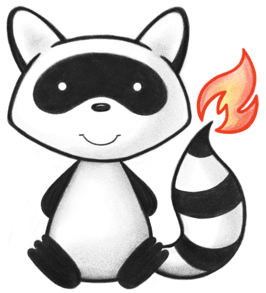
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ProvenanceAgentRole { 037 038 /** 039 * A person entering the data into the originating system 040 */ 041 ENTERER, 042 /** 043 * A person, animal, organization or device that who actually and principally 044 * carries out the activity 045 */ 046 PERFORMER, 047 /** 048 * A party that originates the resource and therefore has responsibility for the 049 * information given in the resource and ownership of this resource 050 */ 051 AUTHOR, 052 /** 053 * A person who verifies the correctness and appropriateness of activity 054 */ 055 VERIFIER, 056 /** 057 * The person authenticated the content and accepted legal responsibility for 058 * its content 059 */ 060 LEGAL, 061 /** 062 * A verifier who attests to the accuracy of the resource 063 */ 064 ATTESTER, 065 /** 066 * A person who reported information that contributed to the resource 067 */ 068 INFORMANT, 069 /** 070 * The entity that is accountable for maintaining a true an accurate copy of the 071 * original record 072 */ 073 CUSTODIAN, 074 /** 075 * A device that operates independently of an author on custodian's algorithms 076 * for data extraction of existing information for purpose of generating a new 077 * artifact. 078 */ 079 ASSEMBLER, 080 /** 081 * A device used by an author to record new information, which may also be used 082 * by the author to select existing information for aggregation with newly 083 * recorded information for the purpose of generating a new artifact. 084 */ 085 COMPOSER, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 091 public static ProvenanceAgentRole fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("enterer".equals(codeString)) 095 return ENTERER; 096 if ("performer".equals(codeString)) 097 return PERFORMER; 098 if ("author".equals(codeString)) 099 return AUTHOR; 100 if ("verifier".equals(codeString)) 101 return VERIFIER; 102 if ("legal".equals(codeString)) 103 return LEGAL; 104 if ("attester".equals(codeString)) 105 return ATTESTER; 106 if ("informant".equals(codeString)) 107 return INFORMANT; 108 if ("custodian".equals(codeString)) 109 return CUSTODIAN; 110 if ("assembler".equals(codeString)) 111 return ASSEMBLER; 112 if ("composer".equals(codeString)) 113 return COMPOSER; 114 throw new FHIRException("Unknown ProvenanceAgentRole code '" + codeString + "'"); 115 } 116 117 public String toCode() { 118 switch (this) { 119 case ENTERER: 120 return "enterer"; 121 case PERFORMER: 122 return "performer"; 123 case AUTHOR: 124 return "author"; 125 case VERIFIER: 126 return "verifier"; 127 case LEGAL: 128 return "legal"; 129 case ATTESTER: 130 return "attester"; 131 case INFORMANT: 132 return "informant"; 133 case CUSTODIAN: 134 return "custodian"; 135 case ASSEMBLER: 136 return "assembler"; 137 case COMPOSER: 138 return "composer"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getSystem() { 147 return "http://hl7.org/fhir/provenance-participant-role"; 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case ENTERER: 153 return "A person entering the data into the originating system"; 154 case PERFORMER: 155 return "A person, animal, organization or device that who actually and principally carries out the activity"; 156 case AUTHOR: 157 return "A party that originates the resource and therefore has responsibility for the information given in the resource and ownership of this resource"; 158 case VERIFIER: 159 return "A person who verifies the correctness and appropriateness of activity"; 160 case LEGAL: 161 return "The person authenticated the content and accepted legal responsibility for its content"; 162 case ATTESTER: 163 return "A verifier who attests to the accuracy of the resource"; 164 case INFORMANT: 165 return "A person who reported information that contributed to the resource"; 166 case CUSTODIAN: 167 return "The entity that is accountable for maintaining a true an accurate copy of the original record"; 168 case ASSEMBLER: 169 return "A device that operates independently of an author on custodian's algorithms for data extraction of existing information for purpose of generating a new artifact."; 170 case COMPOSER: 171 return "A device used by an author to record new information, which may also be used by the author to select existing information for aggregation with newly recorded information for the purpose of generating a new artifact."; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDisplay() { 180 switch (this) { 181 case ENTERER: 182 return "Enterer"; 183 case PERFORMER: 184 return "Performer"; 185 case AUTHOR: 186 return "Author"; 187 case VERIFIER: 188 return "Verifier"; 189 case LEGAL: 190 return "Legal Authenticator"; 191 case ATTESTER: 192 return "Attester"; 193 case INFORMANT: 194 return "Informant"; 195 case CUSTODIAN: 196 return "Custodian"; 197 case ASSEMBLER: 198 return "Assembler"; 199 case COMPOSER: 200 return "Composer"; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208}