOWN: 184 return "Unknown"; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 192}