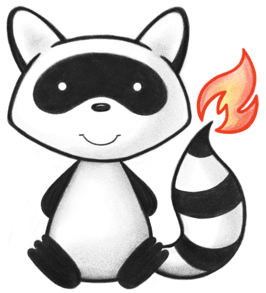
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum RequestIntent { 037 038 /** 039 * The request is a suggestion made by someone/something that does not have an 040 * intention to ensure it occurs and without providing an authorization to act. 041 */ 042 PROPOSAL, 043 /** 044 * The request represents an intention to ensure something occurs without 045 * providing an authorization for others to act. 046 */ 047 PLAN, 048 /** 049 * The request represents a legally binding instruction authored by a Patient or 050 * RelatedPerson. 051 */ 052 DIRECTIVE, 053 /** 054 * The request represents a request/demand and authorization for action by a 055 * Practitioner. 056 */ 057 ORDER, 058 /** 059 * The request represents an original authorization for action. 060 */ 061 ORIGINALORDER, 062 /** 063 * The request represents an automatically generated supplemental authorization 064 * for action based on a parent authorization together with initial results of 065 * the action taken against that parent authorization. 066 */ 067 REFLEXORDER, 068 /** 069 * The request represents the view of an authorization instantiated by a 070 * fulfilling system representing the details of the fulfiller's intention to 071 * act upon a submitted order. 072 */ 073 FILLERORDER, 074 /** 075 * An order created in fulfillment of a broader order that represents the 076 * authorization for a single activity occurrence. E.g. The administration of a 077 * single dose of a drug. 078 */ 079 INSTANCEORDER, 080 /** 081 * The request represents a component or option for a RequestGroup that 082 * establishes timing, conditionality and/or other constraints among a set of 083 * requests. Refer to [[[RequestGroup]]] for additional information on how this 084 * status is used. 085 */ 086 OPTION, 087 /** 088 * added to help the parsers 089 */ 090 NULL; 091 092 public static RequestIntent fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("proposal".equals(codeString)) 096 return PROPOSAL; 097 if ("plan".equals(codeString)) 098 return PLAN; 099 if ("directive".equals(codeString)) 100 return DIRECTIVE; 101 if ("order".equals(codeString)) 102 return ORDER; 103 if ("original-order".equals(codeString)) 104 return ORIGINALORDER; 105 if ("reflex-order".equals(codeString)) 106 return REFLEXORDER; 107 if ("filler-order".equals(codeString)) 108 return FILLERORDER; 109 if ("instance-order".equals(codeString)) 110 return INSTANCEORDER; 111 if ("option".equals(codeString)) 112 return OPTION; 113 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 114 } 115 116 public String toCode() { 117 switch (this) { 118 case PROPOSAL: 119 return "proposal"; 120 case PLAN: 121 return "plan"; 122 case DIRECTIVE: 123 return "directive"; 124 case ORDER: 125 return "order"; 126 case ORIGINALORDER: 127 return "original-order"; 128 case REFLEXORDER: 129 return "reflex-order"; 130 case FILLERORDER: 131 return "filler-order"; 132 case INSTANCEORDER: 133 return "instance-order"; 134 case OPTION: 135 return "option"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getSystem() { 144 return "http://hl7.org/fhir/request-intent"; 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case PROPOSAL: 150 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 151 case PLAN: 152 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 153 case DIRECTIVE: 154 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 155 case ORDER: 156 return "The request represents a request/demand and authorization for action by a Practitioner."; 157 case ORIGINALORDER: 158 return "The request represents an original authorization for action."; 159 case REFLEXORDER: 160 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 161 case FILLERORDER: 162 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 163 case INSTANCEORDER: 164 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 165 case OPTION: 166 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174 public String getDisplay() { 175 switch (this) { 176 case PROPOSAL: 177 return "Proposal"; 178 case PLAN: 179 return "Plan"; 180 case DIRECTIVE: 181 return "Directive"; 182 case ORDER: 183 return "Order"; 184 case ORIGINALORDER: 185 return "Original Order"; 186 case REFLEXORDER: 187 return "Reflex Order"; 188 case FILLERORDER: 189 return "Filler Order"; 190 case INSTANCEORDER: 191 return "Instance Order"; 192 case OPTION: 193 return "Option"; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 201}