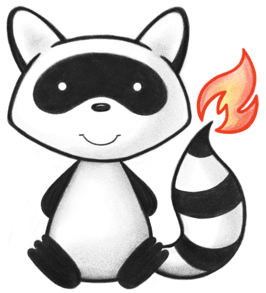
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum SupplydeliveryStatus { 037 038 /** 039 * Supply has been requested, but not delivered. 040 */ 041 INPROGRESS, 042 /** 043 * Supply has been delivered ("completed"). 044 */ 045 COMPLETED, 046 /** 047 * Delivery was not completed. 048 */ 049 ABANDONED, 050 /** 051 * This electronic record should never have existed, though it is possible that 052 * real-world decisions were based on it. (If real-world activity has occurred, 053 * the status should be "cancelled" rather than "entered-in-error".). 054 */ 055 ENTEREDINERROR, 056 /** 057 * added to help the parsers 058 */ 059 NULL; 060 061 public static SupplydeliveryStatus fromCode(String codeString) throws FHIRException { 062 if (codeString == null || "".equals(codeString)) 063 return null; 064 if ("in-progress".equals(codeString)) 065 return INPROGRESS; 066 if ("completed".equals(codeString)) 067 return COMPLETED; 068 if ("abandoned".equals(codeString)) 069 return ABANDONED; 070 if ("entered-in-error".equals(codeString)) 071 return ENTEREDINERROR; 072 throw new FHIRException("Unknown SupplydeliveryStatus code '" + codeString + "'"); 073 } 074 075 public String toCode() { 076 switch (this) { 077 case INPROGRESS: 078 return "in-progress"; 079 case COMPLETED: 080 return "completed"; 081 case ABANDONED: 082 return "abandoned"; 083 case ENTEREDINERROR: 084 return "entered-in-error"; 085 case NULL: 086 return null; 087 default: 088 return "?"; 089 } 090 } 091 092 public String getSystem() { 093 return "http://hl7.org/fhir/supplydelivery-status"; 094 } 095 096 public String getDefinition() { 097 switch (this) { 098 case INPROGRESS: 099 return "Supply has been requested, but not delivered."; 100 case COMPLETED: 101 return "Supply has been delivered (\"completed\")."; 102 case ABANDONED: 103 return "Delivery was not completed."; 104 case ENTEREDINERROR: 105 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getDisplay() { 114 switch (this) { 115 case INPROGRESS: 116 return "In Progress"; 117 case COMPLETED: 118 return "Delivered"; 119 case ABANDONED: 120 return "Abandoned"; 121 case ENTEREDINERROR: 122 return "Entered In Error"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130}