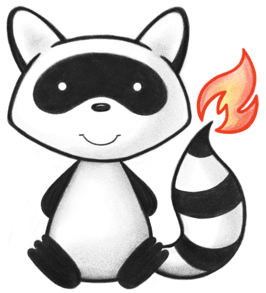
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ResourceStatus { 037 038 /** 039 * The resource was created in error, and should not be treated as valid (note: 040 * in many cases, for various data integrity related reasons, the information 041 * cannot be removed from the record) 042 */ 043 ERROR, 044 /** 045 * The resource describes an action or plan that is proposed, and not yet 046 * approved by the participants 047 */ 048 PROPOSED, 049 /** 050 * The resource describes a course of action that is planned and 051 * agreed/approved, but at the time of recording was still future 052 */ 053 PLANNED, 054 /** 055 * The information in the resource is still being prepared and edited 056 */ 057 DRAFT, 058 /** 059 * A fulfiller has been asked to perform this action, but it has not yet 060 * occurred 061 */ 062 REQUESTED, 063 /** 064 * The fulfiller has received the request, but not yet agreed to carry out the 065 * action 066 */ 067 RECEIVED, 068 /** 069 * The fulfiller chose not to perform the action 070 */ 071 DECLINED, 072 /** 073 * The fulfiller has decided to perform the action, and plans are in train to do 074 * this in the future 075 */ 076 ACCEPTED, 077 /** 078 * The pre-conditions for the action are all fulfilled, and it is imminent 079 */ 080 ARRIVED, 081 /** 082 * The resource describes information that is currently valid or a process that 083 * is presently occuring 084 */ 085 ACTIVE, 086 /** 087 * The process described/requested in this resource has been halted for some 088 * reason 089 */ 090 SUSPENDED, 091 /** 092 * The process described/requested in the resource could not be completed, and 093 * no further action is planned 094 */ 095 FAILED, 096 /** 097 * The information in this resource has been replaced by information in another 098 * resource 099 */ 100 REPLACED, 101 /** 102 * The process described/requested in the resource has been completed, and no 103 * further action is planned 104 */ 105 COMPLETE, 106 /** 107 * The resource describes information that is no longer valid or a process that 108 * is stopped occurring 109 */ 110 INACTIVE, 111 /** 112 * The process described/requested in the resource did not complete - usually 113 * due to some workflow error, and no further action is planned 114 */ 115 ABANDONED, 116 /** 117 * Authoring system does not know the status 118 */ 119 UNKNOWN, 120 /** 121 * The information in this resource is not yet approved 122 */ 123 UNCONFIRMED, 124 /** 125 * The information in this resource is approved 126 */ 127 CONFIRMED, 128 /** 129 * The issue identified by this resource is no longer of concern 130 */ 131 RESOLVED, 132 /** 133 * This information has been ruled out by testing and evaluation 134 */ 135 REFUTED, 136 /** 137 * Potentially true? 138 */ 139 DIFFERENTIAL, 140 /** 141 * This information is still being assembled 142 */ 143 PARTIAL, 144 /** 145 * not available at this time/location 146 */ 147 BUSYUNAVAILABLE, 148 /** 149 * Free for scheduling 150 */ 151 FREE, 152 /** 153 * Ready to act 154 */ 155 ONTARGET, 156 /** 157 * Ahead of the planned timelines 158 */ 159 AHEADOFTARGET, 160 /** 161 * 162 */ 163 BEHINDTARGET, 164 /** 165 * Behind the planned timelines 166 */ 167 NOTREADY, 168 /** 169 * The device transducer is disconnected 170 */ 171 TRANSDUCDISCON, 172 /** 173 * The hardware is disconnected 174 */ 175 HWDISCON, 176 /** 177 * added to help the parsers 178 */ 179 NULL; 180 181 public static ResourceStatus fromCode(String codeString) throws FHIRException { 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("error".equals(codeString)) 185 return ERROR; 186 if ("proposed".equals(codeString)) 187 return PROPOSED; 188 if ("planned".equals(codeString)) 189 return PLANNED; 190 if ("draft".equals(codeString)) 191 return DRAFT; 192 if ("requested".equals(codeString)) 193 return REQUESTED; 194 if ("received".equals(codeString)) 195 return RECEIVED; 196 if ("declined".equals(codeString)) 197 return DECLINED; 198 if ("accepted".equals(codeString)) 199 return ACCEPTED; 200 if ("arrived".equals(codeString)) 201 return ARRIVED; 202 if ("active".equals(codeString)) 203 return ACTIVE; 204 if ("suspended".equals(codeString)) 205 return SUSPENDED; 206 if ("failed".equals(codeString)) 207 return FAILED; 208 if ("replaced".equals(codeString)) 209 return REPLACED; 210 if ("complete".equals(codeString)) 211 return COMPLETE; 212 if ("inactive".equals(codeString)) 213 return INACTIVE; 214 if ("abandoned".equals(codeString)) 215 return ABANDONED; 216 if ("unknown".equals(codeString)) 217 return UNKNOWN; 218 if ("unconfirmed".equals(codeString)) 219 return UNCONFIRMED; 220 if ("confirmed".equals(codeString)) 221 return CONFIRMED; 222 if ("resolved".equals(codeString)) 223 return RESOLVED; 224 if ("refuted".equals(codeString)) 225 return REFUTED; 226 if ("differential".equals(codeString)) 227 return DIFFERENTIAL; 228 if ("partial".equals(codeString)) 229 return PARTIAL; 230 if ("busy-unavailable".equals(codeString)) 231 return BUSYUNAVAILABLE; 232 if ("free".equals(codeString)) 233 return FREE; 234 if ("on-target".equals(codeString)) 235 return ONTARGET; 236 if ("ahead-of-target".equals(codeString)) 237 return AHEADOFTARGET; 238 if ("behind-target".equals(codeString)) 239 return BEHINDTARGET; 240 if ("not-ready".equals(codeString)) 241 return NOTREADY; 242 if ("transduc-discon".equals(codeString)) 243 return TRANSDUCDISCON; 244 if ("hw-discon".equals(codeString)) 245 return HWDISCON; 246 throw new FHIRException("Unknown ResourceStatus code '" + codeString + "'"); 247 } 248 249 public String toCode() { 250 switch (this) { 251 case ERROR: 252 return "error"; 253 case PROPOSED: 254 return "proposed"; 255 case PLANNED: 256 return "planned"; 257 case DRAFT: 258 return "draft"; 259 case REQUESTED: 260 return "requested"; 261 case RECEIVED: 262 return "received"; 263 case DECLINED: 264 return "declined"; 265 case ACCEPTED: 266 return "accepted"; 267 case ARRIVED: 268 return "arrived"; 269 case ACTIVE: 270 return "active"; 271 case SUSPENDED: 272 return "suspended"; 273 case FAILED: 274 return "failed"; 275 case REPLACED: 276 return "replaced"; 277 case COMPLETE: 278 return "complete"; 279 case INACTIVE: 280 return "inactive"; 281 case ABANDONED: 282 return "abandoned"; 283 case UNKNOWN: 284 return "unknown"; 285 case UNCONFIRMED: 286 return "unconfirmed"; 287 case CONFIRMED: 288 return "confirmed"; 289 case RESOLVED: 290 return "resolved"; 291 case REFUTED: 292 return "refuted"; 293 case DIFFERENTIAL: 294 return "differential"; 295 case PARTIAL: 296 return "partial"; 297 case BUSYUNAVAILABLE: 298 return "busy-unavailable"; 299 case FREE: 300 return "free"; 301 case ONTARGET: 302 return "on-target"; 303 case AHEADOFTARGET: 304 return "ahead-of-target"; 305 case BEHINDTARGET: 306 return "behind-target"; 307 case NOTREADY: 308 return "not-ready"; 309 case TRANSDUCDISCON: 310 return "transduc-discon"; 311 case HWDISCON: 312 return "hw-discon"; 313 case NULL: 314 return null; 315 default: 316 return "?"; 317 } 318 } 319 320 public String getSystem() { 321 return "http://hl7.org/fhir/resource-status"; 322 } 323 324 public String getDefinition() { 325 switch (this) { 326 case ERROR: 327 return "The resource was created in error, and should not be treated as valid (note: in many cases, for various data integrity related reasons, the information cannot be removed from the record)"; 328 case PROPOSED: 329 return "The resource describes an action or plan that is proposed, and not yet approved by the participants"; 330 case PLANNED: 331 return "The resource describes a course of action that is planned and agreed/approved, but at the time of recording was still future"; 332 case DRAFT: 333 return "The information in the resource is still being prepared and edited"; 334 case REQUESTED: 335 return "A fulfiller has been asked to perform this action, but it has not yet occurred"; 336 case RECEIVED: 337 return "The fulfiller has received the request, but not yet agreed to carry out the action"; 338 case DECLINED: 339 return "The fulfiller chose not to perform the action"; 340 case ACCEPTED: 341 return "The fulfiller has decided to perform the action, and plans are in train to do this in the future"; 342 case ARRIVED: 343 return "The pre-conditions for the action are all fulfilled, and it is imminent"; 344 case ACTIVE: 345 return "The resource describes information that is currently valid or a process that is presently occuring"; 346 case SUSPENDED: 347 return "The process described/requested in this resource has been halted for some reason"; 348 case FAILED: 349 return "The process described/requested in the resource could not be completed, and no further action is planned"; 350 case REPLACED: 351 return "The information in this resource has been replaced by information in another resource"; 352 case COMPLETE: 353 return "The process described/requested in the resource has been completed, and no further action is planned"; 354 case INACTIVE: 355 return "The resource describes information that is no longer valid or a process that is stopped occurring"; 356 case ABANDONED: 357 return "The process described/requested in the resource did not complete - usually due to some workflow error, and no further action is planned"; 358 case UNKNOWN: 359 return "Authoring system does not know the status"; 360 case UNCONFIRMED: 361 return "The information in this resource is not yet approved"; 362 case CONFIRMED: 363 return "The information in this resource is approved"; 364 case RESOLVED: 365 return "The issue identified by this resource is no longer of concern"; 366 case REFUTED: 367 return "This information has been ruled out by testing and evaluation"; 368 case DIFFERENTIAL: 369 return "Potentially true?"; 370 case PARTIAL: 371 return "This information is still being assembled"; 372 case BUSYUNAVAILABLE: 373 return "not available at this time/location"; 374 case FREE: 375 return "Free for scheduling"; 376 case ONTARGET: 377 return "Ready to act"; 378 case AHEADOFTARGET: 379 return "Ahead of the planned timelines"; 380 case BEHINDTARGET: 381 return ""; 382 case NOTREADY: 383 return "Behind the planned timelines"; 384 case TRANSDUCDISCON: 385 return "The device transducer is disconnected"; 386 case HWDISCON: 387 return "The hardware is disconnected"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getDisplay() { 396 switch (this) { 397 case ERROR: 398 return "error"; 399 case PROPOSED: 400 return "proposed"; 401 case PLANNED: 402 return "planned"; 403 case DRAFT: 404 return "draft"; 405 case REQUESTED: 406 return "requested"; 407 case RECEIVED: 408 return "received"; 409 case DECLINED: 410 return "declined"; 411 case ACCEPTED: 412 return "accepted"; 413 case ARRIVED: 414 return "arrived"; 415 case ACTIVE: 416 return "active"; 417 case SUSPENDED: 418 return "suspended"; 419 case FAILED: 420 return "failed"; 421 case REPLACED: 422 return "replaced"; 423 case COMPLETE: 424 return "complete"; 425 case INACTIVE: 426 return "inactive"; 427 case ABANDONED: 428 return "abandoned"; 429 case UNKNOWN: 430 return "unknown"; 431 case UNCONFIRMED: 432 return "unconfirmed"; 433 case CONFIRMED: 434 return "confirmed"; 435 case RESOLVED: 436 return "resolved"; 437 case REFUTED: 438 return "refuted"; 439 case DIFFERENTIAL: 440 return "differential"; 441 case PARTIAL: 442 return "partial"; 443 case BUSYUNAVAILABLE: 444 return "busy-unavailable"; 445 case FREE: 446 return "free"; 447 case ONTARGET: 448 return "on-target"; 449 case AHEADOFTARGET: 450 return "ahead-of-target"; 451 case BEHINDTARGET: 452 return "behind-target"; 453 case NOTREADY: 454 return "not-ready"; 455 case TRANSDUCDISCON: 456 return "transduc-discon"; 457 case HWDISCON: 458 return "hw-discon"; 459 case NULL: 460 return null; 461 default: 462 return "?"; 463 } 464 } 465 466}