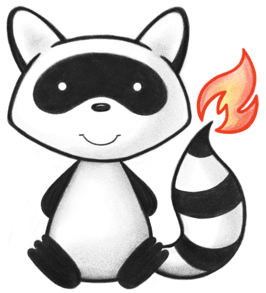
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3AcknowledgementDetailCode { 037 038 /** 039 * Refelects rejections because elements of the communication are not supported 040 * in the current context. 041 */ 042 _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE, 043 /** 044 * The interaction (or: this version of the interaction) is not supported. 045 */ 046 NS200, 047 /** 048 * The Processing ID is not supported. 049 */ 050 NS202, 051 /** 052 * The Version ID is not supported. 053 */ 054 NS203, 055 /** 056 * The processing mode is not supported. 057 */ 058 NS250, 059 /** 060 * The Device.id of the sender is unknown. 061 */ 062 NS260, 063 /** 064 * The receiver requires information in the attentionLine classes for routing 065 * purposes. 066 */ 067 NS261, 068 /** 069 * An internal software component (database, application, queue mechanism, etc.) 070 * has failed, leading to inability to process the message. 071 */ 072 INTERR, 073 /** 074 * Rejection: The message can't be stored by the receiver due to an unspecified 075 * internal application issue. The message was neither processed nor stored by 076 * the receiving application. 077 */ 078 NOSTORE, 079 /** 080 * Error: The destination of this message is known to the receiving application. 081 * Messages have been successfully routed to that destination in the past. The 082 * link to the destination application or an intermediate application is 083 * unavailable. 084 */ 085 RTEDEST, 086 /** 087 * The destination of this message is unknown to the receiving application. The 088 * receiving application in the message does not match the application which 089 * received the message. The message was neither routed, processed nor stored by 090 * the receiving application. 091 */ 092 RTUDEST, 093 /** 094 * Warning: The destination of this message is known to the receiving 095 * application. Messages have been successfully routed to that destination in 096 * the past. The link to the destination application or an intermediate 097 * application is (temporarily) unavailable. The receiving application will 098 * forward the message as soon as the destination can be reached again. 099 */ 100 RTWDEST, 101 /** 102 * Reflects errors in the syntax or structure of the communication. 103 */ 104 SYN, 105 /** 106 * The attribute contained data of the wrong data type, e.g. a numeric attribute 107 * contained "FOO". 108 */ 109 SYN102, 110 /** 111 * Description: Required association or attribute missing in message; or the 112 * sequence of the classes is different than required by the standard or one of 113 * the conformance profiles identified in the message. 114 */ 115 SYN105, 116 /** 117 * Required association missing in message; or the sequence of the classes is 118 * different than required by the standard or one of the conformance profiles 119 * identified in the message. 120 */ 121 SYN100, 122 /** 123 * A required attribute is missing in a class. 124 */ 125 SYN101, 126 /** 127 * Description: The number of repetitions of a group of association or 128 * attributes is less than the required minimum for the standard or of one of 129 * the conformance profiles or templates identified in the message. 130 */ 131 SYN114, 132 /** 133 * Description: A coded attribute or datatype property violates one of the 134 * terminology constraints specified in the standard or one of the conformance 135 * profiles or templates declared by the instance. 136 */ 137 SYN106, 138 /** 139 * An attribute value was compared against the corresponding code system, and no 140 * match was found. 141 */ 142 SYN103, 143 /** 144 * An attribute value referenced a code system that is not valid for an 145 * attribute constrained to CNE. 146 */ 147 SYN104, 148 /** 149 * Description: A coded attribute is referencing a code that has been deprecated 150 * by the owning code system. 151 */ 152 SYN107, 153 /** 154 * Description: The number of repetitions of a (group of) association(s) or 155 * attribute(s) exceeds the limits of the standard or of one of the conformance 156 * profiles or templates identified in the message. 157 */ 158 SYN108, 159 /** 160 * The number of repetitions of a (group of) association(s) exceeds the limits 161 * of the standard or of one of the conformance profiles identified in the 162 * message. 163 */ 164 SYN110, 165 /** 166 * The number of repetitions of an attribute exceeds the limits of the standard 167 * or of one of the conformance profiles identified in the message. 168 */ 169 SYN112, 170 /** 171 * Description: An attribute or association identified as mandatory in a 172 * specification or declared conformance profile or template has been specified 173 * with a null flavor. 174 */ 175 SYN109, 176 /** 177 * Description: The value of an attribute or property differs from the fixed 178 * value asserted in the standard or one of the conformance profiles or 179 * templates declared in the message. 180 */ 181 SYN111, 182 /** 183 * Description: A formal constraint asserted in the standard or one of the 184 * conformance profiles or templates declared in the message has been violated. 185 */ 186 SYN113, 187 /** 188 * added to help the parsers 189 */ 190 NULL; 191 192 public static V3AcknowledgementDetailCode fromCode(String codeString) throws FHIRException { 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("_AcknowledgementDetailNotSupportedCode".equals(codeString)) 196 return _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE; 197 if ("NS200".equals(codeString)) 198 return NS200; 199 if ("NS202".equals(codeString)) 200 return NS202; 201 if ("NS203".equals(codeString)) 202 return NS203; 203 if ("NS250".equals(codeString)) 204 return NS250; 205 if ("NS260".equals(codeString)) 206 return NS260; 207 if ("NS261".equals(codeString)) 208 return NS261; 209 if ("INTERR".equals(codeString)) 210 return INTERR; 211 if ("NOSTORE".equals(codeString)) 212 return NOSTORE; 213 if ("RTEDEST".equals(codeString)) 214 return RTEDEST; 215 if ("RTUDEST".equals(codeString)) 216 return RTUDEST; 217 if ("RTWDEST".equals(codeString)) 218 return RTWDEST; 219 if ("SYN".equals(codeString)) 220 return SYN; 221 if ("SYN102".equals(codeString)) 222 return SYN102; 223 if ("SYN105".equals(codeString)) 224 return SYN105; 225 if ("SYN100".equals(codeString)) 226 return SYN100; 227 if ("SYN101".equals(codeString)) 228 return SYN101; 229 if ("SYN114".equals(codeString)) 230 return SYN114; 231 if ("SYN106".equals(codeString)) 232 return SYN106; 233 if ("SYN103".equals(codeString)) 234 return SYN103; 235 if ("SYN104".equals(codeString)) 236 return SYN104; 237 if ("SYN107".equals(codeString)) 238 return SYN107; 239 if ("SYN108".equals(codeString)) 240 return SYN108; 241 if ("SYN110".equals(codeString)) 242 return SYN110; 243 if ("SYN112".equals(codeString)) 244 return SYN112; 245 if ("SYN109".equals(codeString)) 246 return SYN109; 247 if ("SYN111".equals(codeString)) 248 return SYN111; 249 if ("SYN113".equals(codeString)) 250 return SYN113; 251 throw new FHIRException("Unknown V3AcknowledgementDetailCode code '" + codeString + "'"); 252 } 253 254 public String toCode() { 255 switch (this) { 256 case _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE: 257 return "_AcknowledgementDetailNotSupportedCode"; 258 case NS200: 259 return "NS200"; 260 case NS202: 261 return "NS202"; 262 case NS203: 263 return "NS203"; 264 case NS250: 265 return "NS250"; 266 case NS260: 267 return "NS260"; 268 case NS261: 269 return "NS261"; 270 case INTERR: 271 return "INTERR"; 272 case NOSTORE: 273 return "NOSTORE"; 274 case RTEDEST: 275 return "RTEDEST"; 276 case RTUDEST: 277 return "RTUDEST"; 278 case RTWDEST: 279 return "RTWDEST"; 280 case SYN: 281 return "SYN"; 282 case SYN102: 283 return "SYN102"; 284 case SYN105: 285 return "SYN105"; 286 case SYN100: 287 return "SYN100"; 288 case SYN101: 289 return "SYN101"; 290 case SYN114: 291 return "SYN114"; 292 case SYN106: 293 return "SYN106"; 294 case SYN103: 295 return "SYN103"; 296 case SYN104: 297 return "SYN104"; 298 case SYN107: 299 return "SYN107"; 300 case SYN108: 301 return "SYN108"; 302 case SYN110: 303 return "SYN110"; 304 case SYN112: 305 return "SYN112"; 306 case SYN109: 307 return "SYN109"; 308 case SYN111: 309 return "SYN111"; 310 case SYN113: 311 return "SYN113"; 312 case NULL: 313 return null; 314 default: 315 return "?"; 316 } 317 } 318 319 public String getSystem() { 320 return "http://terminology.hl7.org/CodeSystem/v3-AcknowledgementDetailCode"; 321 } 322 323 public String getDefinition() { 324 switch (this) { 325 case _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE: 326 return "Refelects rejections because elements of the communication are not supported in the current context."; 327 case NS200: 328 return "The interaction (or: this version of the interaction) is not supported."; 329 case NS202: 330 return "The Processing ID is not supported."; 331 case NS203: 332 return "The Version ID is not supported."; 333 case NS250: 334 return "The processing mode is not supported."; 335 case NS260: 336 return "The Device.id of the sender is unknown."; 337 case NS261: 338 return "The receiver requires information in the attentionLine classes for routing purposes."; 339 case INTERR: 340 return "An internal software component (database, application, queue mechanism, etc.) has failed, leading to inability to process the message."; 341 case NOSTORE: 342 return "Rejection: The message can't be stored by the receiver due to an unspecified internal application issue. The message was neither processed nor stored by the receiving application."; 343 case RTEDEST: 344 return "Error: The destination of this message is known to the receiving application. Messages have been successfully routed to that destination in the past. The link to the destination application or an intermediate application is unavailable."; 345 case RTUDEST: 346 return "The destination of this message is unknown to the receiving application. The receiving application in the message does not match the application which received the message. The message was neither routed, processed nor stored by the receiving application."; 347 case RTWDEST: 348 return "Warning: The destination of this message is known to the receiving application. Messages have been successfully routed to that destination in the past. The link to the destination application or an intermediate application is (temporarily) unavailable. The receiving application will forward the message as soon as the destination can be reached again."; 349 case SYN: 350 return "Reflects errors in the syntax or structure of the communication."; 351 case SYN102: 352 return "The attribute contained data of the wrong data type, e.g. a numeric attribute contained \"FOO\"."; 353 case SYN105: 354 return "Description: Required association or attribute missing in message; or the sequence of the classes is different than required by the standard or one of the conformance profiles identified in the message."; 355 case SYN100: 356 return "Required association missing in message; or the sequence of the classes is different than required by the standard or one of the conformance profiles identified in the message."; 357 case SYN101: 358 return "A required attribute is missing in a class."; 359 case SYN114: 360 return "Description: The number of repetitions of a group of association or attributes is less than the required minimum for the standard or of one of the conformance profiles or templates identified in the message."; 361 case SYN106: 362 return "Description: A coded attribute or datatype property violates one of the terminology constraints specified in the standard or one of the conformance profiles or templates declared by the instance."; 363 case SYN103: 364 return "An attribute value was compared against the corresponding code system, and no match was found."; 365 case SYN104: 366 return "An attribute value referenced a code system that is not valid for an attribute constrained to CNE."; 367 case SYN107: 368 return "Description: A coded attribute is referencing a code that has been deprecated by the owning code system."; 369 case SYN108: 370 return "Description: The number of repetitions of a (group of) association(s) or attribute(s) exceeds the limits of the standard or of one of the conformance profiles or templates identified in the message."; 371 case SYN110: 372 return "The number of repetitions of a (group of) association(s) exceeds the limits of the standard or of one of the conformance profiles identified in the message."; 373 case SYN112: 374 return "The number of repetitions of an attribute exceeds the limits of the standard or of one of the conformance profiles identified in the message."; 375 case SYN109: 376 return "Description: An attribute or association identified as mandatory in a specification or declared conformance profile or template has been specified with a null flavor."; 377 case SYN111: 378 return "Description: The value of an attribute or property differs from the fixed value asserted in the standard or one of the conformance profiles or templates declared in the message."; 379 case SYN113: 380 return "Description: A formal constraint asserted in the standard or one of the conformance profiles or templates declared in the message has been violated."; 381 case NULL: 382 return null; 383 default: 384 return "?"; 385 } 386 } 387 388 public String getDisplay() { 389 switch (this) { 390 case _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE: 391 return "AcknowledgementDetailNotSupportedCode"; 392 case NS200: 393 return "Unsupported interaction"; 394 case NS202: 395 return "Unsupported processing id"; 396 case NS203: 397 return "Unsupported version id"; 398 case NS250: 399 return "Unsupported processing Mode"; 400 case NS260: 401 return "Unknown sender"; 402 case NS261: 403 return "Unrecognized attentionline"; 404 case INTERR: 405 return "Internal system error"; 406 case NOSTORE: 407 return "No storage space for message."; 408 case RTEDEST: 409 return "Message routing error, destination unreachable."; 410 case RTUDEST: 411 return "Error: Message routing error, unknown destination."; 412 case RTWDEST: 413 return "Message routing warning, destination unreachable."; 414 case SYN: 415 return "Syntax error"; 416 case SYN102: 417 return "Data type error"; 418 case SYN105: 419 return "Required element missing"; 420 case SYN100: 421 return "Required association missing"; 422 case SYN101: 423 return "Required attribute missing"; 424 case SYN114: 425 return "Insufficient repetitions"; 426 case SYN106: 427 return "Terminology error"; 428 case SYN103: 429 return "Value not found in code system"; 430 case SYN104: 431 return "Invalid code system in CNE"; 432 case SYN107: 433 return "Deprecated code"; 434 case SYN108: 435 return "Number of repetitions exceeds limit"; 436 case SYN110: 437 return "Number of association repetitions exceeds limit"; 438 case SYN112: 439 return "Number of attribute repetitions exceeds limit"; 440 case SYN109: 441 return "Mandatory element with null value"; 442 case SYN111: 443 return "Value does not match fixed value"; 444 case SYN113: 445 return "Formal constraint violation"; 446 case NULL: 447 return null; 448 default: 449 return "?"; 450 } 451 } 452 453}