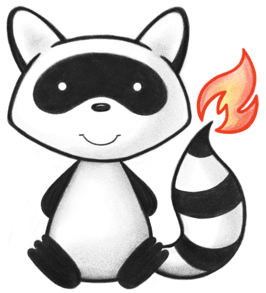
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum RelatedArtifactType { 037 038 /** 039 * Additional documentation for the knowledge resource. This would include 040 * additional instructions on usage as well as additional information on 041 * clinical context or appropriateness. 042 */ 043 DOCUMENTATION, 044 /** 045 * A summary of the justification for the knowledge resource including 046 * supporting evidence, relevant guidelines, or other clinically important 047 * information. This information is intended to provide a way to make the 048 * justification for the knowledge resource available to the consumer of 049 * interventions or results produced by the knowledge resource. 050 */ 051 JUSTIFICATION, 052 /** 053 * Bibliographic citation for papers, references, or other relevant material for 054 * the knowledge resource. This is intended to allow for citation of related 055 * material, but that was not necessarily specifically prepared in connection 056 * with this knowledge resource. 057 */ 058 CITATION, 059 /** 060 * The previous version of the knowledge resource. 061 */ 062 PREDECESSOR, 063 /** 064 * The next version of the knowledge resource. 065 */ 066 SUCCESSOR, 067 /** 068 * The knowledge resource is derived from the related artifact. This is intended 069 * to capture the relationship in which a particular knowledge resource is based 070 * on the content of another artifact, but is modified to capture either a 071 * different set of overall requirements, or a more specific set of requirements 072 * such as those involved in a particular institution or clinical setting. 073 */ 074 DERIVEDFROM, 075 /** 076 * The knowledge resource depends on the given related artifact. 077 */ 078 DEPENDSON, 079 /** 080 * The knowledge resource is composed of the given related artifact. 081 */ 082 COMPOSEDOF, 083 /** 084 * added to help the parsers 085 */ 086 NULL; 087 088 public static RelatedArtifactType fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("documentation".equals(codeString)) 092 return DOCUMENTATION; 093 if ("justification".equals(codeString)) 094 return JUSTIFICATION; 095 if ("citation".equals(codeString)) 096 return CITATION; 097 if ("predecessor".equals(codeString)) 098 return PREDECESSOR; 099 if ("successor".equals(codeString)) 100 return SUCCESSOR; 101 if ("derived-from".equals(codeString)) 102 return DERIVEDFROM; 103 if ("depends-on".equals(codeString)) 104 return DEPENDSON; 105 if ("composed-of".equals(codeString)) 106 return COMPOSEDOF; 107 throw new FHIRException("Unknown RelatedArtifactType code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case DOCUMENTATION: 113 return "documentation"; 114 case JUSTIFICATION: 115 return "justification"; 116 case CITATION: 117 return "citation"; 118 case PREDECESSOR: 119 return "predecessor"; 120 case SUCCESSOR: 121 return "successor"; 122 case DERIVEDFROM: 123 return "derived-from"; 124 case DEPENDSON: 125 return "depends-on"; 126 case COMPOSEDOF: 127 return "composed-of"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getSystem() { 136 return "http://hl7.org/fhir/related-artifact-type"; 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case DOCUMENTATION: 142 return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness."; 143 case JUSTIFICATION: 144 return "A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource."; 145 case CITATION: 146 return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 147 case PREDECESSOR: 148 return "The previous version of the knowledge resource."; 149 case SUCCESSOR: 150 return "The next version of the knowledge resource."; 151 case DERIVEDFROM: 152 return "The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting."; 153 case DEPENDSON: 154 return "The knowledge resource depends on the given related artifact."; 155 case COMPOSEDOF: 156 return "The knowledge resource is composed of the given related artifact."; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDisplay() { 165 switch (this) { 166 case DOCUMENTATION: 167 return "Documentation"; 168 case JUSTIFICATION: 169 return "Justification"; 170 case CITATION: 171 return "Citation"; 172 case PREDECESSOR: 173 return "Predecessor"; 174 case SUCCESSOR: 175 return "Successor"; 176 case DERIVEDFROM: 177 return "Derived From"; 178 case DEPENDSON: 179 return "Depends On"; 180 case COMPOSEDOF: 181 return "Composed Of"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 189}