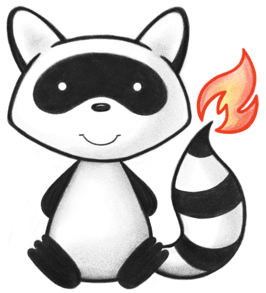
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum OrganizationType { 037 038 /** 039 * An organization that provides healthcare services. 040 */ 041 PROV, 042 /** 043 * A department or ward within a hospital (Generally is not applicable to top 044 * level organizations) 045 */ 046 DEPT, 047 /** 048 * An organizational team is usually a grouping of practitioners that perform a 049 * specific function within an organization (which could be a top level 050 * organization, or a department). 051 */ 052 TEAM, 053 /** 054 * A political body, often used when including organization records for 055 * government bodies such as a Federal Government, State or Local Government. 056 */ 057 GOVT, 058 /** 059 * A company that provides insurance to its subscribers that may include 060 * healthcare related policies. 061 */ 062 INS, 063 /** 064 * A company, charity, or governmental organization, which processes claims 065 * and/or issues payments to providers on behalf of patients or groups of 066 * patients. 067 */ 068 PAY, 069 /** 070 * An educational institution that provides education or research facilities. 071 */ 072 EDU, 073 /** 074 * An organization that is identified as a part of a religious institution. 075 */ 076 RELI, 077 /** 078 * An organization that is identified as a Pharmaceutical/Clinical Research 079 * Sponsor. 080 */ 081 CRS, 082 /** 083 * An un-incorporated community group. 084 */ 085 CG, 086 /** 087 * An organization that is a registered business or corporation but not 088 * identified by other types. 089 */ 090 BUS, 091 /** 092 * Other type of organization not already specified. 093 */ 094 OTHER, 095 /** 096 * added to help the parsers 097 */ 098 NULL; 099 100 public static OrganizationType fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("prov".equals(codeString)) 104 return PROV; 105 if ("dept".equals(codeString)) 106 return DEPT; 107 if ("team".equals(codeString)) 108 return TEAM; 109 if ("govt".equals(codeString)) 110 return GOVT; 111 if ("ins".equals(codeString)) 112 return INS; 113 if ("pay".equals(codeString)) 114 return PAY; 115 if ("edu".equals(codeString)) 116 return EDU; 117 if ("reli".equals(codeString)) 118 return RELI; 119 if ("crs".equals(codeString)) 120 return CRS; 121 if ("cg".equals(codeString)) 122 return CG; 123 if ("bus".equals(codeString)) 124 return BUS; 125 if ("other".equals(codeString)) 126 return OTHER; 127 throw new FHIRException("Unknown OrganizationType code '" + codeString + "'"); 128 } 129 130 public String toCode() { 131 switch (this) { 132 case PROV: 133 return "prov"; 134 case DEPT: 135 return "dept"; 136 case TEAM: 137 return "team"; 138 case GOVT: 139 return "govt"; 140 case INS: 141 return "ins"; 142 case PAY: 143 return "pay"; 144 case EDU: 145 return "edu"; 146 case RELI: 147 return "reli"; 148 case CRS: 149 return "crs"; 150 case CG: 151 return "cg"; 152 case BUS: 153 return "bus"; 154 case OTHER: 155 return "other"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 163 public String getSystem() { 164 return "http://terminology.hl7.org/CodeSystem/organization-type"; 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case PROV: 170 return "An organization that provides healthcare services."; 171 case DEPT: 172 return "A department or ward within a hospital (Generally is not applicable to top level organizations)"; 173 case TEAM: 174 return "An organizational team is usually a grouping of practitioners that perform a specific function within an organization (which could be a top level organization, or a department)."; 175 case GOVT: 176 return "A political body, often used when including organization records for government bodies such as a Federal Government, State or Local Government."; 177 case INS: 178 return "A company that provides insurance to its subscribers that may include healthcare related policies."; 179 case PAY: 180 return "A company, charity, or governmental organization, which processes claims and/or issues payments to providers on behalf of patients or groups of patients."; 181 case EDU: 182 return "An educational institution that provides education or research facilities."; 183 case RELI: 184 return "An organization that is identified as a part of a religious institution."; 185 case CRS: 186 return "An organization that is identified as a Pharmaceutical/Clinical Research Sponsor."; 187 case CG: 188 return "An un-incorporated community group."; 189 case BUS: 190 return "An organization that is a registered business or corporation but not identified by other types."; 191 case OTHER: 192 return "Other type of organization not already specified."; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 200 public String getDisplay() { 201 switch (this) { 202 case PROV: 203 return "Healthcare Provider"; 204 case DEPT: 205 return "Hospital Department"; 206 case TEAM: 207 return "Organizational team"; 208 case GOVT: 209 return "Government"; 210 case INS: 211 return "Insurance Company"; 212 case PAY: 213 return "Payer"; 214 case EDU: 215 return "Educational Institute"; 216 case RELI: 217 return "Religious Institution"; 218 case CRS: 219 return "Clinical Research Sponsor"; 220 case CG: 221 return "Community Group"; 222 case BUS: 223 return "Non-Healthcare Business or Corporation"; 224 case OTHER: 225 return "Other"; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 233}