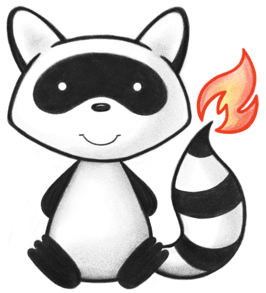
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ServiceUscls { 037 038 /** 039 * Exam, comp, primary 040 */ 041 _1101, 042 /** 043 * Exam, comp, mixed 044 */ 045 _1102, 046 /** 047 * Exam, comp, permanent 048 */ 049 _1103, 050 /** 051 * Exam, recall 052 */ 053 _1201, 054 /** 055 * Exam, emergency 056 */ 057 _1205, 058 /** 059 * Radiograph, series (12) 060 */ 061 _2101, 062 /** 063 * Radiograph, series (16) 064 */ 065 _2102, 066 /** 067 * Radiograph, bitewing 068 */ 069 _2141, 070 /** 071 * Radiograph, panoramic 072 */ 073 _2601, 074 /** 075 * Polishing, 1 unit 076 */ 077 _11101, 078 /** 079 * Polishing, 2 unit 080 */ 081 _11102, 082 /** 083 * Polishing, 3 unit 084 */ 085 _11103, 086 /** 087 * Polishing, 4 unit 088 */ 089 _11104, 090 /** 091 * Amalgam, 1 surface 092 */ 093 _21211, 094 /** 095 * Amalgam, 2 surface 096 */ 097 _21212, 098 /** 099 * Crown, PFM 100 */ 101 _27211, 102 /** 103 * Maryland Bridge 104 */ 105 _67211, 106 /** 107 * Lab, commercial 108 */ 109 _99111, 110 /** 111 * Lab, in office 112 */ 113 _99333, 114 /** 115 * Expense 116 */ 117 _99555, 118 /** 119 * added to help the parsers 120 */ 121 NULL; 122 123 public static ServiceUscls fromCode(String codeString) throws FHIRException { 124 if (codeString == null || "".equals(codeString)) 125 return null; 126 if ("1101".equals(codeString)) 127 return _1101; 128 if ("1102".equals(codeString)) 129 return _1102; 130 if ("1103".equals(codeString)) 131 return _1103; 132 if ("1201".equals(codeString)) 133 return _1201; 134 if ("1205".equals(codeString)) 135 return _1205; 136 if ("2101".equals(codeString)) 137 return _2101; 138 if ("2102".equals(codeString)) 139 return _2102; 140 if ("2141".equals(codeString)) 141 return _2141; 142 if ("2601".equals(codeString)) 143 return _2601; 144 if ("11101".equals(codeString)) 145 return _11101; 146 if ("11102".equals(codeString)) 147 return _11102; 148 if ("11103".equals(codeString)) 149 return _11103; 150 if ("11104".equals(codeString)) 151 return _11104; 152 if ("21211".equals(codeString)) 153 return _21211; 154 if ("21212".equals(codeString)) 155 return _21212; 156 if ("27211".equals(codeString)) 157 return _27211; 158 if ("67211".equals(codeString)) 159 return _67211; 160 if ("99111".equals(codeString)) 161 return _99111; 162 if ("99333".equals(codeString)) 163 return _99333; 164 if ("99555".equals(codeString)) 165 return _99555; 166 throw new FHIRException("Unknown ServiceUscls code '" + codeString + "'"); 167 } 168 169 public String toCode() { 170 switch (this) { 171 case _1101: 172 return "1101"; 173 case _1102: 174 return "1102"; 175 case _1103: 176 return "1103"; 177 case _1201: 178 return "1201"; 179 case _1205: 180 return "1205"; 181 case _2101: 182 return "2101"; 183 case _2102: 184 return "2102"; 185 case _2141: 186 return "2141"; 187 case _2601: 188 return "2601"; 189 case _11101: 190 return "11101"; 191 case _11102: 192 return "11102"; 193 case _11103: 194 return "11103"; 195 case _11104: 196 return "11104"; 197 case _21211: 198 return "21211"; 199 case _21212: 200 return "21212"; 201 case _27211: 202 return "27211"; 203 case _67211: 204 return "67211"; 205 case _99111: 206 return "99111"; 207 case _99333: 208 return "99333"; 209 case _99555: 210 return "99555"; 211 case NULL: 212 return null; 213 default: 214 return "?"; 215 } 216 } 217 218 public String getSystem() { 219 return "http://terminology.hl7.org/CodeSystem/ex-USCLS"; 220 } 221 222 public String getDefinition() { 223 switch (this) { 224 case _1101: 225 return "Exam, comp, primary"; 226 case _1102: 227 return "Exam, comp, mixed"; 228 case _1103: 229 return "Exam, comp, permanent"; 230 case _1201: 231 return "Exam, recall"; 232 case _1205: 233 return "Exam, emergency"; 234 case _2101: 235 return "Radiograph, series (12)"; 236 case _2102: 237 return "Radiograph, series (16)"; 238 case _2141: 239 return "Radiograph, bitewing"; 240 case _2601: 241 return "Radiograph, panoramic"; 242 case _11101: 243 return "Polishing, 1 unit"; 244 case _11102: 245 return "Polishing, 2 unit"; 246 case _11103: 247 return "Polishing, 3 unit"; 248 case _11104: 249 return "Polishing, 4 unit"; 250 case _21211: 251 return "Amalgam, 1 surface"; 252 case _21212: 253 return "Amalgam, 2 surface"; 254 case _27211: 255 return "Crown, PFM"; 256 case _67211: 257 return "Maryland Bridge"; 258 case _99111: 259 return "Lab, commercial"; 260 case _99333: 261 return "Lab, in office"; 262 case _99555: 263 return "Expense"; 264 case NULL: 265 return null; 266 default: 267 return "?"; 268 } 269 } 270 271 public String getDisplay() { 272 switch (this) { 273 case _1101: 274 return "Exam, comp, primary"; 275 case _1102: 276 return "Exam, comp, mixed"; 277 case _1103: 278 return "Exam, comp, permanent"; 279 case _1201: 280 return "Exam, recall"; 281 case _1205: 282 return "Exam, emergency"; 283 case _2101: 284 return "Radiograph, series (12)"; 285 case _2102: 286 return "Radiograph, series (16)"; 287 case _2141: 288 return "Radiograph, bitewing"; 289 case _2601: 290 return "Radiograph, panoramic"; 291 case _11101: 292 return "Polishing, 1 unit"; 293 case _11102: 294 return "Polishing, 2 unit"; 295 case _11103: 296 return "Polishing, 3 unit"; 297 case _11104: 298 return "Polishing, 4 unit"; 299 case _21211: 300 return "Amalgam, 1 surface"; 301 case _21212: 302 return "Amalgam, 2 surface"; 303 case _27211: 304 return "Crown, PFM"; 305 case _67211: 306 return "Maryland Bridge"; 307 case _99111: 308 return "Lab, commercial"; 309 case _99333: 310 return "Lab, in office"; 311 case _99555: 312 return "Expense"; 313 case NULL: 314 return null; 315 default: 316 return "?"; 317 } 318 } 319 320}