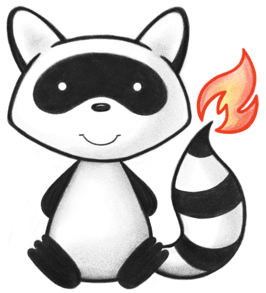
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ServiceCategory { 037 038 /** 039 * Adoption 040 */ 041 _1, 042 /** 043 * Aged Care 044 */ 045 _2, 046 /** 047 * Allied Health 048 */ 049 _34, 050 /** 051 * Alternative & Complementary Therapies 052 */ 053 _3, 054 /** 055 * Child Care and/or Kindergarten 056 */ 057 _4, 058 /** 059 * Child Development 060 */ 061 _5, 062 /** 063 * Child Protection & Family Services 064 */ 065 _6, 066 /** 067 * Community Health Care 068 */ 069 _7, 070 /** 071 * Counselling 072 */ 073 _8, 074 /** 075 * Crisis Line (GPAH use only) 076 */ 077 _36, 078 /** 079 * Death Services 080 */ 081 _9, 082 /** 083 * Dental 084 */ 085 _10, 086 /** 087 * Disability Support 088 */ 089 _11, 090 /** 091 * Drug/Alcohol 092 */ 093 _12, 094 /** 095 * Education & Learning 096 */ 097 _13, 098 /** 099 * Emergency Department 100 */ 101 _14, 102 /** 103 * Employment 104 */ 105 _15, 106 /** 107 * Financial & Material aid 108 */ 109 _16, 110 /** 111 * General Practice/GP (doctor) 112 */ 113 _17, 114 /** 115 * Hospital 116 */ 117 _35, 118 /** 119 * Housing/Homelessness 120 */ 121 _18, 122 /** 123 * Interpreting 124 */ 125 _19, 126 /** 127 * Justice 128 */ 129 _20, 130 /** 131 * Legal 132 */ 133 _21, 134 /** 135 * Mental Health 136 */ 137 _22, 138 /** 139 * NDIA 140 */ 141 _38, 142 /** 143 * Physical Activity & Recreation 144 */ 145 _23, 146 /** 147 * Regulation 148 */ 149 _24, 150 /** 151 * Respite/Carer Support 152 */ 153 _25, 154 /** 155 * Specialist Clinical Pathology - requires referral 156 */ 157 _26, 158 /** 159 * Specialist Medical - requires referral 160 */ 161 _27, 162 /** 163 * Specialist Obstetrics & Gynecology - requires referral 164 */ 165 _28, 166 /** 167 * Specialist Paediatric - requires referral 168 */ 169 _29, 170 /** 171 * Specialist Radiology/Imaging - requires referral 172 */ 173 _30, 174 /** 175 * Specialist Surgical - requires referral 176 */ 177 _31, 178 /** 179 * Support group/s 180 */ 181 _32, 182 /** 183 * Test Message (HSD admin use only) 184 */ 185 _37, 186 /** 187 * Transport 188 */ 189 _33, 190 /** 191 * added to help the parsers 192 */ 193 NULL; 194 195 public static ServiceCategory fromCode(String codeString) throws FHIRException { 196 if (codeString == null || "".equals(codeString)) 197 return null; 198 if ("1".equals(codeString)) 199 return _1; 200 if ("2".equals(codeString)) 201 return _2; 202 if ("34".equals(codeString)) 203 return _34; 204 if ("3".equals(codeString)) 205 return _3; 206 if ("4".equals(codeString)) 207 return _4; 208 if ("5".equals(codeString)) 209 return _5; 210 if ("6".equals(codeString)) 211 return _6; 212 if ("7".equals(codeString)) 213 return _7; 214 if ("8".equals(codeString)) 215 return _8; 216 if ("36".equals(codeString)) 217 return _36; 218 if ("9".equals(codeString)) 219 return _9; 220 if ("10".equals(codeString)) 221 return _10; 222 if ("11".equals(codeString)) 223 return _11; 224 if ("12".equals(codeString)) 225 return _12; 226 if ("13".equals(codeString)) 227 return _13; 228 if ("14".equals(codeString)) 229 return _14; 230 if ("15".equals(codeString)) 231 return _15; 232 if ("16".equals(codeString)) 233 return _16; 234 if ("17".equals(codeString)) 235 return _17; 236 if ("35".equals(codeString)) 237 return _35; 238 if ("18".equals(codeString)) 239 return _18; 240 if ("19".equals(codeString)) 241 return _19; 242 if ("20".equals(codeString)) 243 return _20; 244 if ("21".equals(codeString)) 245 return _21; 246 if ("22".equals(codeString)) 247 return _22; 248 if ("38".equals(codeString)) 249 return _38; 250 if ("23".equals(codeString)) 251 return _23; 252 if ("24".equals(codeString)) 253 return _24; 254 if ("25".equals(codeString)) 255 return _25; 256 if ("26".equals(codeString)) 257 return _26; 258 if ("27".equals(codeString)) 259 return _27; 260 if ("28".equals(codeString)) 261 return _28; 262 if ("29".equals(codeString)) 263 return _29; 264 if ("30".equals(codeString)) 265 return _30; 266 if ("31".equals(codeString)) 267 return _31; 268 if ("32".equals(codeString)) 269 return _32; 270 if ("37".equals(codeString)) 271 return _37; 272 if ("33".equals(codeString)) 273 return _33; 274 throw new FHIRException("Unknown ServiceCategory code '" + codeString + "'"); 275 } 276 277 public String toCode() { 278 switch (this) { 279 case _1: 280 return "1"; 281 case _2: 282 return "2"; 283 case _34: 284 return "34"; 285 case _3: 286 return "3"; 287 case _4: 288 return "4"; 289 case _5: 290 return "5"; 291 case _6: 292 return "6"; 293 case _7: 294 return "7"; 295 case _8: 296 return "8"; 297 case _36: 298 return "36"; 299 case _9: 300 return "9"; 301 case _10: 302 return "10"; 303 case _11: 304 return "11"; 305 case _12: 306 return "12"; 307 case _13: 308 return "13"; 309 case _14: 310 return "14"; 311 case _15: 312 return "15"; 313 case _16: 314 return "16"; 315 case _17: 316 return "17"; 317 case _35: 318 return "35"; 319 case _18: 320 return "18"; 321 case _19: 322 return "19"; 323 case _20: 324 return "20"; 325 case _21: 326 return "21"; 327 case _22: 328 return "22"; 329 case _38: 330 return "38"; 331 case _23: 332 return "23"; 333 case _24: 334 return "24"; 335 case _25: 336 return "25"; 337 case _26: 338 return "26"; 339 case _27: 340 return "27"; 341 case _28: 342 return "28"; 343 case _29: 344 return "29"; 345 case _30: 346 return "30"; 347 case _31: 348 return "31"; 349 case _32: 350 return "32"; 351 case _37: 352 return "37"; 353 case _33: 354 return "33"; 355 case NULL: 356 return null; 357 default: 358 return "?"; 359 } 360 } 361 362 public String getSystem() { 363 return "http://terminology.hl7.org/CodeSystem/service-category"; 364 } 365 366 public String getDefinition() { 367 switch (this) { 368 case _1: 369 return "Adoption"; 370 case _2: 371 return "Aged Care"; 372 case _34: 373 return "Allied Health"; 374 case _3: 375 return "Alternative & Complementary Therapies"; 376 case _4: 377 return "Child Care and/or Kindergarten"; 378 case _5: 379 return "Child Development"; 380 case _6: 381 return "Child Protection & Family Services"; 382 case _7: 383 return "Community Health Care"; 384 case _8: 385 return "Counselling"; 386 case _36: 387 return "Crisis Line (GPAH use only)"; 388 case _9: 389 return "Death Services"; 390 case _10: 391 return "Dental"; 392 case _11: 393 return "Disability Support"; 394 case _12: 395 return "Drug/Alcohol"; 396 case _13: 397 return "Education & Learning"; 398 case _14: 399 return "Emergency Department"; 400 case _15: 401 return "Employment"; 402 case _16: 403 return "Financial & Material aid"; 404 case _17: 405 return "General Practice/GP (doctor)"; 406 case _35: 407 return "Hospital"; 408 case _18: 409 return "Housing/Homelessness"; 410 case _19: 411 return "Interpreting"; 412 case _20: 413 return "Justice"; 414 case _21: 415 return "Legal"; 416 case _22: 417 return "Mental Health"; 418 case _38: 419 return "NDIA"; 420 case _23: 421 return "Physical Activity & Recreation"; 422 case _24: 423 return "Regulation"; 424 case _25: 425 return "Respite/Carer Support"; 426 case _26: 427 return "Specialist Clinical Pathology - requires referral"; 428 case _27: 429 return "Specialist Medical - requires referral"; 430 case _28: 431 return "Specialist Obstetrics & Gynecology - requires referral"; 432 case _29: 433 return "Specialist Paediatric - requires referral"; 434 case _30: 435 return "Specialist Radiology/Imaging - requires referral"; 436 case _31: 437 return "Specialist Surgical - requires referral"; 438 case _32: 439 return "Support group/s"; 440 case _37: 441 return "Test Message (HSD admin use only)"; 442 case _33: 443 return "Transport"; 444 case NULL: 445 return null; 446 default: 447 return "?"; 448 } 449 } 450 451 public String getDisplay() { 452 switch (this) { 453 case _1: 454 return "Adoption"; 455 case _2: 456 return "Aged Care"; 457 case _34: 458 return "Allied Health"; 459 case _3: 460 return "Alternative/Complementary Therapies"; 461 case _4: 462 return "Child Care /Kindergarten"; 463 case _5: 464 return "Child Development"; 465 case _6: 466 return "Child Protection & Family Services"; 467 case _7: 468 return "Community Health Care"; 469 case _8: 470 return "Counselling"; 471 case _36: 472 return "Crisis Line (GPAH use only)"; 473 case _9: 474 return "Death Services"; 475 case _10: 476 return "Dental"; 477 case _11: 478 return "Disability Support"; 479 case _12: 480 return "Drug/Alcohol"; 481 case _13: 482 return "Education & Learning"; 483 case _14: 484 return "Emergency Department"; 485 case _15: 486 return "Employment"; 487 case _16: 488 return "Financial & Material Aid"; 489 case _17: 490 return "General Practice"; 491 case _35: 492 return "Hospital"; 493 case _18: 494 return "Housing/Homelessness"; 495 case _19: 496 return "Interpreting"; 497 case _20: 498 return "Justice"; 499 case _21: 500 return "Legal"; 501 case _22: 502 return "Mental Health"; 503 case _38: 504 return "NDIA"; 505 case _23: 506 return "Physical Activity & Recreation"; 507 case _24: 508 return "Regulation"; 509 case _25: 510 return "Respite/Carer Support"; 511 case _26: 512 return "Specialist Clinical Pathology"; 513 case _27: 514 return "Specialist Medical"; 515 case _28: 516 return "Specialist Obstetrics & Gynecology"; 517 case _29: 518 return "Specialist Paediatric"; 519 case _30: 520 return "Specialist Radiology/Imaging"; 521 case _31: 522 return "Specialist Surgical"; 523 case _32: 524 return "Support Group/s"; 525 case _37: 526 return "Test Message (HSD admin)"; 527 case _33: 528 return "Transport"; 529 case NULL: 530 return null; 531 default: 532 return "?"; 533 } 534 } 535 536}