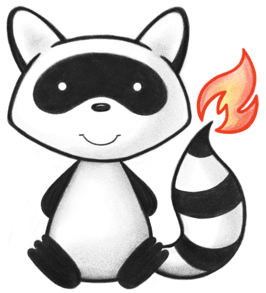
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ResourceSecurityCategory { 037 038 /** 039 * These resources tend to not contain any individual data, or business 040 * sensitive data. Most often these Resources will be available for anonymous 041 * access, meaning there is no access control based on the user or system 042 * requesting. However these Resources do tend to contain important information 043 * that must be authenticated back to the source publishing them, and protected 044 * from integrity failures in communication. For this reason server 045 * authenticated https (TLS) is recommended to provide authentication of the 046 * server and integrity protection in transit. This is normal web-server use of 047 * https. 048 */ 049 ANONYMOUS, 050 /** 051 * These Resources tend to not contain any individual data, but do have data 052 * that describe business or service sensitive data. The use of the term 053 * Business is not intended to only mean an incorporated business, but rather 054 * the more broad concept of an organization, location, or other group that is 055 * not identifable as individuals. Often these resources will require some for 056 * of client authentication to assure that only authorized access is given. The 057 * client access control may be to individuals, or may be to system identity. 058 * For this purpose possible client authentication methods such as: 059 * mutual-authenticated-TLS, APIKey, App signed JWT, or App OAuth client-id JWT 060 * For example: a App that uses a Business protected Provider Directory to 061 * determine other business endpoint details. 062 */ 063 BUSINESS, 064 /** 065 * These Resources do NOT contain Patient data, but do contain individual 066 * information about other participants. These other individuals are 067 * Practitioners, PractionerRole, CareTeam, or other users. These identities are 068 * needed to enable the practice of healthcare. These identities are identities 069 * under general privacy regulations, and thus must consider Privacy risk. Often 070 * access to these other identities are covered by business relationships. For 071 * this purpose access to these Resources will tend to be Role specific using 072 * methods such as RBAC or ABAC. 073 */ 074 INDIVIDUAL, 075 /** 076 * These Resources make up the bulk of FHIR and therefore are the most commonly 077 * understood. These Resources contain highly sesitive health information, or 078 * are closely linked to highly sensitive health information. These Resources 079 * will often use the security labels to differentiate various confidentiality 080 * levels within this broad group of Patient Sensitive data. Access to these 081 * Resources often requires a declared Purpose Of Use. Access to these Resources 082 * is often controlled by a Privacy Consent. 083 */ 084 PATIENT, 085 /** 086 * Some Resources can be used for a wide scope of use-cases that span very 087 * sensitive to very non-sensitive. These Resources do not fall into any of the 088 * above classifications, as their sensitivity is highly variable. These 089 * Resources will need special handling. These Resources often contain metadata 090 * that describes the content in a way that can be used for Access Control 091 * decisions. 092 */ 093 NOTCLASSIFIED, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static ResourceSecurityCategory fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("anonymous".equals(codeString)) 103 return ANONYMOUS; 104 if ("business".equals(codeString)) 105 return BUSINESS; 106 if ("individual".equals(codeString)) 107 return INDIVIDUAL; 108 if ("patient".equals(codeString)) 109 return PATIENT; 110 if ("not-classified".equals(codeString)) 111 return NOTCLASSIFIED; 112 throw new FHIRException("Unknown ResourceSecurityCategory code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case ANONYMOUS: 118 return "anonymous"; 119 case BUSINESS: 120 return "business"; 121 case INDIVIDUAL: 122 return "individual"; 123 case PATIENT: 124 return "patient"; 125 case NOTCLASSIFIED: 126 return "not-classified"; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getSystem() { 135 return "http://terminology.hl7.org/CodeSystem/resource-security-category"; 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case ANONYMOUS: 141 return "These resources tend to not contain any individual data, or business sensitive data. Most often these Resources will be available for anonymous access, meaning there is no access control based on the user or system requesting. However these Resources do tend to contain important information that must be authenticated back to the source publishing them, and protected from integrity failures in communication. For this reason server authenticated https (TLS) is recommended to provide authentication of the server and integrity protection in transit. This is normal web-server use of https."; 142 case BUSINESS: 143 return "These Resources tend to not contain any individual data, but do have data that describe business or service sensitive data. The use of the term Business is not intended to only mean an incorporated business, but rather the more broad concept of an organization, location, or other group that is not identifable as individuals. Often these resources will require some for of client authentication to assure that only authorized access is given. The client access control may be to individuals, or may be to system identity. For this purpose possible client authentication methods such as: mutual-authenticated-TLS, APIKey, App signed JWT, or App OAuth client-id JWT For example: a App that uses a Business protected Provider Directory to determine other business endpoint details."; 144 case INDIVIDUAL: 145 return "These Resources do NOT contain Patient data, but do contain individual information about other participants. These other individuals are Practitioners, PractionerRole, CareTeam, or other users. These identities are needed to enable the practice of healthcare. These identities are identities under general privacy regulations, and thus must consider Privacy risk. Often access to these other identities are covered by business relationships. For this purpose access to these Resources will tend to be Role specific using methods such as RBAC or ABAC."; 146 case PATIENT: 147 return "These Resources make up the bulk of FHIR and therefore are the most commonly understood. These Resources contain highly sesitive health information, or are closely linked to highly sensitive health information. These Resources will often use the security labels to differentiate various confidentiality levels within this broad group of Patient Sensitive data. Access to these Resources often requires a declared Purpose Of Use. Access to these Resources is often controlled by a Privacy Consent."; 148 case NOTCLASSIFIED: 149 return "Some Resources can be used for a wide scope of use-cases that span very sensitive to very non-sensitive. These Resources do not fall into any of the above classifications, as their sensitivity is highly variable. These Resources will need special handling. These Resources often contain metadata that describes the content in a way that can be used for Access Control decisions."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case ANONYMOUS: 160 return "Anonymous READ Access Resource"; 161 case BUSINESS: 162 return "Business Sensitive Resource"; 163 case INDIVIDUAL: 164 return "Individual Sensitive Resource"; 165 case PATIENT: 166 return "Patient Sensitive"; 167 case NOTCLASSIFIED: 168 return "Not classified"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176}