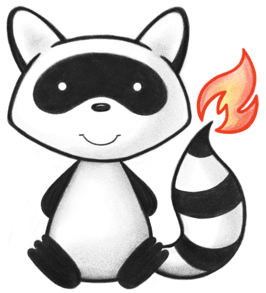
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum QuestionnaireItemControl { 037 038 /** 039 * UI controls relevant to organizing groups of questions 040 */ 041 GROUP, 042 /** 043 * Questions within the group should be listed sequentially 044 */ 045 LIST, 046 /** 047 * Questions within the group are rows in the table with possible answers as 048 * columns. Used for 'choice' questions. 049 */ 050 TABLE, 051 /** 052 * Questions within the group are columns in the table with possible answers as 053 * rows. Used for 'choice' questions. 054 */ 055 HTABLE, 056 /** 057 * Questions within the group are columns in the table with each group 058 * repetition as a row. Used for single-answer questions. 059 */ 060 GTABLE, 061 /** 062 * This table has one row - for the question. Permitted answers are columns. 063 * Used for choice questions. 064 */ 065 ATABLE, 066 /** 067 * The group is to be continuously visible at the top of the questionnaire 068 */ 069 HEADER, 070 /** 071 * The group is to be continuously visible at the bottom of the questionnaire 072 */ 073 FOOTER, 074 /** 075 * UI controls relevant to rendering questionnaire text items 076 */ 077 TEXT, 078 /** 079 * Text is displayed as a paragraph in a sequential position between sibling 080 * items (default behavior) 081 */ 082 INLINE, 083 /** 084 * Text is displayed immediately below or within the answer-entry area of the 085 * containing question item (typically as a guide for what to enter) 086 */ 087 PROMPT, 088 /** 089 * Text is displayed adjacent (horizontally or vertically) to the answer space 090 * for the parent question, typically to indicate a unit of measure 091 */ 092 UNIT, 093 /** 094 * Text is displayed to the left of the set of answer choices or a scaling 095 * control for the parent question item to indicate the meaning of the 'lower' 096 * bound. E.g. 'Strongly disagree' 097 */ 098 LOWER, 099 /** 100 * Text is displayed to the right of the set of answer choices or a scaling 101 * control for the parent question item to indicate the meaning of the 'upper' 102 * bound. E.g. 'Strongly agree' 103 */ 104 UPPER, 105 /** 106 * Text is temporarily visible over top of an item if the mouse is positioned 107 * over top of the text for the containing item 108 */ 109 FLYOVER, 110 /** 111 * Text is displayed in a dialog box or similar control if invoked by pushing a 112 * button or some other UI-appropriate action to request 'help' for a question, 113 * group or the questionnaire as a whole (depending what the text is nested 114 * within) 115 */ 116 HELP, 117 /** 118 * UI controls relevant to capturing question data 119 */ 120 QUESTION, 121 /** 122 * A control which provides a list of potential matches based on text entered 123 * into a control. Used for large choice sets where text-matching is an 124 * appropriate discovery mechanism. 125 */ 126 AUTOCOMPLETE, 127 /** 128 * A control where an item (or multiple items) can be selected from a list that 129 * is only displayed when the user is editing the field. 130 */ 131 DROPDOWN, 132 /** 133 * A control where choices are listed with a box beside them. The box can be 134 * toggled to select or de-select a given choice. Multiple selections may be 135 * possible. 136 */ 137 CHECKBOX, 138 /** 139 * A control where editing an item spawns a separate dialog box or screen 140 * permitting a user to navigate, filter or otherwise discover an appropriate 141 * match. Useful for large choice sets where text matching is not an appropriate 142 * discovery mechanism. Such screens must generally be tuned for the specific 143 * choice list structure. 144 */ 145 LOOKUP, 146 /** 147 * A control where choices are listed with a button beside them. The button can 148 * be toggled to select or de-select a given choice. Selecting one item 149 * deselects all others. 150 */ 151 RADIOBUTTON, 152 /** 153 * A control where an axis is displayed between the high and low values and the 154 * control can be visually manipulated to select a value anywhere on the axis. 155 */ 156 SLIDER, 157 /** 158 * A control where a list of numeric or other ordered values can be scrolled 159 * through via arrows. 160 */ 161 SPINNER, 162 /** 163 * A control where a user can type in their answer freely. 164 */ 165 TEXTBOX, 166 /** 167 * added to help the parsers 168 */ 169 NULL; 170 171 public static QuestionnaireItemControl fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("group".equals(codeString)) 175 return GROUP; 176 if ("list".equals(codeString)) 177 return LIST; 178 if ("table".equals(codeString)) 179 return TABLE; 180 if ("htable".equals(codeString)) 181 return HTABLE; 182 if ("gtable".equals(codeString)) 183 return GTABLE; 184 if ("atable".equals(codeString)) 185 return ATABLE; 186 if ("header".equals(codeString)) 187 return HEADER; 188 if ("footer".equals(codeString)) 189 return FOOTER; 190 if ("text".equals(codeString)) 191 return TEXT; 192 if ("inline".equals(codeString)) 193 return INLINE; 194 if ("prompt".equals(codeString)) 195 return PROMPT; 196 if ("unit".equals(codeString)) 197 return UNIT; 198 if ("lower".equals(codeString)) 199 return LOWER; 200 if ("upper".equals(codeString)) 201 return UPPER; 202 if ("flyover".equals(codeString)) 203 return FLYOVER; 204 if ("help".equals(codeString)) 205 return HELP; 206 if ("question".equals(codeString)) 207 return QUESTION; 208 if ("autocomplete".equals(codeString)) 209 return AUTOCOMPLETE; 210 if ("drop-down".equals(codeString)) 211 return DROPDOWN; 212 if ("check-box".equals(codeString)) 213 return CHECKBOX; 214 if ("lookup".equals(codeString)) 215 return LOOKUP; 216 if ("radio-button".equals(codeString)) 217 return RADIOBUTTON; 218 if ("slider".equals(codeString)) 219 return SLIDER; 220 if ("spinner".equals(codeString)) 221 return SPINNER; 222 if ("text-box".equals(codeString)) 223 return TEXTBOX; 224 throw new FHIRException("Unknown QuestionnaireItemControl code '" + codeString + "'"); 225 } 226 227 public String toCode() { 228 switch (this) { 229 case GROUP: 230 return "group"; 231 case LIST: 232 return "list"; 233 case TABLE: 234 return "table"; 235 case HTABLE: 236 return "htable"; 237 case GTABLE: 238 return "gtable"; 239 case ATABLE: 240 return "atable"; 241 case HEADER: 242 return "header"; 243 case FOOTER: 244 return "footer"; 245 case TEXT: 246 return "text"; 247 case INLINE: 248 return "inline"; 249 case PROMPT: 250 return "prompt"; 251 case UNIT: 252 return "unit"; 253 case LOWER: 254 return "lower"; 255 case UPPER: 256 return "upper"; 257 case FLYOVER: 258 return "flyover"; 259 case HELP: 260 return "help"; 261 case QUESTION: 262 return "question"; 263 case AUTOCOMPLETE: 264 return "autocomplete"; 265 case DROPDOWN: 266 return "drop-down"; 267 case CHECKBOX: 268 return "check-box"; 269 case LOOKUP: 270 return "lookup"; 271 case RADIOBUTTON: 272 return "radio-button"; 273 case SLIDER: 274 return "slider"; 275 case SPINNER: 276 return "spinner"; 277 case TEXTBOX: 278 return "text-box"; 279 case NULL: 280 return null; 281 default: 282 return "?"; 283 } 284 } 285 286 public String getSystem() { 287 return "http://hl7.org/fhir/questionnaire-item-control"; 288 } 289 290 public String getDefinition() { 291 switch (this) { 292 case GROUP: 293 return "UI controls relevant to organizing groups of questions"; 294 case LIST: 295 return "Questions within the group should be listed sequentially"; 296 case TABLE: 297 return "Questions within the group are rows in the table with possible answers as columns. Used for 'choice' questions."; 298 case HTABLE: 299 return "Questions within the group are columns in the table with possible answers as rows. Used for 'choice' questions."; 300 case GTABLE: 301 return "Questions within the group are columns in the table with each group repetition as a row. Used for single-answer questions."; 302 case ATABLE: 303 return "This table has one row - for the question. Permitted answers are columns. Used for choice questions."; 304 case HEADER: 305 return "The group is to be continuously visible at the top of the questionnaire"; 306 case FOOTER: 307 return "The group is to be continuously visible at the bottom of the questionnaire"; 308 case TEXT: 309 return "UI controls relevant to rendering questionnaire text items"; 310 case INLINE: 311 return "Text is displayed as a paragraph in a sequential position between sibling items (default behavior)"; 312 case PROMPT: 313 return "Text is displayed immediately below or within the answer-entry area of the containing question item (typically as a guide for what to enter)"; 314 case UNIT: 315 return "Text is displayed adjacent (horizontally or vertically) to the answer space for the parent question, typically to indicate a unit of measure"; 316 case LOWER: 317 return "Text is displayed to the left of the set of answer choices or a scaling control for the parent question item to indicate the meaning of the 'lower' bound. E.g. 'Strongly disagree'"; 318 case UPPER: 319 return "Text is displayed to the right of the set of answer choices or a scaling control for the parent question item to indicate the meaning of the 'upper' bound. E.g. 'Strongly agree'"; 320 case FLYOVER: 321 return "Text is temporarily visible over top of an item if the mouse is positioned over top of the text for the containing item"; 322 case HELP: 323 return "Text is displayed in a dialog box or similar control if invoked by pushing a button or some other UI-appropriate action to request 'help' for a question, group or the questionnaire as a whole (depending what the text is nested within)"; 324 case QUESTION: 325 return "UI controls relevant to capturing question data"; 326 case AUTOCOMPLETE: 327 return "A control which provides a list of potential matches based on text entered into a control. Used for large choice sets where text-matching is an appropriate discovery mechanism."; 328 case DROPDOWN: 329 return "A control where an item (or multiple items) can be selected from a list that is only displayed when the user is editing the field."; 330 case CHECKBOX: 331 return "A control where choices are listed with a box beside them. The box can be toggled to select or de-select a given choice. Multiple selections may be possible."; 332 case LOOKUP: 333 return "A control where editing an item spawns a separate dialog box or screen permitting a user to navigate, filter or otherwise discover an appropriate match. Useful for large choice sets where text matching is not an appropriate discovery mechanism. Such screens must generally be tuned for the specific choice list structure."; 334 case RADIOBUTTON: 335 return "A control where choices are listed with a button beside them. The button can be toggled to select or de-select a given choice. Selecting one item deselects all others."; 336 case SLIDER: 337 return "A control where an axis is displayed between the high and low values and the control can be visually manipulated to select a value anywhere on the axis."; 338 case SPINNER: 339 return "A control where a list of numeric or other ordered values can be scrolled through via arrows."; 340 case TEXTBOX: 341 return "A control where a user can type in their answer freely."; 342 case NULL: 343 return null; 344 default: 345 return "?"; 346 } 347 } 348 349 public String getDisplay() { 350 switch (this) { 351 case GROUP: 352 return "group"; 353 case LIST: 354 return "List"; 355 case TABLE: 356 return "Vertical Answer Table"; 357 case HTABLE: 358 return "Horizontal Answer Table"; 359 case GTABLE: 360 return "Group Table"; 361 case ATABLE: 362 return "Answer Table"; 363 case HEADER: 364 return "Header"; 365 case FOOTER: 366 return "Footer"; 367 case TEXT: 368 return "text"; 369 case INLINE: 370 return "In-line"; 371 case PROMPT: 372 return "Prompt"; 373 case UNIT: 374 return "Unit"; 375 case LOWER: 376 return "Lower-bound"; 377 case UPPER: 378 return "Upper-bound"; 379 case FLYOVER: 380 return "Fly-over"; 381 case HELP: 382 return "Help-Button"; 383 case QUESTION: 384 return "question"; 385 case AUTOCOMPLETE: 386 return "Auto-complete"; 387 case DROPDOWN: 388 return "Drop down"; 389 case CHECKBOX: 390 return "Check-box"; 391 case LOOKUP: 392 return "Lookup"; 393 case RADIOBUTTON: 394 return "Radio Button"; 395 case SLIDER: 396 return "Slider"; 397 case SPINNER: 398 return "Spinner"; 399 case TEXTBOX: 400 return "Text Box"; 401 case NULL: 402 return null; 403 default: 404 return "?"; 405 } 406 } 407 408}