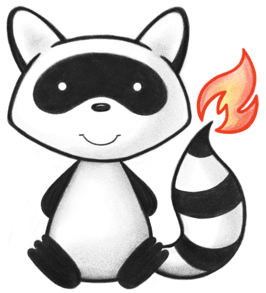
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum StructureDefinitionKind { 037 038 /** 039 * A primitive type that has a value and an extension. These can be used 040 * throughout complex datatype, Resource and extension definitions. Only the 041 * base specification can define primitive types. 042 */ 043 PRIMITIVETYPE, 044 /** 045 * A complex structure that defines a set of data elements that is suitable for 046 * use in 'resources'. The base specification defines a number of complex types, 047 * and other specifications can define additional types. These structures do not 048 * have a maintained identity. 049 */ 050 COMPLEXTYPE, 051 /** 052 * A 'resource' - a directed acyclic graph of elements that aggregrates other 053 * types into an identifiable entity. The base FHIR resources are defined by the 054 * FHIR specification itself but other 'resources' can be defined in additional 055 * specifications (though these will not be recognised as 'resources' by the 056 * FHIR specification (i.e. they do not get end-points etc, or act as the 057 * targets of references in FHIR defined resources - though other specificatiosn 058 * can treat them this way). 059 */ 060 RESOURCE, 061 /** 062 * A pattern or a template that is not intended to be a real resource or complex 063 * type. 064 */ 065 LOGICAL, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 071 public static StructureDefinitionKind fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("primitive-type".equals(codeString)) 075 return PRIMITIVETYPE; 076 if ("complex-type".equals(codeString)) 077 return COMPLEXTYPE; 078 if ("resource".equals(codeString)) 079 return RESOURCE; 080 if ("logical".equals(codeString)) 081 return LOGICAL; 082 throw new FHIRException("Unknown StructureDefinitionKind code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case PRIMITIVETYPE: 088 return "primitive-type"; 089 case COMPLEXTYPE: 090 return "complex-type"; 091 case RESOURCE: 092 return "resource"; 093 case LOGICAL: 094 return "logical"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 return "http://hl7.org/fhir/structure-definition-kind"; 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case PRIMITIVETYPE: 109 return "A primitive type that has a value and an extension. These can be used throughout complex datatype, Resource and extension definitions. Only the base specification can define primitive types."; 110 case COMPLEXTYPE: 111 return "A complex structure that defines a set of data elements that is suitable for use in 'resources'. The base specification defines a number of complex types, and other specifications can define additional types. These structures do not have a maintained identity."; 112 case RESOURCE: 113 return "A 'resource' - a directed acyclic graph of elements that aggregrates other types into an identifiable entity. The base FHIR resources are defined by the FHIR specification itself but other 'resources' can be defined in additional specifications (though these will not be recognised as 'resources' by the FHIR specification (i.e. they do not get end-points etc, or act as the targets of references in FHIR defined resources - though other specificatiosn can treat them this way)."; 114 case LOGICAL: 115 return "A pattern or a template that is not intended to be a real resource or complex type."; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDisplay() { 124 switch (this) { 125 case PRIMITIVETYPE: 126 return "Primitive Data Type"; 127 case COMPLEXTYPE: 128 return "Complex Data Type"; 129 case RESOURCE: 130 return "Resource"; 131 case LOGICAL: 132 return "Logical"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140}